Red Hat Summit NanoService Demo for LPC1768 App Board using OMA Lightweight Objects
Dependencies: Beep C12832_lcd EthernetInterface LM75B MMA7660 mbed-rtos mbed nsdl_lib
Fork of LWM2M_NanoService_Ethernet by
rgb.cpp
00001 // Light resource implementation 00002 00003 #include "mbed.h" 00004 #include "rgb.h" 00005 00006 #define RGB_COLOUR_RES_ID "308/0/0" 00007 #define RGB_SETPT_RES_ID "308/0/5900" 00008 #define RGB_UNITS_RES_ID "308/0/5701" 00009 00010 extern Serial pc; 00011 00012 static int rgb[] = {0, 0, 0}; 00013 00014 class RGB { 00015 public: 00016 RGB(); 00017 void show(float r, float g, float b); 00018 00019 private: 00020 PwmOut rout; 00021 PwmOut gout; 00022 PwmOut bout; 00023 }; 00024 RGB::RGB() : rout(p23), gout(p24), bout(p25) { 00025 pc.printf("RGB.ctor()\r\n"); 00026 rout.period(0.001); 00027 gout.period(0.001); 00028 bout.period(0.001); 00029 } 00030 00031 /* 00032 The RGB LED is common anode, so that "0" is on, and "1" is off. For PWM, the closer to 0.0 the brighter, the closer to 1.0 the dimmer. 00033 This method uses (1.0 - rgb value) to invert. 00034 */ 00035 void RGB::show(float r, float g, float b) { 00036 rout = 1 - r; 00037 gout = 1 - g; 00038 bout = 1 - b; 00039 } 00040 00041 /* 00042 Convert the r|g|b string into the integer parts, each in the range [0,255] 00043 */ 00044 static void decode_rgb(char *rgbstr) 00045 { 00046 char tmp[4]; 00047 memset(tmp, 0, sizeof(tmp)); 00048 char *next; 00049 char *token = rgbstr; 00050 int index = 0; 00051 while((next = strchr(token, '|')) != NULL) { 00052 int len = next - token; 00053 strncpy(tmp, token, len); 00054 rgb[index ++] = atoi(tmp); 00055 token = next + 1; 00056 } 00057 rgb[index] = atoi(token); 00058 pc.printf("decode_rgb(%s) = %d,%d,%d\r\n", rgbstr, rgb[0], rgb[1], rgb[2]); 00059 } 00060 00061 static float RGB_SCALE = 1.0 / 255.0; 00062 /* 00063 The RGB LED is common anode, so that "0" is on, and "1" is off. For PWM, the closer to 0.0 the brighter, the closer to 1.0 the dimmer. 00064 This method uses (1.0 - rgb value) to invert. 00065 */ 00066 static void setRGB() 00067 { 00068 static RGB rgbLed; 00069 pc.printf("Changing to RGB(%d,%d,%d)\r\n", rgb[0], rgb[1], rgb[2]); 00070 float r = rgb[0] * RGB_SCALE; 00071 float g = rgb[1] * RGB_SCALE; 00072 float b = rgb[2] * RGB_SCALE; 00073 rgbLed.show(r, g, b); 00074 } 00075 00076 /* Only GET and PUT method allowed */ 00077 static uint8_t rgb_resource_cb(sn_coap_hdr_s *received_coap_ptr, sn_nsdl_addr_s *address, sn_proto_info_s * proto) 00078 { 00079 sn_coap_hdr_s *coap_res_ptr = 0; 00080 char rgbstr[16]; 00081 00082 pc.printf("rgb callback\r\n"); 00083 00084 if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_GET) 00085 { 00086 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CONTENT); 00087 00088 sprintf(rgbstr, "%d|%d|%d", rgb[0], rgb[1], rgb[2]); 00089 00090 coap_res_ptr->payload_len = strlen(rgbstr); 00091 coap_res_ptr->payload_ptr = (uint8_t*)rgbstr; 00092 sn_nsdl_send_coap_message(address, coap_res_ptr); 00093 } 00094 else if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_PUT) 00095 { 00096 memcpy(rgbstr, (char *)received_coap_ptr->payload_ptr, received_coap_ptr->payload_len); 00097 rgbstr[received_coap_ptr->payload_len] = '\0'; 00098 decode_rgb(rgbstr); 00099 setRGB(); 00100 00101 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CHANGED); 00102 sn_nsdl_send_coap_message(address, coap_res_ptr); 00103 } 00104 00105 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00106 return 0; 00107 } 00108 00109 int create_rgb_resource(sn_nsdl_resource_info_s *resource_ptr) 00110 { 00111 nsdl_create_dynamic_resource(resource_ptr, sizeof(RGB_SETPT_RES_ID)-1, (uint8_t*)RGB_SETPT_RES_ID, 0, 0, 0, &rgb_resource_cb, (SN_GRS_GET_ALLOWED | SN_GRS_PUT_ALLOWED)); 00112 nsdl_create_static_resource(resource_ptr, sizeof(RGB_COLOUR_RES_ID)-1, (uint8_t*) RGB_COLOUR_RES_ID, 0, 0, (uint8_t*) "RBG LED Colour", sizeof("RBG LED Colour")-1); 00113 nsdl_create_static_resource(resource_ptr, sizeof(RGB_UNITS_RES_ID)-1, (uint8_t*) RGB_UNITS_RES_ID, 0, 0, (uint8_t*) "(R|G|B)0-255", sizeof("(R|G|B)0-255")-1); 00114 setRGB(); 00115 return 0; 00116 } 00117 void zero_rgb() 00118 { 00119 memset(rgb, 0, sizeof(rgb)); 00120 setRGB(); 00121 }
Generated on Tue Jul 12 2022 21:21:48 by
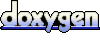