Red Hat Summit NanoService Demo for LPC1768 App Board using OMA Lightweight Objects
Dependencies: Beep C12832_lcd EthernetInterface LM75B MMA7660 mbed-rtos mbed nsdl_lib
Fork of LWM2M_NanoService_Ethernet by
light.cpp
00001 // Light resource implementation 00002 00003 #include "mbed.h" 00004 #include "nsdl_support.h" 00005 #include "light.h" 00006 00007 //#define LIGHT_RES_ID "311/0/5851" 00008 #define LIGHT_FLASH_ID "311/0/5850" 00009 00010 extern Serial pc; 00011 static PwmOut led2(LED2); 00012 static PwmOut led3(LED3); 00013 00014 static DigitalOut out1(p22); 00015 static Ticker flash; 00016 static Timeout reset; 00017 00018 #include "Beep.h" 00019 static Beep buzzer(p26); 00020 Ticker beep_tick; 00021 static void beepHanlder(void); 00022 00023 static void beepHandler(void) 00024 { 00025 buzzer.beep(1000, 0.3f); 00026 } 00027 00028 00029 static void flashHandler(void) 00030 { 00031 out1 = 0; 00032 wait(0.1f); 00033 out1 = 1; 00034 } 00035 00036 ///* Only GET and PUT method allowed */ 00037 //static uint8_t light_resource_cb(sn_coap_hdr_s *received_coap_ptr, sn_nsdl_addr_s *address, sn_proto_info_s * proto) 00038 //{ 00039 // sn_coap_hdr_s *coap_res_ptr = 0; 00040 // static float led_dimm = 0; 00041 // int led_state = 0; 00042 // char led_dimm_temp[4]; 00043 // 00044 // pc.printf("light dimmer callback\r\n"); 00045 // 00046 // if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_GET) { 00047 // coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CONTENT); 00048 // 00049 // led_state = led_dimm * 100; 00050 // sprintf(led_dimm_temp, "%d", led_state); 00051 // 00052 // coap_res_ptr->payload_len = strlen(led_dimm_temp); 00053 // coap_res_ptr->payload_ptr = (uint8_t*)led_dimm_temp; 00054 // sn_nsdl_send_coap_message(address, coap_res_ptr); 00055 // } else if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_PUT) { 00056 // memcpy(led_dimm_temp, (char *)received_coap_ptr->payload_ptr, received_coap_ptr->payload_len); 00057 // 00058 // led_dimm_temp[received_coap_ptr->payload_len] = '\0'; 00059 // 00060 // led_dimm = atof(led_dimm_temp); 00061 // led_dimm = led_dimm/100; 00062 // 00063 // led2.write(led_dimm); 00064 // led3.write(led_dimm); 00065 // 00066 // coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CHANGED); 00067 // sn_nsdl_send_coap_message(address, coap_res_ptr); 00068 // } 00069 // 00070 // sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00071 // return 0; 00072 //} 00073 00074 00075 /* Only GET and PUT method allowed */ 00076 static uint8_t light_flash_resource_cb(sn_coap_hdr_s *received_coap_ptr, sn_nsdl_addr_s *address, sn_proto_info_s * proto) 00077 { 00078 sn_coap_hdr_s *coap_res_ptr = 0; 00079 static uint8_t flash_state = '0'; 00080 00081 pc.printf("flash callback\r\n"); 00082 00083 if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_GET) 00084 { 00085 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CONTENT); 00086 00087 coap_res_ptr->payload_len = 1; 00088 coap_res_ptr->payload_ptr = &flash_state; 00089 sn_nsdl_send_coap_message(address, coap_res_ptr); 00090 } 00091 else if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_PUT) 00092 { 00093 if(received_coap_ptr->payload_len) 00094 { 00095 if(*(received_coap_ptr->payload_ptr) == '1') 00096 { 00097 flash.attach(&flashHandler, 10.0f); 00098 beep_tick.attach(&beepHandler, 0.6f); 00099 flash_state = '1'; 00100 out1 = 1; 00101 } 00102 else if(*(received_coap_ptr->payload_ptr) == '0') 00103 { 00104 out1 = 0; 00105 buzzer.nobeep(); 00106 flash.detach(); 00107 reset.detach(); 00108 beep_tick.detach(); 00109 flash_state = '0'; 00110 } 00111 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CHANGED); 00112 sn_nsdl_send_coap_message(address, coap_res_ptr); 00113 } 00114 } 00115 00116 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00117 00118 return 0; 00119 } 00120 00121 int create_light_resource(sn_nsdl_resource_info_s *resource_ptr) 00122 { 00123 //nsdl_create_dynamic_resource(resource_ptr, sizeof(LIGHT_RES_ID)-1, (uint8_t*)LIGHT_RES_ID, 0, 0, 0, &light_resource_cb, (SN_GRS_GET_ALLOWED | SN_GRS_PUT_ALLOWED)); 00124 nsdl_create_dynamic_resource(resource_ptr, sizeof(LIGHT_FLASH_ID)-1, (uint8_t*)LIGHT_FLASH_ID, 0, 0, 0, &light_flash_resource_cb, (SN_GRS_GET_ALLOWED | SN_GRS_PUT_ALLOWED)); 00125 return 0; 00126 }
Generated on Tue Jul 12 2022 21:21:48 by
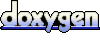