Hard coded pins for nRF51822 in this version
Fork of Grove_3-Axis_Digital_Accelerometer_MMA7660FC_Library by
MMA7660FC.cpp
00001 /* 00002 * MMA7760.h 00003 * Library for accelerometer_MMA7760 00004 * 00005 * Copyright (c) 2013 seeed technology inc. 00006 * Author : FrankieChu 00007 * Create Time : Jan 2013 00008 * Change Log : 00009 * 00010 * The MIT License (MIT) 00011 * 00012 * Permission is hereby granted, free of charge, to any person obtaining a copy 00013 * of this software and associated documentation files (the "Software"), to deal 00014 * in the Software without restriction, including without limitation the rights 00015 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00016 * copies of the Software, and to permit persons to whom the Software is 00017 * furnished to do so, subject to the following conditions: 00018 * 00019 * The above copyright notice and this permission notice shall be included in 00020 * all copies or substantial portions of the Software. 00021 * 00022 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00023 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00024 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00025 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00026 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00027 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00028 * THE SOFTWARE. 00029 */ 00030 00031 #include "MMA7660.h" 00032 00033 I2C i2c(P0_22, P0_20); 00034 00035 /*Function: Write a byte to the register of the MMA7660*/ 00036 void MMA7660::write(uint8_t _register, uint8_t _data) 00037 { 00038 char data[2] = {_register,_data}; 00039 int check; 00040 //Wire.begin(); 00041 //Wire.beginTransmission(MMA7660_ADDR); 00042 //Wire.write(_register); 00043 //Wire.write(_data); 00044 //Wire.endTransmission(); 00045 check = i2c.write(MMA7660_ADDR<<1,data,2); 00046 if(check != 0) 00047 printf("I2C Write Failure\n\r"); 00048 } 00049 /*Function: Read a byte from the regitster of the MMA7660*/ 00050 uint8_t MMA7660::read(uint8_t _register) 00051 { 00052 char data_read[2] = {_register,0}; 00053 int check; 00054 check = i2c.write(MMA7660_ADDR<<1,data_read,1); // write _register to set up read 00055 check = i2c.read(MMA7660_ADDR<<1,data_read,2); // execute read 00056 if(check != 0) 00057 printf("I2C Read failure\n\r"); 00058 printf("read back data 0x%x, 0x%x from register 0x%x",data_read[0],data_read[1],_register); 00059 //uint8_t data_read; 00060 //Wire.begin(); 00061 //Wire.beginTransmission(MMA7660_ADDR); 00062 //Wire.write(_register); 00063 //Wire.endTransmission(); 00064 //Wire.beginTransmission(MMA7660_ADDR); 00065 //Wire.requestFrom(MMA7660_ADDR,1); 00066 //while(Wire.available()) 00067 //{ 00068 // data_read = Wire.read(); 00069 //} 00070 //Wire.endTransmission(); 00071 return data_read[1]; 00072 } 00073 00074 void MMA7660::init() 00075 { 00076 i2c.frequency(400000); 00077 setMode(MMA7660_STAND_BY); 00078 setSampleRate(AUTO_SLEEP_32); 00079 setMode(MMA7660_ACTIVE); 00080 } 00081 void MMA7660::setMode(uint8_t mode) 00082 { 00083 write(MMA7660_MODE,mode); 00084 } 00085 void MMA7660::setSampleRate(uint8_t rate) 00086 { 00087 write(MMA7660_SR,rate); 00088 } 00089 /*Function: Get the contents of the registers in the MMA7660*/ 00090 /* so as to calculate the acceleration. */ 00091 void MMA7660::getXYZ(int8_t *x,int8_t *y,int8_t *z) 00092 { 00093 char val[3]; 00094 int count = 0; 00095 bool done = false; 00096 val[0] = val[1] = val[2] = 64; 00097 // while(Wire.available() > 0) 00098 // Wire.read(); 00099 // Wire.requestFrom(MMA7660_ADDR,3); 00100 // while(Wire.available()) 00101 // { 00102 // if(count < 3) 00103 // { 00104 // while ( val[count] > 63 ) // reload the damn thing it is bad 00105 // { 00106 // val[count] = Wire.read(); 00107 // } 00108 // } 00109 // count++; 00110 // } 00111 for(count = 0; count < 3 && done == false; count ++){ 00112 i2c.read(MMA7660_ADDR<<1, val,3); 00113 if(val[0] < 63 && val[1]<63 && val[2]<63) 00114 done = true; 00115 } 00116 00117 *x = ((char)(val[0]<<2))/4; 00118 *y = ((char)(val[1]<<2))/4; 00119 *z = ((char)(val[2]<<2))/4; 00120 } 00121 00122 void MMA7660::getAcceleration(float *ax,float *ay,float *az) 00123 { 00124 int8_t x,y,z; 00125 getXYZ(&x,&y,&z); 00126 *ax = x/21.00; 00127 *ay = y/21.00; 00128 *az = z/21.00; 00129 }
Generated on Thu Jul 14 2022 02:06:05 by
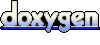