
TEST_LoRaSTboard
Dependencies: BufferedSerial SX1276GenericLib mbed
main.cpp
00001 /* 00002 * Copyright (c) 2017 Helmut Tschemernjak 00003 * 30826 Garbsen (Hannover) Germany 00004 * Licensed under the Apache License, Version 2.0); 00005 */ 00006 #include "main.h" 00007 00008 DigitalOut myled(LED1); 00009 BufferedSerial *ser; 00010 00011 int main() { 00012 SystemClock_Config(); 00013 ser = new BufferedSerial(USBTX, USBRX); 00014 ser->baud(115200*2); 00015 ser->format(8); 00016 ser->printf("Hello World\n\r"); 00017 myled = 1; 00018 00019 SX1276PingPong(); 00020 } 00021 00022 00023 00024 00025 void SystemClock_Config(void) 00026 { 00027 #ifdef B_L072Z_LRWAN1_LORA 00028 /* 00029 * The L072Z_LRWAN1_LORA clock setup is somewhat differnt from the Nucleo board. 00030 * It has no LSE. 00031 */ 00032 RCC_ClkInitTypeDef RCC_ClkInitStruct = {0}; 00033 RCC_OscInitTypeDef RCC_OscInitStruct = {0}; 00034 00035 /* Enable HSE Oscillator and Activate PLL with HSE as source */ 00036 RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSI; 00037 RCC_OscInitStruct.HSEState = RCC_HSE_OFF; 00038 RCC_OscInitStruct.HSIState = RCC_HSI_ON; 00039 RCC_OscInitStruct.HSICalibrationValue = RCC_HSICALIBRATION_DEFAULT; 00040 RCC_OscInitStruct.PLL.PLLState = RCC_PLL_ON; 00041 RCC_OscInitStruct.PLL.PLLSource = RCC_PLLSOURCE_HSI; 00042 RCC_OscInitStruct.PLL.PLLMUL = RCC_PLLMUL_6; 00043 RCC_OscInitStruct.PLL.PLLDIV = RCC_PLLDIV_3; 00044 00045 if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK) { 00046 // Error_Handler(); 00047 } 00048 00049 /* Set Voltage scale1 as MCU will run at 32MHz */ 00050 __HAL_RCC_PWR_CLK_ENABLE(); 00051 __HAL_PWR_VOLTAGESCALING_CONFIG(PWR_REGULATOR_VOLTAGE_SCALE1); 00052 00053 /* Poll VOSF bit of in PWR_CSR. Wait until it is reset to 0 */ 00054 while (__HAL_PWR_GET_FLAG(PWR_FLAG_VOS) != RESET) {}; 00055 00056 /* Select PLL as system clock source and configure the HCLK, PCLK1 and PCLK2 00057 clocks dividers */ 00058 RCC_ClkInitStruct.ClockType = (RCC_CLOCKTYPE_SYSCLK | RCC_CLOCKTYPE_HCLK | RCC_CLOCKTYPE_PCLK1 | RCC_CLOCKTYPE_PCLK2); 00059 RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_PLLCLK; 00060 RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1; 00061 RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV1; 00062 RCC_ClkInitStruct.APB2CLKDivider = RCC_HCLK_DIV1; 00063 if (HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_1) != HAL_OK) { 00064 // Error_Handler(); 00065 } 00066 #endif 00067 } 00068 00069 void dump(const char *title, const void *data, int len, bool dwords) 00070 { 00071 dprintf("dump(\"%s\", 0x%x, %d bytes)", title, data, len); 00072 00073 int i, j, cnt; 00074 unsigned char *u; 00075 const int width = 16; 00076 const int seppos = 7; 00077 00078 cnt = 0; 00079 u = (unsigned char *)data; 00080 while (len > 0) { 00081 ser->printf("%08x: ", (unsigned int)data + cnt); 00082 if (dwords) { 00083 unsigned int *ip = ( unsigned int *)u; 00084 ser->printf(" 0x%08x\r\n", *ip); 00085 u+= 4; 00086 len -= 4; 00087 cnt += 4; 00088 continue; 00089 } 00090 cnt += width; 00091 j = len < width ? len : width; 00092 for (i = 0; i < j; i++) { 00093 ser->printf("%2.2x ", *(u + i)); 00094 if (i == seppos) 00095 ser->putc(' '); 00096 } 00097 ser->putc(' '); 00098 if (j < width) { 00099 i = width - j; 00100 if (i > seppos + 1) 00101 ser->putc(' '); 00102 while (i--) { 00103 printf("%s", " "); 00104 } 00105 } 00106 for (i = 0; i < j; i++) { 00107 int c = *(u + i); 00108 if (c >= ' ' && c <= '~') 00109 ser->putc(c); 00110 else 00111 ser->putc('.'); 00112 if (i == seppos) 00113 ser->putc(' '); 00114 } 00115 len -= width; 00116 u += width; 00117 ser->printf("\r\n"); 00118 } 00119 ser->printf("--\r\n"); 00120 }
Generated on Fri Jul 15 2022 07:36:47 by
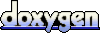