
Both displays functioning independently, we will use this program to bring them both into the same code.
Dependencies: SPI_TFT_ILI9341 TFT_fonts TextLCD mbed
main.cpp
00001 00002 #include "mbed.h" 00003 #include "TextLCD.h" 00004 #include "stdio.h" 00005 #include "SPI_TFT_ILI9341.h" 00006 #include "string" 00007 #include "Arial28x28.h" 00008 #include "Arial24x23.h" 00009 #include "Arial12x12.h" 00010 00011 00012 // Host PC Communication channels 00013 Serial pc(USBTX, USBRX); // tx, rx 00014 00015 // LCD instantiation 00016 TextLCD lcd(PTC7, PTC0, PTD4, PTA12, PTC5, PTC6); // 4bit bus: rs, e, d4-d7 00017 00018 // the display has a backlight switch on board 00019 DigitalOut LCD_LED(PTA13); 00020 DigitalOut pwr(PTD7); 00021 00022 // the TFT is connected to SPI pin 5-7 00023 //SPI_TFT_ILI9341 TFT(p5, p6, p7, p8, p9, p10,"TFT"); // mosi, miso, sclk, cs, reset, dc for lpc1768 00024 SPI_TFT_ILI9341 TFT(PTD2, PTD3, PTD1, PTD5, PTD0, PTA13,"TFT"); // mosi, miso, sclk, cs, reset, dc for frdmkl25z 00025 00026 //void updateDisplay(int time, int date, int temp) { 00027 00028 int main() { // Main program 00029 00030 int time = 1234; 00031 int date = 220517; 00032 int temp = 27; 00033 int weather = 2; // 0 - Rain, 1 - Cloud, 2 - Sun 00034 00035 // Adjust format of time to reflect hours and minutes 00036 int hours = time/100; 00037 int minutes = time - hours*100; 00038 00039 // Adjust format of date to reflect days and minutes 00040 int day = date/10000; 00041 int month = (date - day*10000)/100; 00042 int year = date - month*100 - day*10000; 00043 00044 // Print correctly spaced values on the display 00045 00046 lcd.printf("Time %d:%d", hours, minutes); 00047 00048 // Locate cursor to start of second line 00049 00050 lcd.setAddress(0, 1); 00051 00052 lcd.printf("Date %d/%d/%d", day, month, year); 00053 00054 // Control for the touch screen display 00055 00056 pwr=1; // Power On 00057 00058 LCD_LED = 1; // Backlight on 00059 00060 TFT.claim(stdout); // Send stdout to the TFT display 00061 TFT.set_orientation(1); // Orients screen to wipe in the horizontal direction 00062 TFT.background(Black); // Sets background to black 00063 TFT.foreground(White); // Sets text to white 00064 TFT.cls(); // Clear the screen 00065 00066 TFT.set_orientation(0); // Ensures orientation vertical 0 degrees 00067 TFT.background(Black); // Sets background to black 00068 TFT.cls(); // Clear the screen 00069 00070 TFT.set_font((unsigned char*) Arial24x23); // Select header font 00071 TFT.locate(10, 180); // x,y - locates cursor 00072 TFT.printf("Oxford"); // Print Oxford 00073 00074 TFT.set_font((unsigned char*) Arial28x28); // Select header font 00075 TFT.locate(10,220); // x,y - locates cursor 00076 TFT.printf("%dC", temp); // Print temperature data 00077 00078 // Print weather dependant images on the touch screen 00079 00080 switch(weather) { // Decides which weather state to print based on the web update 00081 00082 case 0: // Rain 00083 00084 TFT.fillcircle(115,78,20,DarkGrey); // An image of the rain, stored on the board and loaded if weather = 0 00085 TFT.fillcircle(130,60,15,DarkGrey); 00086 TFT.fillcircle(160,55,30,DarkGrey); 00087 TFT.fillcircle(190,60,15,DarkGrey); 00088 TFT.fillcircle(205,75,20,DarkGrey); 00089 TFT.fillcircle(158,75,22,DarkGrey); 00090 TFT.fillcircle(130,75,20,DarkGrey); 00091 TFT.fillcircle(185,80,15,DarkGrey); 00092 00093 TFT.line(110,100,110,130,Blue); 00094 TFT.line(134,102,134,136,Blue); 00095 TFT.line(158,101,158,139,Blue); 00096 TFT.line(182,102,182,136,Blue); 00097 TFT.line(206,100,206,130,Blue); 00098 00099 break; 00100 00101 case 1: // Cloud 00102 00103 TFT.fillcircle(115,78,20,White); // An image of a cloud, stored on the board and loaded if weather = 1 00104 TFT.fillcircle(130,60,15,White); 00105 TFT.fillcircle(160,55,30,White); 00106 TFT.fillcircle(190,60,15,White); 00107 TFT.fillcircle(205,75,20,White); 00108 TFT.fillcircle(158,75,22,White); 00109 TFT.fillcircle(130,75,20,White); 00110 TFT.fillcircle(185,80,15,White); 00111 00112 break; 00113 00114 case 2: // Sun 00115 00116 TFT.fillcircle(170,80,40,Yellow); // An image of the sun, stored on the board and loaded if weather = 2 00117 00118 break; 00119 00120 } 00121 00122 }
Generated on Fri Jul 15 2022 21:22:37 by
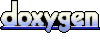