
Added a GPIO to power on/off for external I2C sensor(s) (with LEDs)
Dependencies: UniGraphic mbed vt100
iafLib.h
00001 /** 00002 * Copyright 2015 Afero, Inc. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /** 00018 * afLib public interface 00019 * 00020 * This file defines everything your application should need for commuinicating with the Afero ASR-1 radio module. 00021 * Is there is anything missing from this file, please post a request on the developer forum and we will see what 00022 * we can do. 00023 */ 00024 #ifndef AFLIB_IAFLIB_H 00025 #define AFLIB_IAFLIB_H 00026 00027 #include "mbed.h" 00028 #include <string> 00029 using namespace std; 00030 #include "afErrors.h" 00031 #include "afSPI.h" 00032 00033 #if defined (TARGET_TEENSY3_1) 00034 #include "USBSerial.h" 00035 #endif 00036 00037 #define afMINIMUM_TIME_BETWEEN_REQUESTS 1000 00038 00039 #define MAX_ATTRIBUTE_SIZE 255 00040 00041 typedef void (*isr)(); 00042 typedef void (*onAttributeSet)(const uint8_t requestId, const uint16_t attributeId, const uint16_t valueLen, const uint8_t *value); 00043 typedef void (*onAttributeSetComplete)(const uint8_t requestId, const uint16_t attributeId, const uint16_t valueLen, const uint8_t *value); 00044 00045 class iafLib { 00046 public: 00047 /** 00048 * create 00049 * 00050 * Create an instance of the afLib object. The afLib is a singleton. Calling this method multiple 00051 * times will return the same instance. 00052 * 00053 * @param mcuInterrupt The Arduino interrupt to be used (returned from digitalPinToInterrupt) 00054 * @param isrWrapper This is the isr method that must be defined in your sketch 00055 * @param attrSet Callback for notification of attribute set requests 00056 * @param attrSetComplete Callback for notification of attribute set request completions 00057 * @return iafLib * Instance of iafLib 00058 */ 00059 static iafLib * create(PinName mcuInterrupt, isr isrWrapper, 00060 onAttributeSet attrSet, onAttributeSetComplete attrSetComplete, afSPI *theSPI); 00061 00062 //wsugi 20161128 00063 static void destroy(); 00064 00065 /** 00066 * loop 00067 * 00068 * Called by the loop() method in your sketch to give afLib some CPU time 00069 */ 00070 virtual void loop(void) = 0; 00071 00072 /** 00073 * getAttribute 00074 * 00075 * Request the value of an attribute be returned from the ASR-1. 00076 * Value will be returned in the attrSetComplete callback. 00077 */ 00078 virtual int getAttribute(const uint16_t attrId) = 0; 00079 00080 /** 00081 * setAttribute 00082 * 00083 * Request setting an attribute. 00084 * For MCU attributes, the attribute value will be updated. 00085 * For IO attributes, the attribute value will be updated, and then onAttrSetComplete will be called. 00086 */ 00087 virtual int setAttributeBool(const uint16_t attrId, const bool value) = 0; 00088 00089 virtual int setAttribute8(const uint16_t attrId, const int8_t value) = 0; 00090 00091 virtual int setAttribute16(const uint16_t attrId, const int16_t value) = 0; 00092 00093 virtual int setAttribute32(const uint16_t attrId, const int32_t value) = 0; 00094 00095 virtual int setAttribute64(const uint16_t attrId, const int64_t value) = 0; 00096 00097 virtual int setAttribute(const uint16_t attrId, const string &value) = 0; 00098 00099 virtual int setAttribute(const uint16_t attrId, const uint16_t valueLen, const char *value) = 0; 00100 00101 virtual int setAttribute(const uint16_t attrId, const uint16_t valueLen, const uint8_t *value) = 0; 00102 00103 /** 00104 * setAttributeComplete 00105 * 00106 * Call this in response to an onAttrSet. This lets the ASR-1 know that the set request has been handled. 00107 */ 00108 virtual int setAttributeComplete(uint8_t requestId, const uint16_t attrId, const uint16_t valueLen, const uint8_t *value) = 0; 00109 00110 /** 00111 * isIdle 00112 * 00113 * Call to find out of the ASR-1 is currently handling a request. 00114 * 00115 * @return true if an operation is in progress 00116 */ 00117 virtual bool isIdle() = 0; 00118 00119 /** 00120 * mcuISR 00121 * 00122 * Called by your sketch to pass the interrupt along to afLib. 00123 */ 00124 virtual void mcuISR() = 0; 00125 }; 00126 #endif //AFLIB_IAFLIB_H
Generated on Wed Jul 13 2022 12:25:10 by
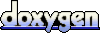