
Added a GPIO to power on/off for external I2C sensor(s) (with LEDs)
Dependencies: UniGraphic mbed vt100
SMTC502AT.cpp
00001 #include "mbed.h" 00002 #include "SMTC502AT.h" 00003 00004 SMTC502AT::SMTC502AT(AnalogIn *ain, float R0, float R1, float B, float T0) 00005 { 00006 _ain = ain ; 00007 _r0 = R0 ; 00008 _r1 = R1 ; 00009 _b = B ; 00010 _t0 = T0 ; 00011 } 00012 00013 SMTC502AT::~SMTC502AT(void) 00014 { 00015 if (_ain) { 00016 delete _ain ; 00017 } 00018 } 00019 00020 /** 00021 * getTemp returns the temperature 00022 * operational temperature is -50C to +105C 00023 */ 00024 float SMTC502AT::getTemp(void) 00025 { 00026 float result = 0.0 ; 00027 float f, raw, rr1, t ; 00028 if (_ain) { 00029 f = _ain->read() ; 00030 #if 0 00031 if (f < 0.087) { /* +105C */ 00032 printf("Temp is Too high or the sensor is absent\n") ; 00033 f = 0.087 ; 00034 } 00035 if (f > 0.978) { /* -50C */ 00036 printf("Temp is Too low or the sensor encountered a problem\n") ; 00037 f = 0.978 ; 00038 } 00039 #endif 00040 raw = f * 3.3 ; 00041 rr1 = _r1 * raw / (3.3 - raw) ; 00042 t = 1.0 / (log(rr1 / _r0) / _b + (1/_t0)) ; 00043 result = t - 273.15 ; 00044 } 00045 return( result ) ; 00046 } 00047
Generated on Wed Jul 13 2022 12:25:10 by
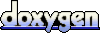