
I2C hang recover function added
Dependencies: UniGraphic mbed vt100
edge_pressure.h
00001 #ifndef _EDGE_PRESSURE_H_ 00002 #define _EDGE_PRESSURE_H_ 00003 #include "mbed.h" 00004 #include "edge_sensor.h" 00005 #include "PSE530.h" 00006 00007 /** 00008 * edge_pressure edge_sensor for measuring gas presssure 00009 */ 00010 00011 class edge_pressure : public edge_sensor { 00012 public: 00013 /** 00014 * constructor 00015 * @param *pse PSE530 pressure sensor object 00016 */ 00017 edge_pressure(PSE530 *pse, DigitalOut *en) ; 00018 00019 /** 00020 * destructor 00021 */ 00022 ~edge_pressure(void) ; 00023 00024 /** 00025 * reset and clear internal values 00026 */ 00027 virtual void reset(void) ; 00028 00029 /** 00030 * prepare for sampling (not used) 00031 */ 00032 virtual void prepare(void) ; 00033 00034 /** 00035 * sample the value 00036 * @returns 0: success non-0: failure 00037 */ 00038 virtual int sample(void) ; 00039 00040 /** 00041 * deliver the sampled value to afero cloud 00042 */ 00043 virtual int deliver(void) ; 00044 00045 /** 00046 * show the value in the display (TFT) 00047 */ 00048 virtual void show(void) ; 00049 // virtual void send_config(void) ; /* send config data to cloud */ 00050 // virtual void recv_config(void) ; /* receive config data from cloud */ 00051 00052 /** 00053 * get_value sample sensor value and calcurate it to the metric value 00054 * @returns measured value in kgf/cm2 00055 */ 00056 float get_value(void) ; 00057 00058 /** 00059 * Set threshold mode 00060 * @param mode int 0: absolute value 1: relative value in percent 00061 */ 00062 void set_thr_mode(int mode) { _thr_mode = mode ; } 00063 00064 /** 00065 * Get threshold mode 00066 * @returns the mode 0: absolute value 1: relative value in percent 00067 */ 00068 int get_thr_mode(void) { return _thr_mode ; } 00069 00070 /** 00071 * Set higher threshold 00072 * @param thr_high int16_t the higher threshold 00073 */ 00074 void set_thr_high(int16_t thr_high) ; 00075 00076 /** 00077 * Get higher threshold, the value is calcurated with expected value 00078 * @param expected float the expected pressure value for current temperature 00079 */ 00080 float get_thr_high(float expected) ; 00081 00082 /** 00083 * Set lower threshold 00084 * @param thr_low int16_t the lower threshold 00085 */ 00086 void set_thr_low(int16_t thr_low) ; 00087 00088 /** 00089 * Get lower threshold, the value is calcurated with expected value 00090 * @param expected float the expected pressure value for current temperature 00091 */ 00092 float get_thr_low(float expected) ; 00093 00094 /** 00095 * draw triangle pointer for GAS pressure mode display 00096 */ 00097 00098 void drawPointer(int c) ; 00099 00100 private: 00101 PSE530 *_pse ; 00102 DigitalOut *_en ; 00103 float _value ; 00104 float _thr_high ; 00105 float _thr_low ; 00106 int _thr_mode ; 00107 float _expected ; 00108 float _higher ; 00109 float _lower ; 00110 } ; 00111 00112 float temp2expected(float temp) ; 00113 extern float *current_temp ; 00114 00115 #endif /* _EDGE_PRESSURE_H_ */
Generated on Sat Jul 16 2022 08:26:44 by
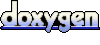