
I2C hang recover function added
Dependencies: UniGraphic mbed vt100
StatusCommand.cpp
00001 /** 00002 * Copyright 2015 Afero, Inc. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "StatusCommand.h" 00018 00019 #define SERIAL_PRINT_DBG_ASR_ON 0 00020 00021 StatusCommand::StatusCommand(uint16_t bytesToSend) { 00022 _cmd = 0x30; 00023 _bytesToSend = bytesToSend; 00024 _bytesToRecv = 0; 00025 } 00026 00027 StatusCommand::StatusCommand() { 00028 _cmd = 0x30; 00029 _bytesToSend = 0; 00030 _bytesToRecv = 0; 00031 } 00032 00033 StatusCommand::~StatusCommand() { 00034 } 00035 00036 uint16_t StatusCommand::getSize() { 00037 return sizeof(_cmd) + sizeof(_bytesToSend) + sizeof(_bytesToRecv); 00038 } 00039 00040 uint16_t StatusCommand::getBytes(int *bytes) { 00041 int index = 0; 00042 00043 bytes[index++] = (_cmd); 00044 bytes[index++] = (_bytesToSend & 0xff); 00045 bytes[index++] = ((_bytesToSend >> 8) & 0xff); 00046 bytes[index++] = (_bytesToRecv & 0xff); 00047 bytes[index++] = ((_bytesToRecv >> 8) & 0xff); 00048 00049 return index; 00050 } 00051 00052 uint8_t StatusCommand::calcChecksum() { 00053 uint8_t result = 0; 00054 00055 result += (_cmd); 00056 result += (_bytesToSend & 0xff); 00057 result += ((_bytesToSend >> 8) & 0xff); 00058 result += (_bytesToRecv & 0xff); 00059 result += ((_bytesToRecv >> 8) & 0xff); 00060 00061 return result; 00062 } 00063 00064 void StatusCommand::setChecksum(uint8_t checksum) { 00065 _checksum = checksum; 00066 } 00067 00068 uint8_t StatusCommand::getChecksum() { 00069 uint8_t result = 0; 00070 00071 result += (_cmd); 00072 result += (_bytesToSend & 0xff); 00073 result += ((_bytesToSend >> 8) & 0xff); 00074 result += (_bytesToRecv & 0xff); 00075 result += ((_bytesToRecv >> 8) & 0xff); 00076 00077 return result; 00078 } 00079 00080 void StatusCommand::setAck(bool ack) { 00081 _cmd = ack ? 0x31 : 0x30; 00082 } 00083 00084 void StatusCommand::setBytesToSend(uint16_t bytesToSend) { 00085 _bytesToSend = bytesToSend; 00086 } 00087 00088 uint16_t StatusCommand::getBytesToSend() { 00089 return _bytesToSend; 00090 } 00091 00092 void StatusCommand::setBytesToRecv(uint16_t bytesToRecv) { 00093 _bytesToRecv = bytesToRecv; 00094 } 00095 00096 uint16_t StatusCommand::getBytesToRecv() { 00097 return _bytesToRecv; 00098 } 00099 00100 bool StatusCommand::equals(StatusCommand *statusCommand) { 00101 return (_cmd == statusCommand->_cmd && _bytesToSend == statusCommand->_bytesToSend && 00102 _bytesToRecv == statusCommand->_bytesToRecv); 00103 } 00104 00105 bool StatusCommand::isValid() { 00106 return (_checksum == calcChecksum()) && (_cmd == 0x30 || _cmd == 0x31); 00107 } 00108 00109 void StatusCommand::dumpBytes() { 00110 #if SERIAL_PRINT_DBG_ASR_ON 00111 int len = getSize(); 00112 int bytes[len]; 00113 getBytes(bytes); 00114 00115 printf("len : %d\n",len); 00116 printf("data : "); 00117 for (int i = 0; i < len; i++) { 00118 if (i > 0) { 00119 printf(", "); 00120 } 00121 int b = bytes[i] & 0xff; 00122 printf("0x%02x", b) ; 00123 } 00124 printf("\n") ; 00125 #endif 00126 } 00127 00128 void StatusCommand::dump() { 00129 #if SERIAL_PRINT_DBG_ASR_ON 00130 printf("cmd : %s\n",_cmd == 0x30 ? "STATUS" : "STATUS_ACK"); 00131 printf("bytes to send : %d\n",_bytesToSend); 00132 printf("bytes to receive : %d\n",_bytesToRecv); 00133 #endif 00134 } 00135
Generated on Sat Jul 16 2022 08:26:44 by
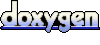