
I2C hang recover function added
Dependencies: UniGraphic mbed vt100
MMA8451Q.h
00001 #ifndef _MMA8451Q_H_ 00002 #define _MMA8451Q_H_ 00003 00004 #include "mbed.h" 00005 /** 00006 * MMA8451Q 3-Axis, 14-bit/8-bit Digital Accelerometer 00007 */ 00008 00009 class MMA8451Q { 00010 public: 00011 /** 00012 * MMA8451Q constructor 00013 * 00014 * @param i2c pointer to the I2C object 00015 * @param addr 7bit addr of the I2C peripheral 00016 */ 00017 MMA8451Q(I2C *i2c, int addr); 00018 00019 /** 00020 * MMA8451Q destructor 00021 */ 00022 ~MMA8451Q(); 00023 00024 /** 00025 * get all x, y, z data as int16_t 00026 * @param data[] three int16_t data will be returned 00027 * @returns I2C status 0: success others: I2C error 00028 */ 00029 int getAllRawData(int16_t data[]) ; 00030 00031 /** 00032 * get all x, y, z data as float 00033 * @param data three float data will be returned 00034 * @returns I2C status 0: success others: I2C error 00035 */ 00036 int getAllData(float value[]) ; 00037 int16_t getRawData(uint8_t addr) ; 00038 int16_t getRawX(void) ; 00039 int16_t getRawY(void) ; 00040 int16_t getRawZ(void) ; 00041 00042 float getAccX(void) ; 00043 float getAccY(void) ; 00044 float getAccZ(void) ; 00045 00046 private: 00047 I2C *p_i2c; 00048 int m_addr; 00049 int readRegs(int addr, uint8_t * data, int len); 00050 int writeRegs(uint8_t * data, int len); 00051 } ; 00052 00053 #endif /* _MMA8451Q_H_ */
Generated on Sat Jul 16 2022 08:26:44 by
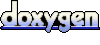