
I2C hang recover function added
Dependencies: UniGraphic mbed vt100
KL25Z_SystemInit.c
00001 #if defined (TARGET_KL25Z) 00002 /* 00003 ** ################################################################### 00004 ** Processor: MKL25Z128VLK4 00005 ** Compilers: ARM Compiler 00006 ** Freescale C/C++ for Embedded ARM 00007 ** GNU C Compiler 00008 ** IAR ANSI C/C++ Compiler for ARM 00009 ** 00010 ** Reference manual: KL25RM, Rev.1, Jun 2012 00011 ** Version: rev. 1.1, 2012-06-21 00012 ** 00013 ** Abstract: 00014 ** Provides a system configuration function and a global variable that 00015 ** contains the system frequency. It configures the device and initializes 00016 ** the oscillator (PLL) that is part of the microcontroller device. 00017 ** 00018 ** Copyright: 2012 Freescale Semiconductor, Inc. All Rights Reserved. 00019 ** 00020 ** http: www.freescale.com 00021 ** mail: support@freescale.com 00022 ** 00023 ** Revisions: 00024 ** - rev. 1.0 (2012-06-13) 00025 ** Initial version. 00026 ** - rev. 1.1 (2012-06-21) 00027 ** Update according to reference manual rev. 1. 00028 ** 00029 ** ################################################################### 00030 */ 00031 00032 /** 00033 * @file MKL25Z4 00034 * @version 1.1 00035 * @date 2012-06-21 00036 * @brief Device specific configuration file for MKL25Z4 (implementation file) 00037 * 00038 * Provides a system configuration function and a global variable that contains 00039 * the system frequency. It configures the device and initializes the oscillator 00040 * (PLL) that is part of the microcontroller device. 00041 */ 00042 00043 #include <stdint.h> 00044 #include "MKL25Z4.h" 00045 00046 //MODIFICATION: We DO want watchdog, uC default after reset is enabled with timeout=1024ms (2^10*LPO=1KHz) 00047 //#define DISABLE_WDOG 1 00048 00049 #define CLOCK_SETUP 1 00050 /* Predefined clock setups 00051 0 ... Multipurpose Clock Generator (MCG) in FLL Engaged Internal (FEI) mode 00052 Reference clock source for MCG module is the slow internal clock source 32.768kHz 00053 Core clock = 41.94MHz, BusClock = 13.98MHz 00054 1 ... Multipurpose Clock Generator (MCG) in PLL Engaged External (PEE) mode 00055 Reference clock source for MCG module is an external crystal 8MHz 00056 Core clock = 48MHz, BusClock = 24MHz 00057 2 ... Multipurpose Clock Generator (MCG) in Bypassed Low Power External (BLPE) mode 00058 Core clock/Bus clock derived directly from an external crystal 8MHz with no multiplication 00059 Core clock = 8MHz, BusClock = 8MHz 00060 3 ... Multipurpose Clock Generator (MCG) in FLL Engaged External (FEE) mode 00061 Reference clock source for MCG module is an external crystal 32.768kHz 00062 Core clock = 47.97MHz, BusClock = 23.98MHz 00063 This setup sets the RTC to be driven by the MCU clock directly without the need of an external source. 00064 RTC register values are retained when MCU is reset although there will be a slight (mSec's)loss of time 00065 accuracy durring the reset period. RTC will reset on power down. 00066 */ 00067 00068 /*---------------------------------------------------------------------------- 00069 Define clock source values 00070 *----------------------------------------------------------------------------*/ 00071 #if (CLOCK_SETUP == 0) 00072 #define CPU_XTAL_CLK_HZ 8000000u /* Value of the external crystal or oscillator clock frequency in Hz */ 00073 #define CPU_INT_SLOW_CLK_HZ 32768u /* Value of the slow internal oscillator clock frequency in Hz */ 00074 #define CPU_INT_FAST_CLK_HZ 4000000u /* Value of the fast internal oscillator clock frequency in Hz */ 00075 #define DEFAULT_SYSTEM_CLOCK 41943040u /* Default System clock value */ 00076 #elif (CLOCK_SETUP == 1) 00077 #define CPU_XTAL_CLK_HZ 8000000u /* Value of the external crystal or oscillator clock frequency in Hz */ 00078 #define CPU_INT_SLOW_CLK_HZ 32768u /* Value of the slow internal oscillator clock frequency in Hz */ 00079 #define CPU_INT_FAST_CLK_HZ 4000000u /* Value of the fast internal oscillator clock frequency in Hz */ 00080 #define DEFAULT_SYSTEM_CLOCK 48000000u /* Default System clock value */ 00081 #elif (CLOCK_SETUP == 2) 00082 #define CPU_XTAL_CLK_HZ 8000000u /* Value of the external crystal or oscillator clock frequency in Hz */ 00083 #define CPU_INT_SLOW_CLK_HZ 32768u /* Value of the slow internal oscillator clock frequency in Hz */ 00084 #define CPU_INT_FAST_CLK_HZ 4000000u /* Value of the fast internal oscillator clock frequency in Hz */ 00085 #define DEFAULT_SYSTEM_CLOCK 8000000u /* Default System clock value */ 00086 #elif (CLOCK_SETUP == 3) 00087 #define CPU_XTAL_CLK_HZ 32768u /* Value of the external crystal or oscillator clock frequency in Hz */ 00088 #define CPU_INT_SLOW_CLK_HZ 32768u /* Value of the slow internal oscillator clock frequency in Hz */ 00089 #define CPU_INT_FAST_CLK_HZ 4000000u /* Value of the fast internal oscillator clock frequency in Hz */ 00090 #define DEFAULT_SYSTEM_CLOCK 47972352u /* Default System clock value */ 00091 #endif /* (CLOCK_SETUP == 3) */ 00092 00093 /* ---------------------------------------------------------------------------- 00094 -- Core clock 00095 ---------------------------------------------------------------------------- */ 00096 00097 //MODIFICATION: That vartiable already exists 00098 // uint32_t SystemCoreClock = DEFAULT_SYSTEM_CLOCK; 00099 00100 /* ---------------------------------------------------------------------------- 00101 -- SystemInit() 00102 ---------------------------------------------------------------------------- */ 00103 00104 void $Sub$$SystemInit (void) { 00105 00106 //MODIFICATION: 00107 // That variable already exists, we set it here 00108 SystemCoreClock = DEFAULT_SYSTEM_CLOCK; 00109 // We want visual indication of boot time with red LED on 00110 //TODO 00111 00112 #if (DISABLE_WDOG) 00113 /* Disable the WDOG module */ 00114 /* SIM_COPC: COPT=0,COPCLKS=0,COPW=0 */ 00115 SIM->COPC = (uint32_t)0x00u; 00116 #endif /* (DISABLE_WDOG) */ 00117 #if (CLOCK_SETUP == 0) 00118 /* SIM->CLKDIV1: OUTDIV1=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,OUTDIV4=2,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0 */ 00119 SIM->CLKDIV1 = (uint32_t)0x00020000UL; /* Update system prescalers */ 00120 /* Switch to FEI Mode */ 00121 /* MCG->C1: CLKS=0,FRDIV=0,IREFS=1,IRCLKEN=1,IREFSTEN=0 */ 00122 MCG->C1 = (uint8_t)0x06U; 00123 /* MCG_C2: LOCRE0=0,??=0,RANGE0=0,HGO0=0,EREFS0=0,LP=0,IRCS=0 */ 00124 MCG->C2 = (uint8_t)0x00U; 00125 /* MCG->C4: DMX32=0,DRST_DRS=1 */ 00126 MCG->C4 = (uint8_t)((MCG->C4 & (uint8_t)~(uint8_t)0xC0U) | (uint8_t)0x20U); 00127 /* OSC0->CR: ERCLKEN=1,??=0,EREFSTEN=0,??=0,SC2P=0,SC4P=0,SC8P=0,SC16P=0 */ 00128 OSC0->CR = (uint8_t)0x80U; 00129 /* MCG->C5: ??=0,PLLCLKEN0=0,PLLSTEN0=0,PRDIV0=0 */ 00130 MCG->C5 = (uint8_t)0x00U; 00131 /* MCG->C6: LOLIE0=0,PLLS=0,CME0=0,VDIV0=0 */ 00132 MCG->C6 = (uint8_t)0x00U; 00133 while((MCG->S & MCG_S_IREFST_MASK) == 0x00U) { /* Check that the source of the FLL reference clock is the internal reference clock. */ 00134 } 00135 while((MCG->S & 0x0CU) != 0x00U) { /* Wait until output of the FLL is selected */ 00136 } 00137 #elif (CLOCK_SETUP == 1) 00138 /* SIM->SCGC5: PORTA=1 */ 00139 SIM->SCGC5 |= (uint32_t)0x0200UL; /* Enable clock gate for ports to enable pin routing */ 00140 /* SIM->CLKDIV1: OUTDIV1=1,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,OUTDIV4=1,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0 */ 00141 SIM->CLKDIV1 = (uint32_t)0x10010000UL; /* Update system prescalers */ 00142 /* PORTA->PCR18: ISF=0,MUX=0 */ 00143 PORTA->PCR[18] &= (uint32_t)~0x01000700UL; 00144 /* PORTA->PCR19: ISF=0,MUX=0 */ 00145 PORTA->PCR[19] &= (uint32_t)~0x01000700UL; 00146 /* Switch to FBE Mode */ 00147 /* OSC0->CR: ERCLKEN=1,??=0,EREFSTEN=0,??=0,SC2P=1,SC4P=0,SC8P=0,SC16P=1 */ 00148 OSC0->CR = (uint8_t)0x89U; 00149 /* MCG->C2: LOCRE0=0,??=0,RANGE0=2,HGO0=0,EREFS0=1,LP=0,IRCS=0 */ 00150 MCG->C2 = (uint8_t)0x24U; 00151 /* MCG->C1: CLKS=2,FRDIV=3,IREFS=0,IRCLKEN=1,IREFSTEN=0 */ 00152 MCG->C1 = (uint8_t)0x9AU; 00153 /* MCG->C4: DMX32=0,DRST_DRS=0 */ 00154 MCG->C4 &= (uint8_t)~(uint8_t)0xE0U; 00155 /* MCG->C5: ??=0,PLLCLKEN0=0,PLLSTEN0=0,PRDIV0=1 */ 00156 MCG->C5 = (uint8_t)0x01U; 00157 /* MCG->C6: LOLIE0=0,PLLS=0,CME0=0,VDIV0=0 */ 00158 MCG->C6 = (uint8_t)0x00U; 00159 while((MCG->S & MCG_S_IREFST_MASK) != 0x00U) { /* Check that the source of the FLL reference clock is the external reference clock. */ 00160 } 00161 while((MCG->S & 0x0CU) != 0x08U) { /* Wait until external reference clock is selected as MCG output */ 00162 } 00163 /* Switch to PBE Mode */ 00164 /* MCG->C6: LOLIE0=0,PLLS=1,CME0=0,VDIV0=0 */ 00165 MCG->C6 = (uint8_t)0x40U; 00166 while((MCG->S & 0x0CU) != 0x08U) { /* Wait until external reference clock is selected as MCG output */ 00167 } 00168 while((MCG->S & MCG_S_LOCK0_MASK) == 0x00U) { /* Wait until locked */ 00169 } 00170 /* Switch to PEE Mode */ 00171 /* MCG->C1: CLKS=0,FRDIV=3,IREFS=0,IRCLKEN=1,IREFSTEN=0 */ 00172 MCG->C1 = (uint8_t)0x1AU; 00173 while((MCG->S & 0x0CU) != 0x0CU) { /* Wait until output of the PLL is selected */ 00174 } 00175 #elif (CLOCK_SETUP == 2) 00176 /* SIM->SCGC5: PORTA=1 */ 00177 SIM->SCGC5 |= (uint32_t)0x0200UL; /* Enable clock gate for ports to enable pin routing */ 00178 /* SIM->CLKDIV1: OUTDIV1=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,OUTDIV4=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0 */ 00179 SIM->CLKDIV1 = (uint32_t)0x00000000UL; /* Update system prescalers */ 00180 /* PORTA->PCR18: ISF=0,MUX=0 */ 00181 PORTA->PCR[18] &= (uint32_t)~0x01000700UL; 00182 /* PORTA->PCR19: ISF=0,MUX=0 */ 00183 PORTA->PCR[19] &= (uint32_t)~0x01000700UL; 00184 /* Switch to FBE Mode */ 00185 /* OSC0->CR: ERCLKEN=1,??=0,EREFSTEN=0,??=0,SC2P=1,SC4P=0,SC8P=0,SC16P=1 */ 00186 OSC0->CR = (uint8_t)0x89U; 00187 /* MCG->C2: LOCRE0=0,??=0,RANGE0=2,HGO0=0,EREFS0=1,LP=0,IRCS=0 */ 00188 MCG->C2 = (uint8_t)0x24U; 00189 /* MCG->C1: CLKS=2,FRDIV=3,IREFS=0,IRCLKEN=1,IREFSTEN=0 */ 00190 MCG->C1 = (uint8_t)0x9AU; 00191 /* MCG->C4: DMX32=0,DRST_DRS=0 */ 00192 MCG->C4 &= (uint8_t)~(uint8_t)0xE0U; 00193 /* MCG->C5: ??=0,PLLCLKEN0=0,PLLSTEN0=0,PRDIV0=0 */ 00194 MCG->C5 = (uint8_t)0x00U; 00195 /* MCG->C6: LOLIE0=0,PLLS=0,CME0=0,VDIV0=0 */ 00196 MCG->C6 = (uint8_t)0x00U; 00197 while((MCG->S & MCG_S_IREFST_MASK) != 0x00U) { /* Check that the source of the FLL reference clock is the external reference clock. */ 00198 } 00199 while((MCG->S & 0x0CU) != 0x08U) { /* Wait until external reference clock is selected as MCG output */ 00200 } 00201 /* Switch to BLPE Mode */ 00202 /* MCG->C2: LOCRE0=0,??=0,RANGE0=2,HGO0=0,EREFS0=1,LP=1,IRCS=0 */ 00203 MCG->C2 = (uint8_t)0x26U; 00204 while((MCG->S & 0x0CU) != 0x08U) { /* Wait until external reference clock is selected as MCG output */ 00205 } 00206 #elif (CLOCK_SETUP == 3) 00207 /* SIM->SCGC5: PORTA=1 */ 00208 SIM->SCGC5 |= SIM_SCGC5_PORTA_MASK; /* Enable clock gate for ports to enable pin routing */ 00209 /* SIM->CLKDIV1: OUTDIV1=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,OUTDIV4=1,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0,??=0 */ 00210 SIM->CLKDIV1 = (SIM_CLKDIV1_OUTDIV1(0x00) | SIM_CLKDIV1_OUTDIV4(0x01)); /* Update system prescalers */ 00211 /* PORTA->PCR[3]: ISF=0,MUX=0 */ 00212 PORTA->PCR[3] &= (uint32_t)~(uint32_t)((PORT_PCR_ISF_MASK | PORT_PCR_MUX(0x07))); 00213 /* PORTA->PCR[4]: ISF=0,MUX=0 */ 00214 PORTA->PCR[4] &= (uint32_t)~(uint32_t)((PORT_PCR_ISF_MASK | PORT_PCR_MUX(0x07))); 00215 /* Switch to FEE Mode */ 00216 /* MCG->C2: LOCRE0=0,??=0,RANGE0=0,HGO0=0,EREFS0=1,LP=0,IRCS=0 */ 00217 MCG->C2 = (MCG_C2_RANGE0(0x00) | MCG_C2_EREFS0_MASK); 00218 /* OSC0->CR: ERCLKEN=1,??=0,EREFSTEN=0,??=0,SC2P=0,SC4P=0,SC8P=0,SC16P=0 */ 00219 OSC0->CR = OSC_CR_ERCLKEN_MASK | OSC_CR_SC16P_MASK | OSC_CR_SC4P_MASK | OSC_CR_SC2P_MASK; 00220 /* MCG->C1: CLKS=0,FRDIV=0,IREFS=0,IRCLKEN=1,IREFSTEN=0 */ 00221 MCG->C1 = (MCG_C1_CLKS(0x00) | MCG_C1_FRDIV(0x00) | MCG_C1_IRCLKEN_MASK); 00222 /* MCG->C4: DMX32=1,DRST_DRS=1 */ 00223 MCG->C4 = (uint8_t)((MCG->C4 & (uint8_t)~(uint8_t)( 00224 MCG_C4_DRST_DRS(0x02) 00225 )) | (uint8_t)( 00226 MCG_C4_DMX32_MASK | 00227 MCG_C4_DRST_DRS(0x01) 00228 )); 00229 while((MCG->S & MCG_S_IREFST_MASK) != 0x00U) { /* Check that the source of the FLL reference clock is the external reference clock. */ 00230 } 00231 while((MCG->S & 0x0CU) != 0x00U) { /* Wait until output of the FLL is selected */ 00232 } 00233 #endif /* (CLOCK_SETUP == 3) */ 00234 } 00235 #endif // TARGET_KL25Z
Generated on Sat Jul 16 2022 08:26:44 by
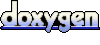