LPS22HH pressure sensor library
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
lps22hh_reg.h
00001 /* 00002 ****************************************************************************** 00003 * @file lps22hh_reg.h 00004 * @author Sensors Software Solution Team 00005 * @brief This file contains all the functions prototypes for the 00006 * lps22hh_reg.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2018 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without 00013 * modification, are permitted provided that the following conditions 00014 * are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright 00018 * notice, this list of conditions and the following disclaimer in the 00019 * documentation and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its 00021 * contributors may be used to endorse or promote products derived from 00022 * this software without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00027 * ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE 00028 * LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00029 * CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF 00030 * SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00031 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00032 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 00033 * ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00034 * POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef LPS22HH_DRIVER_H 00040 #define LPS22HH_DRIVER_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include <stdint.h> 00048 #include <math.h> 00049 00050 /** @addtogroup LPS22HH 00051 * @{ 00052 * 00053 */ 00054 00055 /** @defgroup LPS22HH_sensors_common_types 00056 * @{ 00057 * 00058 */ 00059 00060 #ifndef MEMS_SHARED_TYPES 00061 #define MEMS_SHARED_TYPES 00062 00063 /** 00064 * @defgroup axisXbitXX_t 00065 * @brief These unions are useful to represent different sensors data type. 00066 * These unions are not need by the driver. 00067 * 00068 * REMOVING the unions you are compliant with: 00069 * MISRA-C 2012 [Rule 19.2] -> " Union are not allowed " 00070 * 00071 * @{ 00072 * 00073 */ 00074 00075 typedef union { 00076 int16_t i16bit[3]; 00077 uint8_t u8bit[6]; 00078 } axis3bit16_t; 00079 00080 typedef union { 00081 int16_t i16bit; 00082 uint8_t u8bit[2]; 00083 } axis1bit16_t; 00084 00085 typedef union { 00086 int32_t i32bit[3]; 00087 uint8_t u8bit[12]; 00088 } axis3bit32_t; 00089 00090 typedef union { 00091 int32_t i32bit; 00092 uint8_t u8bit[4]; 00093 } axis1bit32_t; 00094 00095 /** 00096 * @} 00097 * 00098 */ 00099 00100 typedef struct { 00101 uint8_t bit0 : 1; 00102 uint8_t bit1 : 1; 00103 uint8_t bit2 : 1; 00104 uint8_t bit3 : 1; 00105 uint8_t bit4 : 1; 00106 uint8_t bit5 : 1; 00107 uint8_t bit6 : 1; 00108 uint8_t bit7 : 1; 00109 } bitwise_t; 00110 00111 #define PROPERTY_DISABLE (0U) 00112 #define PROPERTY_ENABLE (1U) 00113 00114 #endif /* MEMS_SHARED_TYPES */ 00115 00116 /** 00117 * @} 00118 * 00119 */ 00120 00121 /** @addtogroup LPS22HH_Interfaces_Functions 00122 * @brief This section provide a set of functions used to read and 00123 * write a generic register of the device. 00124 * MANDATORY: return 0 -> no Error. 00125 * @{ 00126 * 00127 */ 00128 00129 typedef int32_t (*lps22hh_write_ptr)(void *, uint8_t, uint8_t *, uint16_t); 00130 typedef int32_t (*lps22hh_read_ptr)(void *, uint8_t, uint8_t *, uint16_t); 00131 00132 typedef struct { 00133 /** Component mandatory fields **/ 00134 lps22hh_write_ptr write_reg; 00135 lps22hh_read_ptr read_reg; 00136 /** Customizable optional pointer **/ 00137 void *handle; 00138 } lps22hh_ctx_t; 00139 00140 /** 00141 * @} 00142 * 00143 */ 00144 00145 /** @defgroup LPS22HH_Infos 00146 * @{ 00147 * 00148 */ 00149 00150 /** I2C Device Address 8 bit format if SA0=0 -> B9 if SA0=1 -> BB **/ 00151 #define LPS22HH_I2C_ADD_H 0xBBU 00152 #define LPS22HH_I2C_ADD_L 0xB9U 00153 00154 /** Device Identification (Who am I) **/ 00155 #define LPS22HH_ID 0xB3U 00156 00157 /** 00158 * @} 00159 * 00160 */ 00161 00162 /** 00163 * @addtogroup LPS22HH_Sensitivity 00164 * @brief These macro are maintained for back compatibility. 00165 * in order to convert data into engineering units please 00166 * use functions: 00167 * -> _from_lsb_to_hpa(int16_t lsb) 00168 * -> _from_lsb_to_celsius(int16_t lsb); 00169 * 00170 * REMOVING the MACRO you are compliant with: 00171 * MISRA-C 2012 [Dir 4.9] -> " avoid function-like macros " 00172 * @{ 00173 * 00174 */ 00175 00176 #define LPS22HH_FROM_LSB_TO_hPa(lsb) (float)( lsb / 4096.0f ) 00177 #define LPS22HH_FROM_LSB_TO_degC(lsb) (float)( lsb / 100.0f ) 00178 00179 /** 00180 * @} 00181 * 00182 */ 00183 00184 #define LPS22HH_INTERRUPT_CFG 0x0BU 00185 typedef struct { 00186 uint8_t pe : 2; /* ple + phe */ 00187 uint8_t lir : 1; 00188 uint8_t diff_en : 1; 00189 uint8_t reset_az : 1; 00190 uint8_t autozero : 1; 00191 uint8_t reset_arp : 1; 00192 uint8_t autorefp : 1; 00193 } lps22hh_interrupt_cfg_t; 00194 00195 #define LPS22HH_THS_P_L 0x0CU 00196 typedef struct { 00197 uint8_t ths : 8; 00198 } lps22hh_ths_p_l_t; 00199 00200 #define LPS22HH_THS_P_H 0x0DU 00201 typedef struct { 00202 uint8_t ths : 7; 00203 uint8_t not_used_01 : 1; 00204 } lps22hh_ths_p_h_t; 00205 00206 #define LPS22HH_IF_CTRL 0x0EU 00207 typedef struct { 00208 uint8_t i2c_disable : 1; 00209 uint8_t i3c_disable : 1; 00210 uint8_t pd_dis_int1 : 1; 00211 uint8_t sdo_pu_en : 1; 00212 uint8_t sda_pu_en : 1; 00213 uint8_t not_used_01 : 2; 00214 uint8_t int_en_i3c : 1; 00215 } lps22hh_if_ctrl_t; 00216 00217 #define LPS22HH_WHO_AM_I 0x0FU 00218 #define LPS22HH_CTRL_REG1 0x10U 00219 typedef struct { 00220 uint8_t sim : 1; 00221 uint8_t bdu : 1; 00222 uint8_t lpfp_cfg : 2; /* en_lpfp + lpfp_cfg */ 00223 uint8_t odr : 3; 00224 uint8_t not_used_01 : 1; 00225 } lps22hh_ctrl_reg1_t; 00226 00227 #define LPS22HH_CTRL_REG2 0x11U 00228 typedef struct { 00229 uint8_t one_shot : 1; 00230 uint8_t low_noise_en : 1; 00231 uint8_t swreset : 1; 00232 uint8_t not_used_01 : 1; 00233 uint8_t if_add_inc : 1; 00234 uint8_t pp_od : 1; 00235 uint8_t int_h_l : 1; 00236 uint8_t boot : 1; 00237 } lps22hh_ctrl_reg2_t; 00238 00239 #define LPS22HH_CTRL_REG3 0x12U 00240 typedef struct { 00241 uint8_t int_s : 2; 00242 uint8_t drdy : 1; 00243 uint8_t int_f_ovr : 1; 00244 uint8_t int_f_wtm : 1; 00245 uint8_t int_f_full : 1; 00246 uint8_t not_used_01 : 2; 00247 } lps22hh_ctrl_reg3_t; 00248 00249 #define LPS22HH_FIFO_CTRL 0x13U 00250 typedef struct { 00251 uint8_t f_mode : 3; /* f_mode + trig_modes */ 00252 uint8_t stop_on_wtm : 1; 00253 uint8_t not_used_01 : 4; 00254 } lps22hh_fifo_ctrl_t; 00255 00256 #define LPS22HH_FIFO_WTM 0x14U 00257 typedef struct { 00258 uint8_t wtm : 7; 00259 uint8_t not_used_01 : 1; 00260 } lps22hh_fifo_wtm_t; 00261 00262 #define LPS22HH_REF_P_XL 0x15U 00263 #define LPS22HH_REF_P_L 0x16U 00264 #define LPS22HH_RPDS_L 0x18U 00265 #define LPS22HH_RPDS_H 0x19U 00266 #define LPS22HH_INT_SOURCE 0x24U 00267 typedef struct { 00268 uint8_t ph : 1; 00269 uint8_t pl : 1; 00270 uint8_t ia : 1; 00271 uint8_t not_used_01 : 5; 00272 } lps22hh_int_source_t; 00273 00274 #define LPS22HH_FIFO_STATUS1 0x25U 00275 #define LPS22HH_FIFO_STATUS2 0x26U 00276 typedef struct { 00277 uint8_t not_used_01 : 5; 00278 uint8_t fifo_full_ia : 1; 00279 uint8_t fifo_ovr_ia : 1; 00280 uint8_t fifo_wtm_ia : 1; 00281 } lps22hh_fifo_status2_t; 00282 00283 #define LPS22HH_STATUS 0x27U 00284 typedef struct { 00285 uint8_t p_da : 1; 00286 uint8_t t_da : 1; 00287 uint8_t not_used_01 : 2; 00288 uint8_t p_or : 1; 00289 uint8_t t_or : 1; 00290 uint8_t not_used_02 : 2; 00291 } lps22hh_status_t; 00292 00293 #define LPS22HH_PRESSURE_OUT_XL 0x28U 00294 #define LPS22HH_PRESSURE_OUT_L 0x29U 00295 #define LPS22HH_PRESSURE_OUT_H 0x2AU 00296 #define LPS22HH_TEMP_OUT_L 0x2BU 00297 #define LPS22HH_TEMP_OUT_H 0x2CU 00298 #define LPS22HH_FIFO_DATA_OUT_PRESS_XL 0x78U 00299 #define LPS22HH_FIFO_DATA_OUT_PRESS_L 0x79U 00300 #define LPS22HH_FIFO_DATA_OUT_PRESS_H 0x7AU 00301 #define LPS22HH_FIFO_DATA_OUT_TEMP_L 0x7BU 00302 #define LPS22HH_FIFO_DATA_OUT_TEMP_H 0x7CU 00303 00304 /** 00305 * @defgroup LPS22HH_Register_Union 00306 * @brief This union group all the registers that has a bitfield 00307 * description. 00308 * This union is useful but not need by the driver. 00309 * 00310 * REMOVING this union you are compliant with: 00311 * MISRA-C 2012 [Rule 19.2] -> " Union are not allowed " 00312 * 00313 * @{ 00314 * 00315 */ 00316 typedef union { 00317 lps22hh_interrupt_cfg_t interrupt_cfg; 00318 lps22hh_if_ctrl_t if_ctrl; 00319 lps22hh_ctrl_reg1_t ctrl_reg1; 00320 lps22hh_ctrl_reg2_t ctrl_reg2; 00321 lps22hh_ctrl_reg3_t ctrl_reg3; 00322 lps22hh_fifo_ctrl_t fifo_ctrl; 00323 lps22hh_fifo_wtm_t fifo_wtm; 00324 lps22hh_int_source_t int_source; 00325 lps22hh_fifo_status2_t fifo_status2; 00326 lps22hh_status_t status; 00327 bitwise_t bitwise; 00328 uint8_t byte; 00329 } lps22hh_reg_t; 00330 00331 /** 00332 * @} 00333 * 00334 */ 00335 00336 int32_t lps22hh_read_reg(lps22hh_ctx_t *ctx, uint8_t reg, uint8_t *data, 00337 uint16_t len); 00338 int32_t lps22hh_write_reg(lps22hh_ctx_t *ctx, uint8_t reg, uint8_t *data, 00339 uint16_t len); 00340 00341 extern float lps22hh_from_lsb_to_hpa(int16_t lsb); 00342 extern float lps22hh_from_lsb_to_celsius(int16_t lsb); 00343 00344 int32_t lps22hh_autozero_rst_set(lps22hh_ctx_t *ctx, uint8_t val); 00345 int32_t lps22hh_autozero_rst_get(lps22hh_ctx_t *ctx, uint8_t *val); 00346 00347 int32_t lps22hh_autozero_set(lps22hh_ctx_t *ctx, uint8_t val); 00348 int32_t lps22hh_autozero_get(lps22hh_ctx_t *ctx, uint8_t *val); 00349 00350 int32_t lps22hh_pressure_snap_rst_set(lps22hh_ctx_t *ctx, uint8_t val); 00351 int32_t lps22hh_pressure_snap_rst_get(lps22hh_ctx_t *ctx, uint8_t *val); 00352 00353 int32_t lps22hh_pressure_snap_set(lps22hh_ctx_t *ctx, uint8_t val); 00354 int32_t lps22hh_pressure_snap_get(lps22hh_ctx_t *ctx, uint8_t *val); 00355 00356 int32_t lps22hh_block_data_update_set(lps22hh_ctx_t *ctx, uint8_t val); 00357 int32_t lps22hh_block_data_update_get(lps22hh_ctx_t *ctx, uint8_t *val); 00358 00359 typedef enum { 00360 LPS22HH_POWER_DOWN = 0x00, 00361 LPS22HH_ONE_SHOOT = 0x08, 00362 LPS22HH_1_Hz = 0x01, 00363 LPS22HH_10_Hz = 0x02, 00364 LPS22HH_25_Hz = 0x03, 00365 LPS22HH_50_Hz = 0x04, 00366 LPS22HH_75_Hz = 0x05, 00367 LPS22HH_1_Hz_LOW_NOISE = 0x11, 00368 LPS22HH_10_Hz_LOW_NOISE = 0x12, 00369 LPS22HH_25_Hz_LOW_NOISE = 0x13, 00370 LPS22HH_50_Hz_LOW_NOISE = 0x14, 00371 LPS22HH_75_Hz_LOW_NOISE = 0x15, 00372 LPS22HH_100_Hz = 0x06, 00373 LPS22HH_200_Hz = 0x07, 00374 } lps22hh_odr_t; 00375 int32_t lps22hh_data_rate_set(lps22hh_ctx_t *ctx, lps22hh_odr_t val); 00376 int32_t lps22hh_data_rate_get(lps22hh_ctx_t *ctx, lps22hh_odr_t *val); 00377 00378 int32_t lps22hh_pressure_ref_set(lps22hh_ctx_t *ctx, uint8_t *buff); 00379 int32_t lps22hh_pressure_ref_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00380 00381 int32_t lps22hh_pressure_offset_set(lps22hh_ctx_t *ctx, uint8_t *buff); 00382 int32_t lps22hh_pressure_offset_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00383 00384 typedef struct { 00385 lps22hh_int_source_t int_source; 00386 lps22hh_fifo_status2_t fifo_status2; 00387 lps22hh_status_t status; 00388 } lps22hh_all_sources_t; 00389 int32_t lps22hh_all_sources_get(lps22hh_ctx_t *ctx, 00390 lps22hh_all_sources_t *val); 00391 00392 int32_t lps22hh_status_reg_get(lps22hh_ctx_t *ctx, lps22hh_status_t *val); 00393 00394 int32_t lps22hh_press_flag_data_ready_get(lps22hh_ctx_t *ctx, uint8_t *val); 00395 00396 int32_t lps22hh_temp_flag_data_ready_get(lps22hh_ctx_t *ctx, uint8_t *val); 00397 00398 int32_t lps22hh_pressure_raw_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00399 00400 int32_t lps22hh_temperature_raw_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00401 00402 int32_t lps22hh_fifo_pressure_raw_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00403 00404 int32_t lps22hh_fifo_temperature_raw_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00405 00406 int32_t lps22hh_device_id_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00407 00408 int32_t lps22hh_reset_set(lps22hh_ctx_t *ctx, uint8_t val); 00409 int32_t lps22hh_reset_get(lps22hh_ctx_t *ctx, uint8_t *val); 00410 00411 int32_t lps22hh_auto_increment_set(lps22hh_ctx_t *ctx, uint8_t val); 00412 int32_t lps22hh_auto_increment_get(lps22hh_ctx_t *ctx, uint8_t *val); 00413 00414 int32_t lps22hh_boot_set(lps22hh_ctx_t *ctx, uint8_t val); 00415 int32_t lps22hh_boot_get(lps22hh_ctx_t *ctx, uint8_t *val); 00416 00417 typedef enum { 00418 LPS22HH_LPF_ODR_DIV_2 = 0, 00419 LPS22HH_LPF_ODR_DIV_9 = 2, 00420 LPS22HH_LPF_ODR_DIV_20 = 3, 00421 } lps22hh_lpfp_cfg_t; 00422 int32_t lps22hh_lp_bandwidth_set(lps22hh_ctx_t *ctx, lps22hh_lpfp_cfg_t val); 00423 int32_t lps22hh_lp_bandwidth_get(lps22hh_ctx_t *ctx, lps22hh_lpfp_cfg_t *val); 00424 00425 typedef enum { 00426 LPS22HH_I2C_ENABLE = 0, 00427 LPS22HH_I2C_DISABLE = 1, 00428 } lps22hh_i2c_disable_t; 00429 int32_t lps22hh_i2c_interface_set(lps22hh_ctx_t *ctx, 00430 lps22hh_i2c_disable_t val); 00431 int32_t lps22hh_i2c_interface_get(lps22hh_ctx_t *ctx, 00432 lps22hh_i2c_disable_t *val); 00433 00434 typedef enum { 00435 LPS22HH_I3C_ENABLE = 0x00, 00436 LPS22HH_I3C_ENABLE_INT_PIN_ENABLE = 0x10, 00437 LPS22HH_I3C_DISABLE = 0x11, 00438 } lps22hh_i3c_disable_t; 00439 int32_t lps22hh_i3c_interface_set(lps22hh_ctx_t *ctx, 00440 lps22hh_i3c_disable_t val); 00441 int32_t lps22hh_i3c_interface_get(lps22hh_ctx_t *ctx, 00442 lps22hh_i3c_disable_t *val); 00443 00444 typedef enum { 00445 LPS22HH_PULL_UP_DISCONNECT = 0, 00446 LPS22HH_PULL_UP_CONNECT = 1, 00447 } lps22hh_pu_en_t; 00448 int32_t lps22hh_sdo_sa0_mode_set(lps22hh_ctx_t *ctx, lps22hh_pu_en_t val); 00449 int32_t lps22hh_sdo_sa0_mode_get(lps22hh_ctx_t *ctx, lps22hh_pu_en_t *val); 00450 int32_t lps22hh_sda_mode_set(lps22hh_ctx_t *ctx, lps22hh_pu_en_t val); 00451 int32_t lps22hh_sda_mode_get(lps22hh_ctx_t *ctx, lps22hh_pu_en_t *val); 00452 00453 typedef enum { 00454 LPS22HH_SPI_4_WIRE = 0, 00455 LPS22HH_SPI_3_WIRE = 1, 00456 } lps22hh_sim_t; 00457 int32_t lps22hh_spi_mode_set(lps22hh_ctx_t *ctx, lps22hh_sim_t val); 00458 int32_t lps22hh_spi_mode_get(lps22hh_ctx_t *ctx, lps22hh_sim_t *val); 00459 00460 typedef enum { 00461 LPS22HH_INT_PULSED = 0, 00462 LPS22HH_INT_LATCHED = 1, 00463 } lps22hh_lir_t; 00464 int32_t lps22hh_int_notification_set(lps22hh_ctx_t *ctx, lps22hh_lir_t val); 00465 int32_t lps22hh_int_notification_get(lps22hh_ctx_t *ctx, lps22hh_lir_t *val); 00466 00467 typedef enum { 00468 LPS22HH_PUSH_PULL = 0, 00469 LPS22HH_OPEN_DRAIN = 1, 00470 } lps22hh_pp_od_t; 00471 int32_t lps22hh_pin_mode_set(lps22hh_ctx_t *ctx, lps22hh_pp_od_t val); 00472 int32_t lps22hh_pin_mode_get(lps22hh_ctx_t *ctx, lps22hh_pp_od_t *val); 00473 00474 typedef enum { 00475 LPS22HH_ACTIVE_HIGH = 0, 00476 LPS22HH_ACTIVE_LOW = 1, 00477 } lps22hh_int_h_l_t; 00478 int32_t lps22hh_pin_polarity_set(lps22hh_ctx_t *ctx, lps22hh_int_h_l_t val); 00479 int32_t lps22hh_pin_polarity_get(lps22hh_ctx_t *ctx, lps22hh_int_h_l_t *val); 00480 00481 int32_t lps22hh_pin_int_route_set(lps22hh_ctx_t *ctx, 00482 lps22hh_ctrl_reg3_t *val); 00483 int32_t lps22hh_pin_int_route_get(lps22hh_ctx_t *ctx, 00484 lps22hh_ctrl_reg3_t *val); 00485 00486 typedef enum { 00487 LPS22HH_NO_THRESHOLD = 0, 00488 LPS22HH_POSITIVE = 1, 00489 LPS22HH_NEGATIVE = 2, 00490 LPS22HH_BOTH = 3, 00491 } lps22hh_pe_t; 00492 int32_t lps22hh_int_on_threshold_set(lps22hh_ctx_t *ctx, lps22hh_pe_t val); 00493 int32_t lps22hh_int_on_threshold_get(lps22hh_ctx_t *ctx, lps22hh_pe_t *val); 00494 00495 int32_t lps22hh_int_treshold_set(lps22hh_ctx_t *ctx, uint16_t buff); 00496 int32_t lps22hh_int_treshold_get(lps22hh_ctx_t *ctx, uint16_t *buff); 00497 00498 typedef enum { 00499 LPS22HH_BYPASS_MODE = 0, 00500 LPS22HH_FIFO_MODE = 1, 00501 LPS22HH_STREAM_MODE = 2, 00502 LPS22HH_DYNAMIC_STREAM_MODE = 3, 00503 LPS22HH_BYPASS_TO_FIFO_MODE = 5, 00504 LPS22HH_BYPASS_TO_STREAM_MODE = 6, 00505 LPS22HH_STREAM_TO_FIFO_MODE = 7, 00506 } lps22hh_f_mode_t; 00507 int32_t lps22hh_fifo_mode_set(lps22hh_ctx_t *ctx, lps22hh_f_mode_t val); 00508 int32_t lps22hh_fifo_mode_get(lps22hh_ctx_t *ctx, lps22hh_f_mode_t *val); 00509 00510 int32_t lps22hh_fifo_stop_on_wtm_set(lps22hh_ctx_t *ctx, uint8_t val); 00511 int32_t lps22hh_fifo_stop_on_wtm_get(lps22hh_ctx_t *ctx, uint8_t *val); 00512 00513 int32_t lps22hh_fifo_watermark_set(lps22hh_ctx_t *ctx, uint8_t val); 00514 int32_t lps22hh_fifo_watermark_get(lps22hh_ctx_t *ctx, uint8_t *val); 00515 00516 int32_t lps22hh_fifo_data_level_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00517 00518 int32_t lps22hh_fifo_src_get(lps22hh_ctx_t *ctx, lps22hh_fifo_status2_t *val); 00519 00520 int32_t lps22hh_fifo_full_flag_get(lps22hh_ctx_t *ctx, uint8_t *val); 00521 00522 int32_t lps22hh_fifo_ovr_flag_get(lps22hh_ctx_t *ctx, uint8_t *val); 00523 00524 int32_t lps22hh_fifo_wtm_flag_get(lps22hh_ctx_t *ctx, uint8_t *val); 00525 00526 int32_t lps22hh_fifo_ovr_on_int_set(lps22hh_ctx_t *ctx, uint8_t val); 00527 int32_t lps22hh_fifo_ovr_on_int_get(lps22hh_ctx_t *ctx, uint8_t *val); 00528 00529 int32_t lps22hh_fifo_threshold_on_int_set(lps22hh_ctx_t *ctx, uint8_t val); 00530 int32_t lps22hh_fifo_threshold_on_int_get(lps22hh_ctx_t *ctx, uint8_t *val); 00531 00532 int32_t lps22hh_fifo_full_on_int_set(lps22hh_ctx_t *ctx, uint8_t val); 00533 int32_t lps22hh_fifo_full_on_int_get(lps22hh_ctx_t *ctx, uint8_t *val); 00534 00535 /** 00536 * @} 00537 * 00538 */ 00539 00540 #ifdef __cplusplus 00541 } 00542 #endif 00543 00544 #endif /*LPS22HH_REGS_H */ 00545 00546 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 13 2022 17:27:40 by
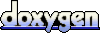