
Team Kryptonite EE300 Keypad Subsystem
Fork of mbed_can_bus_test1 by
main.cpp
00001 #include "mbed.h" 00002 #include <iostream> 00003 #include <cmath> 00004 #include <iomanip> 00005 00006 #include "Keypad.h" 00007 00008 Ticker ticker; 00009 Serial PC(USBTX, USBRX); 00010 DigitalOut led1(LED1); 00011 DigitalOut led2(LED2); 00012 CAN can1(p9,p10); 00013 CAN can2(p30,p29); 00014 DigitalIn pin21(p21); 00015 DigitalIn pin22(p22); 00016 DigitalIn pin23(p23); 00017 DigitalIn pin24(p24); 00018 DigitalIn pin25(p25); 00019 DigitalIn pin26(p26); 00020 DigitalIn pin27(p27); 00021 00022 00023 //Declare KeyPad GPIO pins here 00024 00025 char Keytable[] = { '1', '2', '3', //row0, p1, mbedpin21 00026 '4', '5', '6', //row1, p2, mbedpin22 00027 '7', '8', '9', //row2, p3, mbedpin23 00028 '*', '0', '#', //row3, p4, mbedpin24 00029 }; 00030 //col0, col1, col2 00031 //p5, p6, p7 00032 //mbedpin25, mbedpin26, mbedpin27 00033 00034 void functionone(int& Index, int& count); 00035 void functiontwo(); 00036 void functionthree(); 00037 00038 using namespace std; 00039 00040 int32_t Index; 00041 int32_t count = 0; 00042 00043 uint32_t cbAfterInput(uint32_t index) 00044 { 00045 Index = index; 00046 return 0; 00047 } 00048 00049 //Universal ID: 1337 00050 //CAN Message Info 00051 char counter = '0'; //keypad system CAN test variable 00052 char start = '*'; //Start 00053 char stop = '#'; //Stop / Reset 00054 char amp5 = '5'; //Digipot 00055 char amp4 = '4'; //Digipot 00056 char amp3 = '3'; //Digipot 00057 char amp2 = '2'; //Digipot 00058 char amp1 = '1'; //Digipot 00059 char sin10Hz = 'q'; //DAC - 00060 char sin1Hz = 'w'; //DAC - 00061 char sqr10Hz = 'o'; //DAC - 00062 char sqr1Hz = 't'; //DAC - 00063 char saveUSB = 'u'; //ADC -0 00064 char saveLocal = 'l'; //ADC That's an l as in labeorphily 00065 char keypress; 00066 00067 00068 //CAN Send (Parameter = message data) 00069 void send(char sendMsg) { 00070 if(can1.write(CANMessage(1337, &sendMsg, 1))) 00071 { 00072 printf("Command sent: %c\n\n", sendMsg); 00073 } 00074 led1 = !led1; 00075 } 00076 00077 00078 /* write Switch statements*/ 00079 //Setup Choice Switch Statements 00080 00081 void functionone(int& Index, int& count)// Get Keypad Input to change Index 00082 { 00083 while(count == Index) 00084 { 00085 Keypad keypad(p21, p22, p23, p24, p25, p26, p27, NC); 00086 keypad.attach(&cbAfterInput); 00087 keypad.start(); 00088 } 00089 count = Index; 00090 00091 } 00092 00093 void functiontwo() //DAC 00094 { 00095 00096 int reset = -1; 00097 00098 while(reset == -1) 00099 { 00100 cout << "-------------------------------------------------\n\r" << endl; 00101 cout << "For 10Hz Sine Wave select: 3\n\r" << endl; 00102 cout << "For 1Hz Sine Wave select: 4\n\r" << endl; 00103 cout << "For 10Hz Square Wave select: 5\n\r" << endl; 00104 cout << "For 1Hz Square Wave select: 6\n\r" << endl; 00105 00106 functionone(Index, count); 00107 00108 if (Index == 2) 00109 { 00110 cout << "10Hz Sine Wave has been selected \n\r" << endl; 00111 send(sin10Hz); 00112 reset = 1; 00113 } 00114 else if (Index == 3) 00115 { 00116 cout << "1Hz Sine Wave has been selected\n\r" << endl; 00117 send(sin1Hz); 00118 reset = 1; 00119 } 00120 else if (Index == 4) 00121 { 00122 cout << "10Hz Square Wave has been selected \n\r" << endl; 00123 send(sqr10Hz); 00124 reset = 1; 00125 } 00126 else if (Index == 5) 00127 { 00128 cout << "1Hz Square Wave has been selected \n\r" << endl; 00129 send(sqr1Hz); 00130 reset = 1; 00131 } 00132 else 00133 { 00134 reset = -1; 00135 } 00136 } 00137 } 00138 00139 void functionthree()//Digipot Config (select amplitude) 00140 { 00141 int flag = -1; 00142 00143 while (flag == -1) 00144 { 00145 cout << "-------------------------------------------------\n\r" << endl; 00146 cout << "Select Waveform Amplitude\n\r"; 00147 cout << "1V = 1,\n2V = 2,\n3V = 3,\n4V = 4,\n5v = 5\n\r" << endl; 00148 00149 functionone(Index, count); 00150 00151 if (Index == 0) 00152 { 00153 send(amp1); 00154 flag =1; 00155 } 00156 else if (Index == 1) 00157 { 00158 send(amp2); 00159 flag = 1; 00160 } 00161 else if (Index == 2) 00162 { 00163 send(amp3); 00164 flag = 1; 00165 } 00166 else if (Index == 3) 00167 { 00168 send(amp4); 00169 flag = 1; 00170 } 00171 else if (Index == 4) 00172 { 00173 send(amp5); 00174 flag = 1; 00175 } 00176 else 00177 { 00178 flag = -1; 00179 } 00180 } 00181 } 00182 00183 void functionfour() //ADC Config (Save Location Settings) 00184 { 00185 int flag = -1; 00186 00187 while(flag == -1) 00188 { 00189 cout << "-------------------------------------------------\n\r" << endl; 00190 cout << "Select Save Location:\n\r" << endl; 00191 cout << "Save Locally (default) = 5, Save to USB = 6\n\r" << endl; 00192 00193 functionone(Index, count); 00194 00195 if (Index == 4) 00196 { 00197 cout << "Save locally has been selected\n\r" << endl; 00198 send(saveLocal); 00199 flag = 1; 00200 } 00201 else if (Index == 5) 00202 { 00203 cout << "Save to USB has been selected\n\r" << endl; 00204 send(saveUSB); 00205 flag = 1; 00206 } 00207 else 00208 { 00209 flag =-1; 00210 } 00211 } 00212 } 00213 00214 void functionfive()//Start Stop Waveform Command Menu 00215 { 00216 int flag = -1; 00217 00218 while(flag == -1) 00219 { 00220 cout << "Start/Stop waveform: start = 7, stop = 8 \n\r" << endl; 00221 00222 functionone(Index, count); 00223 00224 if (Index ==6) 00225 { 00226 cout << "Wave has been started\n\r" << endl; 00227 send(start); 00228 flag = 1; 00229 } 00230 else if (Index == 7) 00231 { 00232 cout << "Wave has been stopped\n\r" << endl; 00233 send(stop); 00234 flag = 1; 00235 } 00236 else 00237 { 00238 flag = -1; 00239 } 00240 } 00241 } 00242 00243 00244 00245 00246 00247 int main() 00248 { 00249 cout << "EE 300 Embedded System Black Box Transfer Function Data Acquisition\n\r" << endl; 00250 while(1) 00251 { 00252 int flag2 = -1; 00253 00254 while(flag2 == -1) 00255 { 00256 cout << "-------------------------------------------------\n\r" << endl; 00257 cout << "Select Wave Type = 9,\nSelect Wave Amplitude = *,\n\r"; 00258 cout << "Select Save Location =0,\nStart/Stop Wave = #\n\r" << endl; 00259 functionone(Index, count); 00260 00261 if (Index == 8)//9 00262 { 00263 functiontwo();//DAC Config Function 00264 flag2 = 1; 00265 } 00266 else if (Index == 9)//* 00267 { 00268 functionthree();//Digipot Config 00269 flag2 = 1; 00270 } 00271 else if (Index == 10) //0 00272 { 00273 functionfour();//ADC Config 00274 flag2 = 1; 00275 } 00276 else if (Index == 11)//# 00277 { 00278 functionfive();//Start/Stop Waveform Function 00279 flag2 = 1; 00280 } 00281 else 00282 { 00283 flag2 = -1; 00284 } 00285 } 00286 00287 } 00288 }
Generated on Sat Jul 16 2022 21:47:45 by
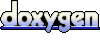