
Example of AWS IoT connection and Web Dashboard thru STM32 Nucleo evaluation board and mbed OS.
Dependencies: X_NUCLEO_IKS01A1 mbed FP MQTTPacket DnsQuery ATParser
WiFiInterface.h
00001 /* WiFiInterface 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef WIFI_INTERFACE_H 00018 #define WIFI_INTERFACE_H 00019 00020 #include "NetworkStack.h" 00021 00022 /** Enum of WiFi encryption types 00023 * 00024 * The security type specifies a particular security to use when 00025 * connected to a WiFi network 00026 * 00027 * @enum nsapi_protocol_t 00028 */ 00029 enum nsapi_security_t { 00030 NSAPI_SECURITY_NONE = 0, /*!< open access point */ 00031 NSAPI_SECURITY_WEP, /*!< phrase conforms to WEP */ 00032 NSAPI_SECURITY_WPA, /*!< phrase conforms to WPA */ 00033 NSAPI_SECURITY_WPA2, /*!< phrase conforms to WPA2 */ 00034 }; 00035 00036 /** WiFiInterface class 00037 * 00038 * Common interface that is shared between WiFi devices 00039 */ 00040 class WiFiInterface 00041 { 00042 public: 00043 /** Start the interface 00044 * 00045 * Attempts to connect to a WiFi network. If passphrase is invalid, 00046 * NSAPI_ERROR_AUTH_ERROR is returned. 00047 * 00048 * @param ssid Name of the network to connect to 00049 * @param pass Security passphrase to connect to the network 00050 * @param security Type of encryption for connection 00051 * @return 0 on success, negative error code on failure 00052 */ 00053 virtual int connect(const char *ssid, const char *pass, nsapi_security_t security = NSAPI_SECURITY_NONE) = 0; 00054 00055 /** Stop the interface 00056 * 00057 * @return 0 on success, negative error code on failure 00058 */ 00059 virtual int disconnect() = 0; 00060 00061 /** Get the local MAC address 00062 * 00063 * @return Null-terminated representation of the local MAC address 00064 */ 00065 virtual const char *get_mac_address() = 0; 00066 }; 00067 00068 #endif
Generated on Wed Jul 13 2022 20:28:49 by
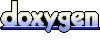