
Example of AWS IoT connection and Web Dashboard thru STM32 Nucleo evaluation board and mbed OS.
Dependencies: X_NUCLEO_IKS01A1 mbed FP MQTTPacket DnsQuery ATParser
UDPSocket.cpp
00001 /* Socket 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "UDPSocket.h" 00018 #include "Timer.h" 00019 00020 UDPSocket::UDPSocket() 00021 { 00022 } 00023 00024 UDPSocket::UDPSocket(NetworkStack *iface) 00025 { 00026 open(iface); 00027 } 00028 00029 int UDPSocket::open(NetworkStack *iface) 00030 { 00031 return Socket::open(iface, NSAPI_UDP); 00032 } 00033 00034 int UDPSocket::sendto(const char *host, uint16_t port, const void *data, unsigned size) 00035 { 00036 SocketAddress addr(_iface, host, port); 00037 if (!addr) { 00038 return NSAPI_ERROR_DNS_FAILURE; 00039 } 00040 00041 return sendto(addr, data, size); 00042 } 00043 00044 int UDPSocket::sendto(const SocketAddress &address, const void *data, unsigned size) 00045 { 00046 mbed::Timer timer; 00047 timer.start(); 00048 mbed::Timeout timeout; 00049 if (_timeout >= 0) { 00050 timeout.attach_us(&Socket::wakeup, _timeout * 1000); 00051 } 00052 00053 while (true) { 00054 if (!_socket) { 00055 return NSAPI_ERROR_NO_SOCKET; 00056 } 00057 00058 int sent = _iface->socket_sendto(_socket, address, data, size); 00059 if (sent != NSAPI_ERROR_WOULD_BLOCK 00060 || (_timeout >= 0 && timer.read_ms() >= _timeout)) { 00061 return sent; 00062 } 00063 00064 __WFI(); 00065 } 00066 } 00067 00068 int UDPSocket::recvfrom(SocketAddress *address, void *buffer, unsigned size) 00069 { 00070 mbed::Timer timer; 00071 timer.start(); 00072 mbed::Timeout timeout; 00073 if (_timeout >= 0) { 00074 timeout.attach_us(&Socket::wakeup, _timeout * 1000); 00075 } 00076 00077 while (true) { 00078 if (!_socket) { 00079 return NSAPI_ERROR_NO_SOCKET; 00080 } 00081 00082 int recv = _iface->socket_recvfrom(_socket, address, buffer, size); 00083 if (recv != NSAPI_ERROR_WOULD_BLOCK 00084 || (_timeout >= 0 && timer.read_ms() >= _timeout)) { 00085 return recv; 00086 } 00087 00088 __WFI(); 00089 } 00090 }
Generated on Wed Jul 13 2022 20:28:49 by
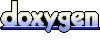