
BMS_T2
Dependencies: INA226
LTC681x.h
00001 /* 00002 General BMS Library 00003 LTC681x.h 00004 */ 00005 #ifndef LTC681X_H 00006 #define LTC681X_H 00007 00008 #include "mbed.h" 00009 //#include <stdint.h> 00010 #include "bms.h" 00011 //#include "LT_SPI.h" 00012 00013 //#define MBED 00014 //#ifdef MBED 00015 //#include "mbed.h"//remove when using with LINDUINO 00016 //#endif 00017 00018 //#ifdef LINDUINO 00019 //#include <Arduino.h> 00020 //#endif 00021 00022 //#define IC_LTC6813 00023 00024 #define MD_422HZ_1KHZ 0 00025 #define MD_27KHZ_14KHZ 1 00026 #define MD_7KHZ_3KHZ 2 00027 #define MD_26HZ_2KHZ 3 00028 00029 #define ADC_OPT_ENABLED 1 00030 #define ADC_OPT_DISABLED 0 00031 00032 #define CELL_CH_ALL 0 00033 #define CELL_CH_1and7 1 00034 #define CELL_CH_2and8 2 00035 #define CELL_CH_3and9 3 00036 #define CELL_CH_4and10 4 00037 #define CELL_CH_5and11 5 00038 #define CELL_CH_6and12 6 00039 00040 #define SELFTEST_1 1 00041 #define SELFTEST_2 2 00042 00043 #define AUX_CH_ALL 0 00044 #define AUX_CH_GPIO1 1 00045 #define AUX_CH_GPIO2 2 00046 #define AUX_CH_GPIO3 3 00047 #define AUX_CH_GPIO4 4 00048 #define AUX_CH_GPIO5 5 00049 #define AUX_CH_VREF2 6 00050 00051 #define STAT_CH_ALL 0 00052 #define STAT_CH_SOC 1 00053 #define STAT_CH_ITEMP 2 00054 #define STAT_CH_VREGA 3 00055 #define STAT_CH_VREGD 4 00056 00057 #define DCP_DISABLED 0 00058 #define DCP_ENABLED 1 00059 00060 #define PULL_UP_CURRENT 1 00061 #define PULL_DOWN_CURRENT 0 00062 00063 00064 00065 #define NUM_RX_BYT 8 00066 #define CELL 1 00067 #define AUX 2 00068 #define STAT 3 00069 #define CFGR 0 00070 #define CFGRB 4 00071 #define CS_PIN 10 00072 00073 //! Cell Voltage data structure. 00074 typedef struct 00075 { 00076 uint16_t c_codes[18];//!< Cell Voltage Codes 00077 uint8_t pec_match[6];//!< If a PEC error was detected during most recent read cmd 00078 } cv; 00079 00080 //! AUX Reg Voltage Data 00081 typedef struct 00082 { 00083 uint16_t a_codes[9];//!< Aux Voltage Codes 00084 uint8_t pec_match[4];//!< If a PEC error was detected during most recent read cmd 00085 } ax; 00086 00087 typedef struct 00088 { 00089 uint16_t stat_codes[4];//!< A two dimensional array of the stat voltage codes. 00090 uint8_t flags[3]; //!< byte array that contains the uv/ov flag data 00091 uint8_t mux_fail[1]; //!< Mux self test status flag 00092 uint8_t thsd[1]; //!< Thermal shutdown status 00093 uint8_t pec_match[2];//!< If a PEC error was detected during most recent read cmd 00094 } st; 00095 00096 typedef struct 00097 { 00098 uint8_t tx_data[6]; 00099 uint8_t rx_data[8]; 00100 uint8_t rx_pec_match;//!< If a PEC error was detected during most recent read cmd 00101 } ic_register; 00102 00103 typedef struct 00104 { 00105 uint16_t pec_count; 00106 uint16_t cfgr_pec; 00107 uint16_t cell_pec[6]; 00108 uint16_t aux_pec[4]; 00109 uint16_t stat_pec[2]; 00110 } pec_counter; 00111 00112 typedef struct 00113 { 00114 uint8_t cell_channels; 00115 uint8_t stat_channels; 00116 uint8_t aux_channels; 00117 uint8_t num_cv_reg; 00118 uint8_t num_gpio_reg; 00119 uint8_t num_stat_reg; 00120 } register_cfg; 00121 00122 typedef struct 00123 { 00124 00125 ic_register config; 00126 ic_register configb; 00127 cv cells; 00128 ax aux; 00129 st stat; 00130 ic_register com; 00131 ic_register pwm; 00132 ic_register pwmb; 00133 ic_register sctrl; 00134 ic_register sctrlb; 00135 bool isospi_reverse; 00136 pec_counter crc_count; 00137 register_cfg ic_reg; 00138 long system_open_wire; 00139 } cell_asic; 00140 00141 00142 00143 00144 /*! calculates and returns the CRC15 00145 @returns The calculated pec15 as an unsigned int 00146 */ 00147 uint16_t pec15_calc(uint8_t len, //!< the length of the data array being passed to the function 00148 uint8_t *data //!< the array of data that the PEC will be generated from 00149 ); 00150 00151 /*! Wake isoSPI up from idle state */ 00152 void wakeup_idle(uint8_t total_ic);//!< number of ICs in the daisy chain 00153 00154 /*! Wake the LTC6813 from the sleep state */ 00155 void wakeup_sleep(uint8_t total_ic); //!< number of ICs in the daisy chain 00156 00157 /*! Sense a command to the bms IC. This code will calculate the PEC code for the transmitted command*/ 00158 void cmd_68(uint8_t tx_cmd[2]); //!< 2 Byte array containing the BMS command to be sent 00159 00160 //! Writes an array of data to the daisy chain 00161 void write_68(uint8_t total_ic , //!< number of ICs in the daisy chain 00162 uint8_t tx_cmd[2], //!< 2 Byte array containing the BMS command to be sent 00163 uint8_t data[] //!< Array containing the data to be written to the BMS ICs 00164 ); 00165 //! Issues a command onto the daisy chain and reads back 6*total_ic data in the rx_data array 00166 int8_t read_68( uint8_t total_ic, //!< number of ICs in the daisy chain 00167 uint8_t tx_cmd[2], //!< 2 Byte array containing the BMS command to be sent 00168 uint8_t *rx_data); //!< Array that the read back data will be stored. 00169 00170 /*! Starts the Mux Decoder diagnostic self test 00171 00172 Running this command will start the Mux Decoder Diagnostic Self Test 00173 This test takes roughly 1mS to complete. The MUXFAIL bit will be updated, 00174 the bit will be set to 1 for a failure and 0 if the test has been passed. 00175 */ 00176 void LTC681x_diagn(); 00177 00178 //! Sends the poll adc command 00179 //! @returns 1 byte read back after a pladc command. If the byte is not 0xFF ADC conversion has completed 00180 uint8_t LTC681x_pladc(); 00181 00182 //! This function will block operation until the ADC has finished it's conversion 00183 //! @returns the approximate time it took for the ADC function to complete. 00184 uint32_t LTC681x_pollAdc(); 00185 00186 /*! Starts cell voltage conversion 00187 00188 Starts ADC conversions of the LTC6811 Cpin inputs. 00189 The type of ADC conversion executed can be changed by setting the following parameters: 00190 */ 00191 void LTC681x_adcv(uint8_t MD, //!< ADC Conversion Mode 00192 uint8_t DCP, //!< Controls if Discharge is permitted during conversion 00193 uint8_t CH //!< Sets which Cell channels are converted 00194 ); 00195 00196 /*! Starts cell voltage and GPIO 1&2 conversion 00197 */ 00198 void LTC681x_adcvax( 00199 uint8_t MD, //!< ADC Conversion Mode 00200 uint8_t DCP //!< Controls if Discharge is permitted during conversion 00201 ); 00202 00203 00204 /*! Starts cell voltage self test conversion 00205 */ 00206 void LTC681x_cvst( 00207 uint8_t MD, //!< ADC Conversion Mode 00208 uint8_t ST //!< Self Test Mode 00209 ); 00210 00211 /*! Starts cell voltage and SOC conversion 00212 */ 00213 void LTC681x_adcvsc( 00214 uint8_t MD, //!< ADC Conversion Mode 00215 uint8_t DCP //!< Controls if Discharge is permitted during conversion 00216 ); 00217 /*! Starts cell voltage overlap conversion 00218 */ 00219 void LTC681x_adol( 00220 uint8_t MD, //!< ADC Conversion Mode 00221 uint8_t DCP //!< Discharge permitted during conversion 00222 ); 00223 00224 /*! Start an open wire Conversion 00225 */ 00226 void LTC681x_adow( 00227 uint8_t MD, //!< ADC Conversion Mode 00228 uint8_t PUP //!< Controls if Discharge is permitted during conversion 00229 ); 00230 00231 00232 /*! Start a GPIO and Vref2 Conversion 00233 */ 00234 void LTC681x_adax( 00235 uint8_t MD, //!< ADC Conversion Mode 00236 uint8_t CHG //!< Sets which GPIO channels are converted 00237 ); 00238 00239 /*! Start an GPIO Redundancy test 00240 */ 00241 void LTC681x_adaxd( 00242 uint8_t MD, //!< ADC Conversion Mode 00243 uint8_t CHG //!< Sets which GPIO channels are converted 00244 ); 00245 00246 /*! Start an Auxiliary Register Self Test Conversion 00247 */ 00248 void LTC681x_axst( 00249 uint8_t MD, //!< ADC Conversion Mode 00250 uint8_t ST //!< Sets if self test 1 or 2 is run 00251 ); 00252 00253 00254 00255 /*! Start a Status ADC Conversion 00256 */ 00257 void LTC681x_adstat( 00258 uint8_t MD, //!< ADC Conversion Mode 00259 uint8_t CHST //!< Sets which Stat channels are converted 00260 ); 00261 00262 /*! Start a Status register redundancy test Conversion 00263 */ 00264 void LTC681x_adstatd( 00265 uint8_t MD, //!< ADC Mode 00266 uint8_t CHST //!< Sets which Status channels are converted 00267 ); 00268 00269 00270 /*! Start a Status Register Self Test Conversion 00271 */ 00272 void LTC681x_statst( 00273 uint8_t MD, //!< ADC Conversion Mode 00274 uint8_t ST //!< Sets if self test 1 or 2 is run 00275 ); 00276 00277 void LTC681x_rdcv_reg(uint8_t reg, //!<Determines which cell voltage register is read back 00278 uint8_t total_ic, //!<the number of ICs in the 00279 uint8_t *data //!<An array of the unparsed cell codes 00280 ); 00281 /*! helper function that parses voltage measurement registers 00282 */ 00283 int8_t parse_cells(uint8_t current_ic, 00284 uint8_t cell_reg, 00285 uint8_t cell_data[], 00286 uint16_t *cell_codes, 00287 uint8_t *ic_pec); 00288 00289 /*! Read the raw data from the LTC681x auxiliary register 00290 00291 The function reads a single GPIO voltage register and stores thre read data 00292 in the *data point as a byte array. This function is rarely used outside of 00293 the LTC681x_rdaux() command. 00294 */ 00295 void LTC681x_rdaux_reg( uint8_t reg, //Determines which GPIO voltage register is read back 00296 uint8_t total_ic, //The number of ICs in the system 00297 uint8_t *data //Array of the unparsed auxiliary codes 00298 ); 00299 /*! Read the raw data from the LTC681x stat register 00300 00301 The function reads a single GPIO voltage register and stores thre read data 00302 in the *data point as a byte array. This function is rarely used outside of 00303 the LTC681x_rdstat() command. 00304 */ 00305 void LTC681x_rdstat_reg(uint8_t reg, //Determines which stat register is read back 00306 uint8_t total_ic, //The number of ICs in the system 00307 uint8_t *data //Array of the unparsed stat codes 00308 ); 00309 00310 /*! Clears the LTC681x cell voltage registers 00311 00312 The command clears the cell voltage registers and initializes 00313 all values to 1. The register will read back hexadecimal 0xFF 00314 after the command is sent. 00315 */ 00316 void LTC681x_clrcell(); 00317 /*! Clears the LTC681x Auxiliary registers 00318 00319 The command clears the Auxiliary registers and initializes 00320 all values to 1. The register will read back hexadecimal 0xFF 00321 after the command is sent. 00322 */ 00323 void LTC681x_clraux(); 00324 00325 /*! Clears the LTC681x Stat registers 00326 00327 The command clears the Stat registers and initializes 00328 all values to 1. The register will read back hexadecimal 0xFF 00329 after the command is sent. 00330 */ 00331 void LTC681x_clrstat(); 00332 00333 /*! Clears the LTC681x SCTRL registers 00334 00335 The command clears the SCTRL registers and initializes 00336 all values to 0. The register will read back hexadecimal 0x00 00337 after the command is sent. 00338 */ 00339 void LTC681x_clrsctrl(); 00340 00341 /*! Starts the Mux Decoder diagnostic self test 00342 00343 Running this command will start the Mux Decoder Diagnostic Self Test 00344 This test takes roughly 1mS to complete. The MUXFAIL bit will be updated, 00345 the bit will be set to 1 for a failure and 0 if the test has been passed. 00346 */ 00347 void LTC681x_diagn(); 00348 00349 /*! Reads and parses the LTC681x cell voltage registers. 00350 00351 The function is used to read the cell codes of the LTC6811. 00352 This function will send the requested read commands parse the data 00353 and store the cell voltages in the cell_asic structure. 00354 */ 00355 uint8_t LTC681x_rdcv(uint8_t reg, // Controls which cell voltage register is read back. 00356 uint8_t total_ic, // the number of ICs in the system 00357 cell_asic ic[] // Array of the parsed cell codes 00358 ); 00359 00360 /*! Reads and parses the LTC681x auxiliary registers. 00361 00362 The function is used to read the parsed GPIO codes of the LTC6811. This function will send the requested 00363 read commands parse the data and store the gpio voltages in the cell_asic structure. 00364 */ 00365 int8_t LTC681x_rdaux(uint8_t reg, //Determines which GPIO voltage register is read back. 00366 uint8_t total_ic,//the number of ICs in the system 00367 cell_asic ic[]//!< Measurement Data Structure 00368 ); 00369 00370 /*! Reads and parses the LTC681x stat registers. 00371 00372 The function is used to read the parsed status codes of the LTC6811. This function will send the requested 00373 read commands parse the data and store the status voltages in the cell_asic structure 00374 */ 00375 int8_t LTC681x_rdstat( uint8_t reg, //!<Determines which Stat register is read back. 00376 uint8_t total_ic,//!<the number of ICs in the system 00377 cell_asic ic[]//!< Measurement Data Structure 00378 ); 00379 /*! Write the LTC681x CFGRA 00380 00381 This command will write the configuration registers of the LTC681xs 00382 connected in a daisy chain stack. The configuration is written in descending 00383 order so the last device's configuration is written first. 00384 */ 00385 void LTC681x_wrcfg(uint8_t total_ic, //The number of ICs being written to 00386 cell_asic ic[] //A two dimensional array of the configuration data that will be written 00387 ); 00388 /*! Write the LTC681x CFGRB register 00389 00390 This command will write the configuration registers of the LTC681xs 00391 connected in a daisy chain stack. The configuration is written in descending 00392 order so the last device's configuration is written first. 00393 */ 00394 void LTC681x_wrcfgb(uint8_t total_ic, //The number of ICs being written to 00395 cell_asic ic[] //A two dimensional array of the configuration data that will be written 00396 ); 00397 /*! Reads the LTC681x CFGRA register 00398 */ 00399 int8_t LTC681x_rdcfg(uint8_t total_ic, //Number of ICs in the system 00400 cell_asic ic[] //A two dimensional array that the function stores the read configuration data. 00401 ); 00402 00403 /*! Reads the LTC681x CFGRB register 00404 */ 00405 int8_t LTC681x_rdcfgb(uint8_t total_ic, //Number of ICs in the system 00406 cell_asic ic[] //A two dimensional array that the function stores the read configuration data. 00407 ); 00408 00409 00410 /*! Reads pwm registers of a LTC6811 daisy chain 00411 */ 00412 int8_t LTC681x_rdpwm(uint8_t total_ic, //!<Number of ICs in the system 00413 uint8_t pwmReg, //!< The PWM Register to be written A or B 00414 cell_asic ic[] //!< ASIC Variable 00415 ); 00416 00417 /*! Write the LTC681x PWM register 00418 00419 This command will write the pwm registers of the LTC681x 00420 connected in a daisy chain stack. The pwm is written in descending 00421 order so the last device's pwm is written first. 00422 */ 00423 void LTC681x_wrpwm(uint8_t total_ic, //!< The number of ICs being written to 00424 uint8_t pwmReg, //!< The PWM Register to be written 00425 cell_asic ic[] //!< ASIC Variable 00426 ); 00427 00428 /*! issues a stcomm command and clocks data out of the COMM register */ 00429 void LTC681x_stcomm(); 00430 00431 /*! Reads comm registers of a LTC681x daisy chain 00432 */ 00433 int8_t LTC681x_rdcomm(uint8_t total_ic, //!< Number of ICs in the system 00434 cell_asic ic[] //!< ASIC Variable 00435 ); 00436 00437 /*! Write the LTC681x COMM register 00438 00439 This command will write the comm registers of the LTC681x 00440 connected in a daisy chain stack. The comm is written in descending 00441 order so the last device's configuration is written first. 00442 */ 00443 void LTC681x_wrcomm(uint8_t total_ic, //!< The number of ICs being written to 00444 cell_asic ic[] ///!< ASIC Variable 00445 ); 00446 00447 /*! Selft Test Helper Function*/ 00448 uint16_t LTC681x_st_lookup( 00449 uint8_t MD, //ADC Mode 00450 uint8_t ST //Self Test 00451 ); 00452 00453 /*! Helper Function to clear DCC bits in the CFGR Registers*/ 00454 void clear_discharge(uint8_t total_ic, 00455 cell_asic ic[]); 00456 00457 /*! Helper function that runs the ADC Self Tests*/ 00458 int16_t LTC681x_run_cell_adc_st(uint8_t adc_reg, 00459 uint8_t total_ic, 00460 cell_asic ic[]); 00461 00462 /*! Helper function that runs the ADC Digital Redudancy commands and checks output for errors*/ 00463 int16_t LTC681x_run_adc_redundancy_st(uint8_t adc_mode, 00464 uint8_t adc_reg, 00465 uint8_t total_ic, 00466 cell_asic ic[]); 00467 00468 /*! Helper function that runs the datasheet open wire algorithm*/ 00469 void LTC681x_run_openwire(uint8_t total_ic, 00470 cell_asic ic[]); 00471 00472 /*! Helper Function that runs the ADC Overlap test*/ 00473 uint16_t LTC681x_run_adc_overlap(uint8_t total_ic, 00474 cell_asic ic[]); 00475 /*! Helper Function that counts overall PEC errors and register/IC PEC errors*/ 00476 void LTC681x_check_pec(uint8_t total_ic, 00477 uint8_t reg, 00478 cell_asic ic[]); 00479 00480 /*! Helper Function that resets the PEC error counters */ 00481 void LTC681x_reset_crc_count(uint8_t total_ic, 00482 cell_asic ic[]); 00483 00484 /*! Helper Function to initialize the CFGR data structures*/ 00485 void LTC681x_init_cfg(uint8_t total_ic, 00486 cell_asic ic[]); 00487 00488 /*! Helper function to set appropriate bits in CFGR register based on bit function*/ 00489 void LTC681x_set_cfgr(uint8_t nIC, 00490 cell_asic ic[], 00491 bool refon, 00492 bool adcopt, 00493 bool gpio[5], 00494 bool dcc[12]); 00495 00496 /*! Helper function to turn the refon bit HIGH or LOW*/ 00497 void LTC681x_set_cfgr_refon(uint8_t nIC, 00498 cell_asic ic[], 00499 bool refon); 00500 00501 /*! Helper function to turn the ADCOPT bit HIGH or LOW*/ 00502 void LTC681x_set_cfgr_adcopt(uint8_t nIC, 00503 cell_asic ic[], 00504 bool adcopt); 00505 00506 /*! Helper function to turn the GPIO bits HIGH or LOW*/ 00507 void LTC681x_set_cfgr_gpio(uint8_t nIC, 00508 cell_asic ic[], 00509 bool gpio[]); 00510 00511 /*! Helper function to turn the DCC bits HIGH or LOW*/ 00512 void LTC681x_set_cfgr_dis(uint8_t nIC, 00513 cell_asic ic[], 00514 bool dcc[]); 00515 /*! Helper function to set uv field in CFGRA register*/ 00516 void LTC681x_set_cfgr_uv(uint8_t nIC, 00517 cell_asic ic[], 00518 uint16_t uv); 00519 00520 /*! Helper function to set ov field in CFGRA register*/ 00521 void LTC681x_set_cfgr_ov(uint8_t nIC, 00522 cell_asic ic[], 00523 uint16_t ov); 00524 00525 00526 00527 //#ifdef MBED 00528 //This needs a PROGMEM = when using with a LINDUINO 00529 const uint16_t crc15Table[256] = {0x0,0xc599, 0xceab, 0xb32, 0xd8cf, 0x1d56, 0x1664, 0xd3fd, 0xf407, 0x319e, 0x3aac, //!<precomputed CRC15 Table 00530 0xff35, 0x2cc8, 0xe951, 0xe263, 0x27fa, 0xad97, 0x680e, 0x633c, 0xa6a5, 0x7558, 0xb0c1, 00531 0xbbf3, 0x7e6a, 0x5990, 0x9c09, 0x973b, 0x52a2, 0x815f, 0x44c6, 0x4ff4, 0x8a6d, 0x5b2e, 00532 0x9eb7, 0x9585, 0x501c, 0x83e1, 0x4678, 0x4d4a, 0x88d3, 0xaf29, 0x6ab0, 0x6182, 0xa41b, 00533 0x77e6, 0xb27f, 0xb94d, 0x7cd4, 0xf6b9, 0x3320, 0x3812, 0xfd8b, 0x2e76, 0xebef, 0xe0dd, 00534 0x2544, 0x2be, 0xc727, 0xcc15, 0x98c, 0xda71, 0x1fe8, 0x14da, 0xd143, 0xf3c5, 0x365c, 00535 0x3d6e, 0xf8f7,0x2b0a, 0xee93, 0xe5a1, 0x2038, 0x7c2, 0xc25b, 0xc969, 0xcf0, 0xdf0d, 00536 0x1a94, 0x11a6, 0xd43f, 0x5e52, 0x9bcb, 0x90f9, 0x5560, 0x869d, 0x4304, 0x4836, 0x8daf, 00537 0xaa55, 0x6fcc, 0x64fe, 0xa167, 0x729a, 0xb703, 0xbc31, 0x79a8, 0xa8eb, 0x6d72, 0x6640, 00538 0xa3d9, 0x7024, 0xb5bd, 0xbe8f, 0x7b16, 0x5cec, 0x9975, 0x9247, 0x57de, 0x8423, 0x41ba, 00539 0x4a88, 0x8f11, 0x57c, 0xc0e5, 0xcbd7, 0xe4e, 0xddb3, 0x182a, 0x1318, 0xd681, 0xf17b, 00540 0x34e2, 0x3fd0, 0xfa49, 0x29b4, 0xec2d, 0xe71f, 0x2286, 0xa213, 0x678a, 0x6cb8, 0xa921, 00541 0x7adc, 0xbf45, 0xb477, 0x71ee, 0x5614, 0x938d, 0x98bf, 0x5d26, 0x8edb, 0x4b42, 0x4070, 00542 0x85e9, 0xf84, 0xca1d, 0xc12f, 0x4b6, 0xd74b, 0x12d2, 0x19e0, 0xdc79, 0xfb83, 0x3e1a, 0x3528, 00543 0xf0b1, 0x234c, 0xe6d5, 0xede7, 0x287e, 0xf93d, 0x3ca4, 0x3796, 0xf20f, 0x21f2, 0xe46b, 0xef59, 00544 0x2ac0, 0xd3a, 0xc8a3, 0xc391, 0x608, 0xd5f5, 0x106c, 0x1b5e, 0xdec7, 0x54aa, 0x9133, 0x9a01, 00545 0x5f98, 0x8c65, 0x49fc, 0x42ce, 0x8757, 0xa0ad, 0x6534, 0x6e06, 0xab9f, 0x7862, 0xbdfb, 0xb6c9, 00546 0x7350, 0x51d6, 0x944f, 0x9f7d, 0x5ae4, 0x8919, 0x4c80, 0x47b2, 0x822b, 0xa5d1, 0x6048, 0x6b7a, 00547 0xaee3, 0x7d1e, 0xb887, 0xb3b5, 0x762c, 0xfc41, 0x39d8, 0x32ea, 0xf773, 0x248e, 0xe117, 0xea25, 00548 0x2fbc, 0x846, 0xcddf, 0xc6ed, 0x374, 0xd089, 0x1510, 0x1e22, 0xdbbb, 0xaf8, 0xcf61, 0xc453, 00549 0x1ca, 0xd237, 0x17ae, 0x1c9c, 0xd905, 0xfeff, 0x3b66, 0x3054, 0xf5cd, 0x2630, 0xe3a9, 0xe89b, 00550 0x2d02, 0xa76f, 0x62f6, 0x69c4, 0xac5d, 0x7fa0, 0xba39, 0xb10b, 0x7492, 0x5368, 0x96f1, 0x9dc3, 00551 0x585a, 0x8ba7, 0x4e3e, 0x450c, 0x8095 00552 }; 00553 00554 //#else 00555 //const uint16_t crc15Table[256] PROGMEM = {0x0,0xc599, 0xceab, 0xb32, 0xd8cf, 0x1d56, 0x1664, 0xd3fd, 0xf407, 0x319e, 0x3aac, //!<precomputed CRC15 Table 00556 // 0xff35, 0x2cc8, 0xe951, 0xe263, 0x27fa, 0xad97, 0x680e, 0x633c, 0xa6a5, 0x7558, 0xb0c1, 00557 // 0xbbf3, 0x7e6a, 0x5990, 0x9c09, 0x973b, 0x52a2, 0x815f, 0x44c6, 0x4ff4, 0x8a6d, 0x5b2e, 00558 // 0x9eb7, 0x9585, 0x501c, 0x83e1, 0x4678, 0x4d4a, 0x88d3, 0xaf29, 0x6ab0, 0x6182, 0xa41b, 00559 // 0x77e6, 0xb27f, 0xb94d, 0x7cd4, 0xf6b9, 0x3320, 0x3812, 0xfd8b, 0x2e76, 0xebef, 0xe0dd, 00560 // 0x2544, 0x2be, 0xc727, 0xcc15, 0x98c, 0xda71, 0x1fe8, 0x14da, 0xd143, 0xf3c5, 0x365c, 00561 // 0x3d6e, 0xf8f7,0x2b0a, 0xee93, 0xe5a1, 0x2038, 0x7c2, 0xc25b, 0xc969, 0xcf0, 0xdf0d, 00562 // 0x1a94, 0x11a6, 0xd43f, 0x5e52, 0x9bcb, 0x90f9, 0x5560, 0x869d, 0x4304, 0x4836, 0x8daf, 00563 // 0xaa55, 0x6fcc, 0x64fe, 0xa167, 0x729a, 0xb703, 0xbc31, 0x79a8, 0xa8eb, 0x6d72, 0x6640, 00564 // 0xa3d9, 0x7024, 0xb5bd, 0xbe8f, 0x7b16, 0x5cec, 0x9975, 0x9247, 0x57de, 0x8423, 0x41ba, 00565 // 0x4a88, 0x8f11, 0x57c, 0xc0e5, 0xcbd7, 0xe4e, 0xddb3, 0x182a, 0x1318, 0xd681, 0xf17b, 00566 // 0x34e2, 0x3fd0, 0xfa49, 0x29b4, 0xec2d, 0xe71f, 0x2286, 0xa213, 0x678a, 0x6cb8, 0xa921, 00567 // 0x7adc, 0xbf45, 0xb477, 0x71ee, 0x5614, 0x938d, 0x98bf, 0x5d26, 0x8edb, 0x4b42, 0x4070, 00568 // 0x85e9, 0xf84, 0xca1d, 0xc12f, 0x4b6, 0xd74b, 0x12d2, 0x19e0, 0xdc79, 0xfb83, 0x3e1a, 0x3528, 00569 // 0xf0b1, 0x234c, 0xe6d5, 0xede7, 0x287e, 0xf93d, 0x3ca4, 0x3796, 0xf20f, 0x21f2, 0xe46b, 0xef59, 00570 // 0x2ac0, 0xd3a, 0xc8a3, 0xc391, 0x608, 0xd5f5, 0x106c, 0x1b5e, 0xdec7, 0x54aa, 0x9133, 0x9a01, 00571 // 0x5f98, 0x8c65, 0x49fc, 0x42ce, 0x8757, 0xa0ad, 0x6534, 0x6e06, 0xab9f, 0x7862, 0xbdfb, 0xb6c9, 00572 // 0x7350, 0x51d6, 0x944f, 0x9f7d, 0x5ae4, 0x8919, 0x4c80, 0x47b2, 0x822b, 0xa5d1, 0x6048, 0x6b7a, 00573 // 0xaee3, 0x7d1e, 0xb887, 0xb3b5, 0x762c, 0xfc41, 0x39d8, 0x32ea, 0xf773, 0x248e, 0xe117, 0xea25, 00574 // 0x2fbc, 0x846, 0xcddf, 0xc6ed, 0x374, 0xd089, 0x1510, 0x1e22, 0xdbbb, 0xaf8, 0xcf61, 0xc453, 00575 // 0x1ca, 0xd237, 0x17ae, 0x1c9c, 0xd905, 0xfeff, 0x3b66, 0x3054, 0xf5cd, 0x2630, 0xe3a9, 0xe89b, 00576 // 0x2d02, 0xa76f, 0x62f6, 0x69c4, 0xac5d, 0x7fa0, 0xba39, 0xb10b, 0x7492, 0x5368, 0x96f1, 0x9dc3, 00577 // 0x585a, 0x8ba7, 0x4e3e, 0x450c, 0x8095 00578 // }; 00579 //#endif 00580 #endif
Generated on Tue Jul 12 2022 21:25:39 by
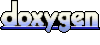