
各ピンへのread/writeを提供するサーバサンプル
Dependencies: NySNICInterface mbed-rtos mbed
Fork of RESTServerSample2 by
RequestHandler.cpp
00001 #include "mbed.h" 00002 #include "RequestHandler.h" 00003 #include "RPCObject.h" 00004 #include "HTTPServer.h" 00005 00006 00007 int GetRequestHandler::handle(RPCObject& cmd, char* reply) 00008 { 00009 int value; 00010 std::map<PinName, RPCClass*>::iterator itor; 00011 00012 printf("handling GET request.\r\n"); 00013 itor = cmd.pinObjects.find(cmd.get_pin_name()); 00014 if(itor == cmd.pinObjects.end()){ 00015 printf("The pin is not created.\r\n"); 00016 return HTTP_404_NOTFOUND; 00017 } 00018 value = itor->second->read(); 00019 00020 reply[0] = '0' + value; 00021 reply[1] = '\0'; 00022 00023 return HTTP_200_OK; 00024 } 00025 00026 00027 int PostRequestHandler::handle(RPCObject& cmd, char* reply) 00028 { 00029 int value = cmd.get_value(); 00030 std::map<PinName, RPCClass*>::iterator itor; 00031 00032 printf("handling POST request.\r\n"); 00033 switch(value){ 00034 case 0: 00035 case 1: 00036 //update 00037 printf("now updating the object to %d.\r\n", value); 00038 itor = cmd.pinObjects.find(cmd.get_pin_name()); 00039 if(itor == cmd.pinObjects.end()){ 00040 printf("The pin is not created.\r\n"); 00041 return HTTP_404_NOTFOUND; 00042 } 00043 itor->second->write(value); 00044 break; 00045 case -1: 00046 //create 00047 printf("now createing the object.\r\n"); 00048 if(!cmd.create_pin_object(reply)){ 00049 return -1; 00050 } 00051 break; 00052 case -2: 00053 // delete 00054 itor = cmd.pinObjects.find(cmd.get_pin_name()); 00055 if(itor == cmd.pinObjects.end()){ 00056 printf("The pin is not created.\r\n"); 00057 return HTTP_404_NOTFOUND; 00058 } 00059 delete itor->second; 00060 cmd.pinObjects.erase(cmd.pinObjects.find(cmd.get_pin_name())); 00061 break; 00062 default: 00063 return -1; 00064 } 00065 00066 return HTTP_200_OK; 00067 } 00068 00069 00070 int DeleteRequestHandler::handle(RPCObject& cmd, char* reply) 00071 { 00072 std::map<PinName, RPCClass*>::iterator itor; 00073 00074 printf("handling DELETE request.\r\n"); 00075 itor = cmd.pinObjects.find(cmd.get_pin_name()); 00076 if(itor == cmd.pinObjects.end()){ 00077 printf("The pin is not created.\r\n"); 00078 return HTTP_404_NOTFOUND; 00079 } 00080 delete itor->second; 00081 cmd.pinObjects.erase(cmd.pinObjects.find(cmd.get_pin_name())); 00082 00083 return HTTP_200_OK; 00084 } 00085 00086
Generated on Tue Jul 12 2022 18:49:20 by
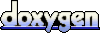