
Websocket_Sample for MurataTypeYD
Embed:
(wiki syntax)
Show/hide line numbers
SNIC_WifiInterface.cpp
00001 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00002 * muRata, SWITCH SCIENCE Wi-FI module TypeYD-SNIC UART. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #include "SNIC_WifiInterface.h" 00020 #include "SNIC_UartMsgUtil.h" 00021 00022 #define UART_CONNECT_BUF_SIZE 512 00023 unsigned char gCONNECT_BUF[UART_CONNECT_BUF_SIZE]; 00024 static char ip_addr[17] = "\0"; 00025 00026 C_SNIC_WifiInterface::C_SNIC_WifiInterface( PinName tx, PinName rx, PinName cts, PinName rts, PinName reset, PinName alarm, int baud) 00027 { 00028 mUART_tx = tx; 00029 mUART_rx = rx; 00030 mUART_cts = cts; 00031 mUART_rts = rts;; 00032 mUART_baud = baud; 00033 mModuleReset = reset; 00034 } 00035 00036 C_SNIC_WifiInterface::~C_SNIC_WifiInterface() 00037 { 00038 } 00039 00040 int C_SNIC_WifiInterface::init() 00041 { 00042 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00043 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00044 00045 /* Initialize UART */ 00046 snic_core_p->initUart( mUART_tx, mUART_rx, mUART_baud ); 00047 00048 /* Module reset */ 00049 snic_core_p->resetModule( mModuleReset ); 00050 00051 wait(1); 00052 /* Initialize SNIC API */ 00053 // Get buffer for response payload from MemoryPool 00054 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00055 if( payload_buf_p == NULL ) 00056 { 00057 DEBUG_PRINT("snic_init payload_buf_p NULL\r\n"); 00058 return -1; 00059 } 00060 00061 C_SNIC_Core::tagSNIC_INIT_REQ_T req; 00062 // Make request 00063 req.cmd_sid = UART_CMD_SID_SNIC_INIT_REQ; 00064 req.seq = mUartRequestSeq++; 00065 req.buf_size[0] = 0x08; 00066 req.buf_size[1] = 0x00; 00067 00068 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00069 unsigned int command_len; 00070 // Preparation of command 00071 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00072 , sizeof(C_SNIC_Core::tagSNIC_INIT_REQ_T), payload_buf_p->buf, command_array_p ); 00073 00074 // Send uart command request 00075 snic_core_p->sendUart( command_len, command_array_p ); 00076 00077 int ret; 00078 // Wait UART response 00079 ret = uartCmdMgr_p->wait(); 00080 if( ret != 0 ) 00081 { 00082 DEBUG_PRINT( "snic_init failed\r\n" ); 00083 snic_core_p->freeCmdBuf( payload_buf_p ); 00084 return -1; 00085 } 00086 00087 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00088 { 00089 DEBUG_PRINT("snic_init status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00090 snic_core_p->freeCmdBuf( payload_buf_p ); 00091 return -1; 00092 } 00093 snic_core_p->freeCmdBuf( payload_buf_p ); 00094 00095 return ret; 00096 } 00097 00098 int C_SNIC_WifiInterface::getFWVersion( unsigned char *version_p ) 00099 { 00100 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00101 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00102 00103 // Get buffer for response payload from MemoryPool 00104 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00105 if( payload_buf_p == NULL ) 00106 { 00107 DEBUG_PRINT("getFWVersion payload_buf_p NULL\r\n"); 00108 return -1; 00109 } 00110 00111 C_SNIC_Core::tagGEN_FW_VER_GET_REQ_T req; 00112 // Make request 00113 req.cmd_sid = UART_CMD_SID_GEN_FW_VER_GET_REQ; 00114 req.seq = mUartRequestSeq++; 00115 00116 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00117 unsigned int command_len; 00118 // Preparation of command 00119 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_GEN, req.cmd_sid, (unsigned char *)&req 00120 , sizeof(C_SNIC_Core::tagGEN_FW_VER_GET_REQ_T), payload_buf_p->buf, command_array_p ); 00121 00122 int ret; 00123 00124 // Send uart command request 00125 snic_core_p->sendUart( command_len, command_array_p ); 00126 00127 // Wait UART response 00128 ret = uartCmdMgr_p->wait(); 00129 if( ret != 0 ) 00130 { 00131 DEBUG_PRINT( "getFWversion failed\r\n" ); 00132 snic_core_p->freeCmdBuf( payload_buf_p ); 00133 return -1; 00134 } 00135 00136 if( uartCmdMgr_p->getCommandStatus() == 0 ) 00137 { 00138 unsigned char version_len = payload_buf_p->buf[3]; 00139 memcpy( version_p, &payload_buf_p->buf[4], version_len ); 00140 } 00141 snic_core_p->freeCmdBuf( payload_buf_p ); 00142 return 0; 00143 } 00144 00145 int C_SNIC_WifiInterface::connect(const char *ssid_p, unsigned char ssid_len, E_SECURITY sec_type 00146 , const char *sec_key_p, unsigned char sec_key_len) 00147 { 00148 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00149 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00150 00151 // Parameter check(SSID) 00152 if( (ssid_p == NULL) || (ssid_len == 0) ) 00153 { 00154 DEBUG_PRINT( "connect failed [ parameter NG:SSID ]\r\n" ); 00155 return -1; 00156 } 00157 00158 // Parameter check(Security key) 00159 if( (sec_type != e_SEC_OPEN) && ( (sec_key_len == 0) || (sec_key_p == NULL) ) ) 00160 { 00161 DEBUG_PRINT( "connect failed [ parameter NG:Security key ]\r\n" ); 00162 return -1; 00163 } 00164 00165 // Get buffer for response payload from MemoryPool 00166 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00167 if( payload_buf_p == NULL ) 00168 { 00169 DEBUG_PRINT("connect payload_buf_p NULL\r\n"); 00170 return -1; 00171 } 00172 00173 unsigned char *buf = &gCONNECT_BUF[0]; 00174 unsigned int buf_len = 0; 00175 unsigned int command_len; 00176 00177 memset( buf, 0, UART_CONNECT_BUF_SIZE ); 00178 // Make request 00179 buf[0] = UART_CMD_SID_WIFI_JOIN_REQ; 00180 buf_len++; 00181 buf[1] = mUartRequestSeq++; 00182 buf_len++; 00183 // SSID 00184 memcpy( &buf[2], ssid_p, ssid_len ); 00185 buf_len += ssid_len; 00186 buf_len++; 00187 00188 // Security mode 00189 buf[ buf_len ] = (unsigned char)sec_type; 00190 buf_len++; 00191 00192 // Security key 00193 if( sec_type != e_SEC_OPEN ) 00194 { 00195 buf[ buf_len ] = sec_key_len; 00196 buf_len++; 00197 if( sec_key_len > 0 ) 00198 { 00199 memcpy( &buf[buf_len], sec_key_p, sec_key_len ); 00200 buf_len += sec_key_len; 00201 } 00202 } 00203 00204 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00205 // Preparation of command 00206 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, UART_CMD_SID_WIFI_JOIN_REQ, buf 00207 , buf_len, payload_buf_p->buf, command_array_p ); 00208 00209 // Send uart command request 00210 snic_core_p->sendUart( command_len, command_array_p ); 00211 00212 int ret; 00213 // Wait UART response 00214 ret = uartCmdMgr_p->wait(); 00215 if(uartCmdMgr_p->getCommandStatus() != UART_CMD_RES_WIFI_ERR_ALREADY_JOINED) 00216 { 00217 DEBUG_PRINT( "Already connected\r\n" ); 00218 } 00219 else 00220 { 00221 if( ret != 0 ) 00222 { 00223 DEBUG_PRINT( "join failed\r\n" ); 00224 snic_core_p->freeCmdBuf( payload_buf_p ); 00225 return -1; 00226 } 00227 } 00228 00229 if(uartCmdMgr_p->getCommandStatus() != 0) 00230 { 00231 DEBUG_PRINT("join status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00232 snic_core_p->freeCmdBuf( payload_buf_p ); 00233 return -1; 00234 } 00235 snic_core_p->freeCmdBuf( payload_buf_p ); 00236 00237 return ret; 00238 } 00239 00240 int C_SNIC_WifiInterface::disconnect() 00241 { 00242 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00243 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00244 00245 // Get buffer for response payload from MemoryPool 00246 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00247 if( payload_buf_p == NULL ) 00248 { 00249 DEBUG_PRINT("disconnect payload_buf_p NULL\r\n"); 00250 return -1; 00251 } 00252 00253 C_SNIC_Core::tagWIFI_DISCONNECT_REQ_T req; 00254 // Make request 00255 req.cmd_sid = UART_CMD_SID_WIFI_DISCONNECT_REQ; 00256 req.seq = mUartRequestSeq++; 00257 00258 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00259 unsigned int command_len; 00260 // Preparation of command 00261 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00262 , sizeof(C_SNIC_Core::tagWIFI_DISCONNECT_REQ_T), payload_buf_p->buf, command_array_p ); 00263 00264 // Send uart command request 00265 snic_core_p->sendUart( command_len, command_array_p ); 00266 00267 int ret; 00268 // Wait UART response 00269 ret = uartCmdMgr_p->wait(); 00270 if( ret != 0 ) 00271 { 00272 DEBUG_PRINT( "disconnect failed\r\n" ); 00273 snic_core_p->freeCmdBuf( payload_buf_p ); 00274 return -1; 00275 } 00276 00277 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00278 { 00279 DEBUG_PRINT("disconnect status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00280 ret = -1; 00281 } 00282 snic_core_p->freeCmdBuf( payload_buf_p ); 00283 return ret; 00284 } 00285 00286 int C_SNIC_WifiInterface::scan( const char *ssid_p, unsigned char *bssid_p 00287 , void (*result_handler_p)(tagSCAN_RESULT_T *scan_result) ) 00288 { 00289 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00290 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00291 00292 // Get buffer for response payload from MemoryPool 00293 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00294 if( payload_buf_p == NULL ) 00295 { 00296 DEBUG_PRINT("scan payload_buf_p NULL\r\n"); 00297 return -1; 00298 } 00299 00300 C_SNIC_Core::tagWIFI_SCAN_REQ_T req; 00301 unsigned int buf_len = 0; 00302 00303 memset( &req, 0, sizeof(C_SNIC_Core::tagWIFI_SCAN_REQ_T) ); 00304 // Make request 00305 req.cmd_sid = UART_CMD_SID_WIFI_SCAN_REQ; 00306 buf_len++; 00307 req.seq = mUartRequestSeq++; 00308 buf_len++; 00309 00310 // Set scan type(Active scan) 00311 req.scan_type = 0; 00312 buf_len++; 00313 // Set bss type(any) 00314 req.bss_type = 2; 00315 buf_len++; 00316 // Set BSSID 00317 if( bssid_p != NULL ) 00318 { 00319 memcpy( req.bssid, bssid_p, BSSID_MAC_LENTH ); 00320 } 00321 buf_len += BSSID_MAC_LENTH; 00322 // Set channel list(0) 00323 req.chan_list = 0; 00324 buf_len++; 00325 //Set SSID 00326 if( ssid_p != NULL ) 00327 { 00328 strcpy( (char *)req.ssid, ssid_p ); 00329 buf_len += strlen(ssid_p); 00330 } 00331 buf_len++; 00332 00333 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00334 unsigned int command_len; 00335 // Preparation of command 00336 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00337 , buf_len, payload_buf_p->buf, command_array_p ); 00338 00339 // Set scan result callback 00340 uartCmdMgr_p->setScanResultHandler( result_handler_p ); 00341 00342 // Send uart command request 00343 snic_core_p->sendUart( command_len, command_array_p ); 00344 00345 int ret; 00346 // Wait UART response 00347 ret = uartCmdMgr_p->wait(); 00348 DEBUG_PRINT( "scan wait:%d\r\n", ret ); 00349 if( ret != 0 ) 00350 { 00351 DEBUG_PRINT( "scan failed\r\n" ); 00352 snic_core_p->freeCmdBuf( payload_buf_p ); 00353 return -1; 00354 } 00355 00356 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00357 { 00358 DEBUG_PRINT("scan status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00359 snic_core_p->freeCmdBuf( payload_buf_p ); 00360 return -1; 00361 } 00362 00363 snic_core_p->freeCmdBuf( payload_buf_p ); 00364 00365 return ret; 00366 } 00367 00368 int C_SNIC_WifiInterface::wifi_on( const char *country_p ) 00369 { 00370 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00371 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00372 00373 // Parameter check 00374 if( country_p == NULL ) 00375 { 00376 DEBUG_PRINT("wifi_on parameter error\r\n"); 00377 return -1; 00378 } 00379 00380 // Get buffer for response payload from MemoryPool 00381 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00382 if( payload_buf_p == NULL ) 00383 { 00384 DEBUG_PRINT("wifi_on payload_buf_p NULL\r\n"); 00385 return -1; 00386 } 00387 00388 C_SNIC_Core::tagWIFI_ON_REQ_T req; 00389 // Make request 00390 req.cmd_sid = UART_CMD_SID_WIFI_ON_REQ; 00391 req.seq = mUartRequestSeq++; 00392 memcpy( req.country, country_p, COUNTRYC_CODE_LENTH ); 00393 00394 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00395 unsigned int command_len; 00396 // Preparation of command 00397 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00398 , sizeof(C_SNIC_Core::tagWIFI_ON_REQ_T), payload_buf_p->buf, command_array_p ); 00399 00400 // Send uart command request 00401 snic_core_p->sendUart( command_len, command_array_p ); 00402 00403 int ret; 00404 // Wait UART response 00405 ret = uartCmdMgr_p->wait(); 00406 if( ret != 0 ) 00407 { 00408 DEBUG_PRINT( "wifi_on failed\r\n" ); 00409 snic_core_p->freeCmdBuf( payload_buf_p ); 00410 return -1; 00411 } 00412 00413 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00414 { 00415 DEBUG_PRINT("wifi_on status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00416 snic_core_p->freeCmdBuf( payload_buf_p ); 00417 return -1; 00418 } 00419 snic_core_p->freeCmdBuf( payload_buf_p ); 00420 00421 return ret; 00422 } 00423 00424 int C_SNIC_WifiInterface::wifi_off() 00425 { 00426 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00427 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00428 00429 // Get buffer for response payload from MemoryPool 00430 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00431 if( payload_buf_p == NULL ) 00432 { 00433 DEBUG_PRINT("wifi_off payload_buf_p NULL\r\n"); 00434 return -1; 00435 } 00436 00437 C_SNIC_Core::tagWIFI_OFF_REQ_T req; 00438 // Make request 00439 req.cmd_sid = UART_CMD_SID_WIFI_OFF_REQ; 00440 req.seq = mUartRequestSeq++; 00441 00442 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00443 unsigned int command_len; 00444 // Preparation of command 00445 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00446 , sizeof(C_SNIC_Core::tagWIFI_OFF_REQ_T), payload_buf_p->buf, command_array_p ); 00447 00448 // Send uart command request 00449 snic_core_p->sendUart( command_len, command_array_p ); 00450 00451 int ret; 00452 // Wait UART response 00453 ret = uartCmdMgr_p->wait(); 00454 if( ret != 0 ) 00455 { 00456 DEBUG_PRINT( "wifi_off failed\r\n" ); 00457 snic_core_p->freeCmdBuf( payload_buf_p ); 00458 return -1; 00459 } 00460 00461 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00462 { 00463 DEBUG_PRINT("wifi_off status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00464 snic_core_p->freeCmdBuf( payload_buf_p ); 00465 return -1; 00466 } 00467 snic_core_p->freeCmdBuf( payload_buf_p ); 00468 00469 return ret; 00470 } 00471 00472 int C_SNIC_WifiInterface::getRssi( signed char *rssi_p ) 00473 { 00474 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00475 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00476 if( rssi_p == NULL ) 00477 { 00478 DEBUG_PRINT("getRssi parameter error\r\n"); 00479 return -1; 00480 } 00481 00482 // Get buffer for response payload from MemoryPool 00483 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00484 if( payload_buf_p == NULL ) 00485 { 00486 DEBUG_PRINT("getRssi payload_buf_p NULL\r\n"); 00487 return -1; 00488 } 00489 00490 C_SNIC_Core::tagWIFI_GET_STA_RSSI_REQ_T req; 00491 00492 // Make request 00493 req.cmd_sid = UART_CMD_SID_WIFI_GET_STA_RSSI_REQ; 00494 req.seq = mUartRequestSeq++; 00495 00496 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00497 unsigned int command_len; 00498 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00499 , sizeof(C_SNIC_Core::tagWIFI_GET_STA_RSSI_REQ_T), payload_buf_p->buf, command_array_p ); 00500 00501 int ret; 00502 // Send uart command request 00503 snic_core_p->sendUart( command_len, command_array_p ); 00504 00505 // Wait UART response 00506 ret = uartCmdMgr_p->wait(); 00507 if( ret != 0 ) 00508 { 00509 DEBUG_PRINT( "getRssi failed\r\n" ); 00510 snic_core_p->freeCmdBuf( payload_buf_p ); 00511 return -1; 00512 } 00513 00514 *rssi_p = (signed char)payload_buf_p->buf[2]; 00515 00516 snic_core_p->freeCmdBuf( payload_buf_p ); 00517 return 0; 00518 } 00519 00520 int C_SNIC_WifiInterface::getWifiStatus( tagWIFI_STATUS_T *status_p) 00521 { 00522 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00523 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00524 00525 if( status_p == NULL ) 00526 { 00527 DEBUG_PRINT("getWifiStatus parameter error\r\n"); 00528 return -1; 00529 } 00530 00531 // Get buffer for response payload from MemoryPool 00532 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00533 if( payload_buf_p == NULL ) 00534 { 00535 DEBUG_PRINT("getWifiStatus payload_buf_p NULL\r\n"); 00536 return -1; 00537 } 00538 00539 C_SNIC_Core::tagWIFI_GET_STATUS_REQ_T req; 00540 // Make request 00541 req.cmd_sid = UART_CMD_SID_WIFI_GET_STATUS_REQ; 00542 req.seq = mUartRequestSeq++; 00543 req.interface = 0; 00544 00545 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00546 unsigned int command_len; 00547 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00548 , sizeof(C_SNIC_Core::tagWIFI_GET_STATUS_REQ_T), payload_buf_p->buf, command_array_p ); 00549 00550 // Send uart command request 00551 snic_core_p->sendUart( command_len, command_array_p ); 00552 00553 int ret; 00554 // Wait UART response 00555 ret = uartCmdMgr_p->wait(); 00556 if( ret != 0 ) 00557 { 00558 DEBUG_PRINT( "getWifiStatus failed\r\n" ); 00559 snic_core_p->freeCmdBuf( payload_buf_p ); 00560 return -1; 00561 } 00562 00563 // set status 00564 status_p->status = (E_WIFI_STATUS)payload_buf_p->buf[2]; 00565 00566 // set Mac address 00567 if( status_p->status != e_STATUS_OFF ) 00568 { 00569 memcpy( status_p->mac_address, &payload_buf_p->buf[3], BSSID_MAC_LENTH ); 00570 } 00571 00572 // set SSID 00573 if( ( status_p->status == e_STA_JOINED ) || ( status_p->status == e_AP_STARTED ) ) 00574 { 00575 memcpy( status_p->ssid, &payload_buf_p->buf[9], strlen( (char *)&payload_buf_p->buf[9]) ); 00576 } 00577 00578 snic_core_p->freeCmdBuf( payload_buf_p ); 00579 return 0; 00580 } 00581 00582 int C_SNIC_WifiInterface::setIPConfig( bool is_DHCP 00583 , const char *ip_p, const char *mask_p, const char *gateway_p ) 00584 { 00585 // Parameter check 00586 if( is_DHCP == false ) 00587 { 00588 if( (ip_p == NULL) || (mask_p == NULL) ||(gateway_p == NULL) ) 00589 { 00590 DEBUG_PRINT("setIPConfig parameter error\r\n"); 00591 return -1; 00592 } 00593 } 00594 00595 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00596 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00597 00598 // Get buffer for response payload from MemoryPool 00599 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00600 if( payload_buf_p == NULL ) 00601 { 00602 DEBUG_PRINT("setIPConfig payload_buf_p NULL\r\n"); 00603 return -1; 00604 } 00605 00606 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00607 unsigned int command_len; 00608 if( is_DHCP == true ) 00609 { 00610 C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_DHCP_T req; 00611 // Make request 00612 req.cmd_sid = UART_CMD_SID_SNIC_IP_CONFIG_REQ; 00613 req.seq = mUartRequestSeq++; 00614 req.interface = 0; 00615 req.dhcp = 1; 00616 00617 // Preparation of command 00618 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00619 , sizeof(C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_DHCP_T), payload_buf_p->buf, command_array_p ); 00620 } 00621 else 00622 { 00623 C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_STATIC_T req; 00624 // Make request 00625 req.cmd_sid = UART_CMD_SID_SNIC_IP_CONFIG_REQ; 00626 req.seq = mUartRequestSeq++; 00627 req.interface = 0; 00628 req.dhcp = 0; 00629 00630 // Set paramter of address 00631 int addr_temp; 00632 addr_temp = C_SNIC_UartMsgUtil::addrToInteger( ip_p ); 00633 C_SNIC_UartMsgUtil::convertIntToByteAdday( addr_temp, (char *)req.ip_addr ); 00634 addr_temp = C_SNIC_UartMsgUtil::addrToInteger( mask_p ); 00635 C_SNIC_UartMsgUtil::convertIntToByteAdday( addr_temp, (char *)req.netmask ); 00636 addr_temp = C_SNIC_UartMsgUtil::addrToInteger( gateway_p ); 00637 C_SNIC_UartMsgUtil::convertIntToByteAdday( addr_temp, (char *)req.gateway ); 00638 00639 // Preparation of command 00640 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00641 , sizeof(C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_STATIC_T), payload_buf_p->buf, command_array_p ); 00642 } 00643 // Send uart command request 00644 snic_core_p->sendUart( command_len, command_array_p ); 00645 00646 int ret; 00647 // Wait UART response 00648 ret = uartCmdMgr_p->wait(); 00649 if( ret != 0 ) 00650 { 00651 DEBUG_PRINT( "setIPConfig failed\r\n" ); 00652 snic_core_p->freeCmdBuf( payload_buf_p ); 00653 return -1; 00654 } 00655 00656 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00657 { 00658 DEBUG_PRINT("setIPConfig status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00659 snic_core_p->freeCmdBuf( payload_buf_p ); 00660 return -1; 00661 } 00662 00663 snic_core_p->freeCmdBuf( payload_buf_p ); 00664 return ret; 00665 } 00666 00667 char* C_SNIC_WifiInterface::getIPAddress() { 00668 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00669 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00670 00671 snic_core_p->lockAPI(); 00672 // Get local ip address. 00673 // Get buffer for response payload from MemoryPool 00674 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00675 if( payload_buf_p == NULL ) 00676 { 00677 DEBUG_PRINT("getIPAddress payload_buf_p NULL\r\n"); 00678 snic_core_p->unlockAPI(); 00679 return 0; 00680 } 00681 00682 C_SNIC_Core::tagSNIC_GET_DHCP_INFO_REQ_T req; 00683 // Make request 00684 req.cmd_sid = UART_CMD_SID_SNIC_GET_DHCP_INFO_REQ; 00685 req.seq = mUartRequestSeq++; 00686 req.interface = 0; 00687 00688 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00689 unsigned int command_len; 00690 // Preparation of command 00691 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00692 , sizeof(C_SNIC_Core::tagSNIC_GET_DHCP_INFO_REQ_T), payload_buf_p->buf, command_array_p ); 00693 // Send uart command request 00694 snic_core_p->sendUart( command_len, command_array_p ); 00695 // Wait UART response 00696 int ret = uartCmdMgr_p->wait(); 00697 if( ret != 0 ) 00698 { 00699 DEBUG_PRINT( "getIPAddress failed\r\n" ); 00700 snic_core_p->freeCmdBuf( payload_buf_p ); 00701 snic_core_p->unlockAPI(); 00702 return 0; 00703 } 00704 00705 if( uartCmdMgr_p->getCommandStatus() != UART_CMD_RES_SNIC_SUCCESS ) 00706 { 00707 snic_core_p->freeCmdBuf( payload_buf_p ); 00708 snic_core_p->unlockAPI(); 00709 return 0; 00710 } 00711 00712 snic_core_p->freeCmdBuf( payload_buf_p ); 00713 snic_core_p->unlockAPI(); 00714 00715 sprintf(ip_addr, "%d.%d.%d.%d\0", payload_buf_p->buf[9], payload_buf_p->buf[10], payload_buf_p->buf[11], payload_buf_p->buf[12]); 00716 00717 return ip_addr; 00718 } 00719
Generated on Thu Jul 14 2022 06:29:01 by
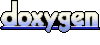