
WallbotBLE default code
Dependencies: mbed
Fork of BLE_WallbotBLE_Challenge by
ble_gls.h
00001 /* Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 */ 00011 00012 /** @file 00013 * 00014 * @defgroup ble_sdk_srv_gls Glucose Service 00015 * @{ 00016 * @ingroup ble_sdk_srv 00017 * @brief Glucose Service module. 00018 * 00019 * @details This module implements the Glucose Service. 00020 * 00021 * @note The application must propagate BLE stack events to the Glucose Service module by calling 00022 * ble_gls_on_ble_evt() from the from the @ref ble_stack_handler callback. 00023 * 00024 * @note Attention! 00025 * To maintain compliance with Nordic Semiconductor ASA Bluetooth profile 00026 * qualification listings, this section of source code must not be modified. 00027 */ 00028 00029 #ifndef BLE_GLS_H__ 00030 #define BLE_GLS_H__ 00031 00032 #include <stdint.h> 00033 #include <stdbool.h> 00034 #include "ble.h" 00035 #include "ble_srv_common.h " 00036 #include "ble_date_time.h" 00037 00038 /**@brief Glucose feature */ 00039 #define BLE_GLS_FEATURE_LOW_BATT 0x0001 /**< Low Battery Detection During Measurement Supported */ 00040 #define BLE_GLS_FEATURE_MALFUNC 0x0002 /**< Sensor Malfunction Detection Supported */ 00041 #define BLE_GLS_FEATURE_SAMPLE_SIZE 0x0004 /**< Sensor Sample Size Supported */ 00042 #define BLE_GLS_FEATURE_INSERT_ERR 0x0008 /**< Sensor Strip Insertion Error Detection Supported */ 00043 #define BLE_GLS_FEATURE_TYPE_ERR 0x0010 /**< Sensor Strip Type Error Detection Supported */ 00044 #define BLE_GLS_FEATURE_RES_HIGH_LOW 0x0020 /**< Sensor Result High-Low Detection Supported */ 00045 #define BLE_GLS_FEATURE_TEMP_HIGH_LOW 0x0040 /**< Sensor Temperature High-Low Detection Supported */ 00046 #define BLE_GLS_FEATURE_READ_INT 0x0080 /**< Sensor Read Interrupt Detection Supported */ 00047 #define BLE_GLS_FEATURE_GENERAL_FAULT 0x0100 /**< General Device Fault Supported */ 00048 #define BLE_GLS_FEATURE_TIME_FAULT 0x0200 /**< Time Fault Supported */ 00049 #define BLE_GLS_FEATURE_MULTI_BOND 0x0400 /**< Multiple Bond Supported */ 00050 00051 /**@brief Glucose measurement flags */ 00052 #define BLE_GLS_MEAS_FLAG_TIME_OFFSET 0x01 /**< Time Offset Present */ 00053 #define BLE_GLS_MEAS_FLAG_CONC_TYPE_LOC 0x02 /**< Glucose Concentration, Type, and Sample Location Present */ 00054 #define BLE_GLS_MEAS_FLAG_UNITS_KG_L 0x00 /**< Glucose Concentration Units kg/L */ 00055 #define BLE_GLS_MEAS_FLAG_UNITS_MOL_L 0x04 /**< Glucose Concentration Units mol/L */ 00056 #define BLE_GLS_MEAS_FLAG_SENSOR_STATUS 0x08 /**< Sensor Status Annunciation Present */ 00057 #define BLE_GLS_MEAS_FLAG_CONTEXT_INFO 0x10 /**< Context Information Follows */ 00058 00059 /**@brief Glucose measurement type */ 00060 #define BLE_GLS_MEAS_TYPE_CAP_BLOOD 1 /**< Capillary whole blood */ 00061 #define BLE_GLS_MEAS_TYPE_CAP_PLASMA 2 /**< Capillary plasma */ 00062 #define BLE_GLS_MEAS_TYPE_VEN_BLOOD 3 /**< Venous whole blood */ 00063 #define BLE_GLS_MEAS_TYPE_VEN_PLASMA 4 /**< Venous plasma */ 00064 #define BLE_GLS_MEAS_TYPE_ART_BLOOD 5 /**< Arterial whole blood */ 00065 #define BLE_GLS_MEAS_TYPE_ART_PLASMA 6 /**< Arterial plasma */ 00066 #define BLE_GLS_MEAS_TYPE_UNDET_BLOOD 7 /**< Undetermined whole blood */ 00067 #define BLE_GLS_MEAS_TYPE_UNDET_PLASMA 8 /**< Undetermined plasma */ 00068 #define BLE_GLS_MEAS_TYPE_FLUID 9 /**< Interstitial fluid (ISF) */ 00069 #define BLE_GLS_MEAS_TYPE_CONTROL 10 /**< Control solution */ 00070 00071 /**@brief Glucose measurement location */ 00072 #define BLE_GLS_MEAS_LOC_FINGER 1 /**< Finger */ 00073 #define BLE_GLS_MEAS_LOC_AST 2 /**< Alternate Site Test (AST) */ 00074 #define BLE_GLS_MEAS_LOC_EAR 3 /**< Earlobe */ 00075 #define BLE_GLS_MEAS_LOC_CONTROL 4 /**< Control solution */ 00076 #define BLE_GLS_MEAS_LOC_NOT_AVAIL 15 /**< Sample Location value not available */ 00077 00078 /**@brief Glucose sensor status annunciation */ 00079 #define BLE_GLS_MEAS_STATUS_BATT_LOW 0x0001 /**< Device battery low at time of measurement */ 00080 #define BLE_GLS_MEAS_STATUS_SENSOR_FAULT 0x0002 /**< Sensor malfunction or faulting at time of measurement */ 00081 #define BLE_GLS_MEAS_STATUS_SAMPLE_SIZE 0x0004 /**< Sample size for blood or control solution insufficient at time of measurement */ 00082 #define BLE_GLS_MEAS_STATUS_STRIP_INSERT 0x0008 /**< Strip insertion error */ 00083 #define BLE_GLS_MEAS_STATUS_STRIP_TYPE 0x0010 /**< Strip type incorrect for device */ 00084 #define BLE_GLS_MEAS_STATUS_RESULT_HIGH 0x0020 /**< Sensor result higher than the device can process */ 00085 #define BLE_GLS_MEAS_STATUS_RESULT_LOW 0x0040 /**< Sensor result lower than the device can process */ 00086 #define BLE_GLS_MEAS_STATUS_TEMP_HIGH 0x0080 /**< Sensor temperature too high for valid test/result at time of measurement */ 00087 #define BLE_GLS_MEAS_STATUS_TEMP_LOW 0x0100 /**< Sensor temperature too low for valid test/result at time of measurement */ 00088 #define BLE_GLS_MEAS_STATUS_STRIP_PULL 0x0200 /**< Sensor read interrupted because strip was pulled too soon at time of measurement */ 00089 #define BLE_GLS_MEAS_STATUS_GENERAL_FAULT 0x0400 /**< General device fault has occurred in the sensor */ 00090 #define BLE_GLS_MEAS_STATUS_TIME_FAULT 0x0800 /**< Time fault has occurred in the sensor and time may be inaccurate */ 00091 00092 /**@brief Glucose measurement context flags */ 00093 #define BLE_GLS_CONTEXT_FLAG_CARB 0x01 /**< Carbohydrate id and carbohydrate present */ 00094 #define BLE_GLS_CONTEXT_FLAG_MEAL 0x02 /**< Meal present */ 00095 #define BLE_GLS_CONTEXT_FLAG_TESTER 0x04 /**< Tester-health present */ 00096 #define BLE_GLS_CONTEXT_FLAG_EXERCISE 0x08 /**< Exercise duration and exercise intensity present */ 00097 #define BLE_GLS_CONTEXT_FLAG_MED 0x10 /**< Medication ID and medication present */ 00098 #define BLE_GLS_CONTEXT_FLAG_MED_KG 0x00 /**< Medication value units, kilograms */ 00099 #define BLE_GLS_CONTEXT_FLAG_MED_L 0x20 /**< Medication value units, liters */ 00100 #define BLE_GLS_CONTEXT_FLAG_HBA1C 0x40 /**< Hba1c present */ 00101 #define BLE_GLS_CONTEXT_FLAG_EXT 0x80 /**< Extended flags present */ 00102 00103 /**@brief Glucose measurement context carbohydrate ID */ 00104 #define BLE_GLS_CONTEXT_CARB_BREAKFAST 1 /**< Breakfast */ 00105 #define BLE_GLS_CONTEXT_CARB_LUNCH 2 /**< Lunch */ 00106 #define BLE_GLS_CONTEXT_CARB_DINNER 3 /**< Dinner */ 00107 #define BLE_GLS_CONTEXT_CARB_SNACK 4 /**< Snack */ 00108 #define BLE_GLS_CONTEXT_CARB_DRINK 5 /**< Drink */ 00109 #define BLE_GLS_CONTEXT_CARB_SUPPER 6 /**< Supper */ 00110 #define BLE_GLS_CONTEXT_CARB_BRUNCH 7 /**< Brunch */ 00111 00112 /**@brief Glucose measurement context meal */ 00113 #define BLE_GLS_CONTEXT_MEAL_PREPRANDIAL 1 /**< Preprandial (before meal) */ 00114 #define BLE_GLS_CONTEXT_MEAL_POSTPRANDIAL 2 /**< Postprandial (after meal) */ 00115 #define BLE_GLS_CONTEXT_MEAL_FASTING 3 /**< Fasting */ 00116 #define BLE_GLS_CONTEXT_MEAL_CASUAL 4 /**< Casual (snacks, drinks, etc.) */ 00117 #define BLE_GLS_CONTEXT_MEAL_BEDTIME 5 /**< Bedtime */ 00118 00119 /**@brief Glucose measurement context tester */ 00120 #define BLE_GLS_CONTEXT_TESTER_SELF 1 /**< Self */ 00121 #define BLE_GLS_CONTEXT_TESTER_PRO 2 /**< Health care professional */ 00122 #define BLE_GLS_CONTEXT_TESTER_LAB 3 /**< Lab test */ 00123 #define BLE_GLS_CONTEXT_TESTER_NOT_AVAIL 15 /**< Tester value not available */ 00124 00125 /**@brief Glucose measurement context health */ 00126 #define BLE_GLS_CONTEXT_HEALTH_MINOR 1 /**< Minor health issues */ 00127 #define BLE_GLS_CONTEXT_HEALTH_MAJOR 2 /**< Major health issues */ 00128 #define BLE_GLS_CONTEXT_HEALTH_MENSES 3 /**< During menses */ 00129 #define BLE_GLS_CONTEXT_HEALTH_STRESS 4 /**< Under stress */ 00130 #define BLE_GLS_CONTEXT_HEALTH_NONE 5 /**< No health issues */ 00131 #define BLE_GLS_CONTEXT_HEALTH_NOT_AVAIL 15 /**< Health value not available */ 00132 00133 /**@brief Glucose measurement context medication ID */ 00134 #define BLE_GLS_CONTEXT_MED_RAPID 1 /**< Rapid acting insulin */ 00135 #define BLE_GLS_CONTEXT_MED_SHORT 2 /**< Short acting insulin */ 00136 #define BLE_GLS_CONTEXT_MED_INTERMED 3 /**< Intermediate acting insulin */ 00137 #define BLE_GLS_CONTEXT_MED_LONG 4 /**< Long acting insulin */ 00138 #define BLE_GLS_CONTEXT_MED_PREMIX 5 /**< Pre-mixed insulin */ 00139 00140 /**@brief SFLOAT format (IEEE-11073 16-bit FLOAT, meaning 4 bits for exponent (base 10) and 12 bits mantissa) */ 00141 typedef struct 00142 { 00143 int8_t exponent; /**< Base 10 exponent, should be using only 4 bits */ 00144 int16_t mantissa; /**< Mantissa, should be using only 12 bits */ 00145 } sfloat_t; 00146 00147 /**@brief Glucose Service event type. */ 00148 typedef enum 00149 { 00150 BLE_GLS_EVT_NOTIFICATION_ENABLED, /**< Glucose value notification enabled event. */ 00151 BLE_GLS_EVT_NOTIFICATION_DISABLED /**< Glucose value notification disabled event. */ 00152 } ble_gls_evt_type_t; 00153 00154 /**@brief Glucose Service event. */ 00155 typedef struct 00156 { 00157 ble_gls_evt_type_t evt_type; /**< Type of event. */ 00158 } ble_gls_evt_t; 00159 00160 // Forward declaration of the ble_gls_t type. 00161 typedef struct ble_gls_s ble_gls_t; 00162 00163 /**@brief Glucose Service event handler type. */ 00164 typedef void (*ble_gls_evt_handler_t) (ble_gls_t * p_gls, ble_gls_evt_t * p_evt); 00165 00166 /**@brief Glucose Measurement structure. This contains glucose measurement value. */ 00167 typedef struct 00168 { 00169 uint8_t flags; /**< Flags */ 00170 uint16_t sequence_number; /**< Sequence number */ 00171 ble_date_time_t base_time; /**< Time stamp */ 00172 int16_t time_offset; /**< Time offset */ 00173 sfloat_t glucose_concentration; /**< Glucose concentration */ 00174 uint8_t type; /**< Type */ 00175 uint8_t sample_location; /**< Sample location */ 00176 uint16_t sensor_status_annunciation; /**< Sensor status annunciation */ 00177 } ble_gls_meas_t; 00178 00179 /**@brief Glucose measurement context structure */ 00180 typedef struct 00181 { 00182 uint8_t flags; /**< Flags */ 00183 uint8_t extended_flags; /**< Extended Flags */ 00184 uint8_t carbohydrate_id; /**< Carbohydrate ID */ 00185 sfloat_t carbohydrate; /**< Carbohydrate */ 00186 uint8_t meal; /**< Meal */ 00187 uint8_t tester_and_health; /**< Tester and health */ 00188 uint16_t exercise_duration; /**< Exercise Duration */ 00189 uint8_t exercise_intensity; /**< Exercise Intensity */ 00190 uint8_t medication_id; /**< Medication ID */ 00191 sfloat_t medication; /**< Medication */ 00192 uint16_t hba1c; /**< HbA1c */ 00193 } ble_gls_meas_context_t; 00194 00195 /**@brief Glucose measurement record */ 00196 typedef struct 00197 { 00198 ble_gls_meas_t meas; /**< Glucose measurement */ 00199 ble_gls_meas_context_t context; /**< Glucose measurement context */ 00200 } ble_gls_rec_t; 00201 00202 /**@brief Glucose Service init structure. This contains all options and data needed for 00203 * initialization of the service. */ 00204 typedef struct 00205 { 00206 ble_gls_evt_handler_t evt_handler; /**< Event handler to be called for handling events in the Glucose Service. */ 00207 ble_srv_error_handler_t error_handler; /**< Function to be called in case of an error. */ 00208 uint16_t feature; /**< Glucose Feature value indicating supported features. */ 00209 bool is_context_supported; /**< Determines if optional Glucose Measurement Context is to be supported. */ 00210 } ble_gls_init_t; 00211 00212 /**@brief Glucose Service structure. This contains various status information for the service. */ 00213 typedef struct ble_gls_s 00214 { 00215 ble_gls_evt_handler_t evt_handler; /**< Event handler to be called for handling events in the Glucose Service. */ 00216 ble_srv_error_handler_t error_handler; /**< Function to be called in case of an error. */ 00217 uint16_t service_handle; /**< Handle of Glucose Service (as provided by the BLE stack). */ 00218 ble_gatts_char_handles_t glm_handles; /**< Handles related to the Glucose Measurement characteristic. */ 00219 ble_gatts_char_handles_t glm_context_handles; /**< Handles related to the Glucose Measurement Context characteristic. */ 00220 ble_gatts_char_handles_t glf_handles; /**< Handles related to the Glucose Feature characteristic. */ 00221 ble_gatts_char_handles_t racp_handles; /**< Handles related to the Record Access Control Point characteristic. */ 00222 uint16_t conn_handle; /**< Handle of the current connection (as provided by the BLE stack, is BLE_CONN_HANDLE_INVALID if not in a connection). */ 00223 uint16_t feature; 00224 bool is_context_supported; 00225 } ble_gls_t; 00226 00227 /**@brief Function for initializing the Glucose Service. 00228 * 00229 * @details This call allows the application to initialize the Glucose Service. 00230 * 00231 * @param[out] p_gls Glucose Service structure. This structure will have to be supplied by 00232 * the application. It will be initialized by this function, and will later 00233 * be used to identify this particular service instance. 00234 * @param[in] p_gls_init Information needed to initialize the service. 00235 * 00236 * @return NRF_SUCCESS on successful initialization of service, otherwise an error code. 00237 */ 00238 uint32_t ble_gls_init(ble_gls_t * p_gls, const ble_gls_init_t * p_gls_init); 00239 00240 /**@brief Function for handling the Application's BLE Stack events. 00241 * 00242 * @details Handles all events from the BLE stack of interest to the Glucose Service. 00243 * 00244 * @param[in] p_gls Glucose Service structure. 00245 * @param[in] p_ble_evt Event received from the BLE stack. 00246 */ 00247 void ble_gls_on_ble_evt(ble_gls_t * p_gls, ble_evt_t * p_ble_evt); 00248 00249 /**@brief Function for reporting a new glucose measurement to the glucose service module. 00250 * 00251 * @details The application calls this function after having performed a new glucose measurement. 00252 * The new measurement is recorded in the RACP database. 00253 * 00254 * @param[in] p_gls Glucose Service structure. 00255 * @param[in] p_rec Pointer to glucose record (measurement plus context). 00256 * 00257 * @return NRF_SUCCESS on success, otherwise an error code. 00258 */ 00259 uint32_t ble_gls_glucose_new_meas(ble_gls_t * p_gls, ble_gls_rec_t * p_rec); 00260 00261 #endif // BLE_GLS_H__ 00262 00263 /** @} */ 00264 00265 /** @endcond */
Generated on Tue Jul 12 2022 15:15:13 by
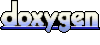