
Code for the Nordic nrf51822 component of our project. For project group Alloy.
Dependencies: BLE_API mbed nRF51822
Fork of idd_hw5_group_alloy_BLE by
main.cpp
00001 /** 00002 * Interactive Device Design, Fall 2014 00003 * Homework 5 - Wind and Window controller 00004 * Nordic nrf51822 Board 00005 * 00006 * Ian Shain 00007 * Doug Cook 00008 * Kiyana Salkeld 00009 * Elizabeth Lin 00010 * 00011 */ 00012 00013 #include "mbed.h" 00014 #include "BLEDevice.h" 00015 #include "HealthThermometerService.h" 00016 #include "UARTService.h" 00017 #include <string> 00018 00019 BLEDevice ble; 00020 DigitalOut led1(LED1); 00021 DigitalOut led2(LED2); 00022 00023 UARTService *uartServicePtr; 00024 00025 Serial kl25z(P0_9, P0_11); // P0_9 (gray) -> TX, P0_11 (purple) -> RX 00026 00027 void disconnectionCallback(Gap::Handle_t handle, Gap::DisconnectionReason_t reason) 00028 { 00029 kl25z.putc('h'); 00030 kl25z.putc('f'); 00031 ble.startAdvertising(); 00032 } 00033 00034 void onDataWritten(const GattCharacteristicWriteCBParams *params) 00035 { 00036 if ((uartServicePtr != NULL) && (params->charHandle == uartServicePtr->getTXCharacteristicHandle())) { 00037 uint16_t bytesRead = params->len; 00038 if (bytesRead <= 1) { 00039 kl25z.putc('c'); 00040 } else { 00041 kl25z.putc('d'); 00042 } 00043 ble.updateCharacteristicValue(uartServicePtr->getRXCharacteristicHandle(), params->data, bytesRead); 00044 } 00045 } 00046 00047 bool updateWindAdvertisement = false; 00048 00049 void periodicCallback(void) 00050 { 00051 led1 = !led1; 00052 updateWindAdvertisement = true; 00053 } 00054 00055 bool newMessage = false; 00056 string measurement = ""; 00057 void callback() { 00058 newMessage = true; 00059 measurement += kl25z.getc(); 00060 } 00061 00062 int main(void) 00063 { 00064 led1 = 1; 00065 Ticker ticker; 00066 ticker.attach(periodicCallback, 1); 00067 00068 kl25z.baud(9600); 00069 kl25z.format(8,Serial::Odd,1); 00070 kl25z.attach(callback); 00071 00072 //pc.printf("Initialising the nRF51822\n\r"); 00073 kl25z.putc('e'); 00074 ble.init(); 00075 ble.onDisconnection(disconnectionCallback); 00076 ble.onDataWritten(onDataWritten); 00077 00078 HealthThermometerService thermometerService(ble, 0, HealthThermometerService::LOCATION_EAR); 00079 00080 /* setup advertising */ 00081 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00082 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00083 ble.accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, 00084 (const uint8_t *)"AlloyWindow", sizeof("AlloyWindow") - 1); 00085 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00086 (const uint8_t *)UARTServiceUUID_reversed, sizeof(UARTServiceUUID_reversed)); 00087 00088 ble.setAdvertisingInterval(160); /* 100ms; in multiples of 0.625ms. */ 00089 ble.startAdvertising(); 00090 00091 UARTService uartService(ble); 00092 uartServicePtr = &uartService; 00093 00094 while (true) { 00095 if (newMessage) { 00096 led2 = !led2; // data receiver notification 00097 // broadcast wind speed value 00098 thermometerService.updateTemperature(atoi(measurement.c_str())); 00099 measurement = ""; 00100 newMessage = false; 00101 } else { 00102 ble.waitForEvent(); 00103 } 00104 } 00105 }
Generated on Sun Jul 17 2022 15:26:03 by
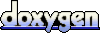