CAMCTL - One shot capture. - Calibration of camera to be able to work under different light conditions.
Dependents: frdm_MasterVehicle
camctl.h
00001 #ifndef _CAMCTL_H_ 00002 #define _CAMCTL_H_ 00003 00004 #include "mbed.h" 00005 00006 /********************************************************************************** 00007 * Camera parameters 00008 ***********************************************************************************/ 00009 00010 #define BASE_PERIOD 20/* us 00011 * Description: All times are based on this time 00012 */ 00013 00014 #define START_DELAY 10/* x1000 = 1ms 00015 * Description: All times are based on this time 00016 */ 00017 00018 #define SI_PULSE_WIDTH 15/* x BASE_PERIOD = 10ms 00019 * Description: Pulse width of SI signal 00020 */ 00021 00022 00023 #define SI_PULSE_WIDTH_HALF (SI_PULSE_WIDTH/2)/* x BASE_PERIOD = 10ms 00024 * Description: Pulse width of SI signal 00025 */ 00026 00027 #define CLK_PULSE_WIDTH_HALF (SI_PULSE_WIDTH/2)/* x BASE_PERIOD = 10ms 00028 * Description: Pulse width of SI signal 00029 */ 00030 00031 /*#define EXPOSURE_TIME (uint8)(15)*//* x BASE_PERIOD = 10ms 00032 * Description: Time between firts SI pulse and second one. 00033 */ 00034 00035 #define CLK_PULSE_WIDTH SI_PULSE_WIDTH/* x BASE_PERIOD = 10ms 00036 * Description: Must be the same value used for SI_PULSE_WIDTH 00037 */ 00038 00039 #define NO_OF_CLK_PULSES 129/* 00040 * Description: Number of pulses to trigger between 2 SI pulses. 00041 */ 00042 00043 #define IDLE_TIME 15/*us 00044 * Description: Time to wait before the next SI pulse. 00045 */ 00046 00047 #define BUFFER_LENGTH 128/* 00048 * Description: Number of to get from linescan camera. 00049 */ 00050 00051 /********************************************************************************** 00052 * Variable types definition 00053 ***********************************************************************************/ 00054 typedef enum 00055 { 00056 nContinuous = 0, 00057 nOneShot, 00058 nNone, 00059 nNumberOfModes 00060 }CAMCTL__tenCaptureMode; 00061 00062 typedef struct 00063 { 00064 uint8_t u8ActiveCapture : 1; 00065 uint8_t u8ClkEnd : 1; 00066 CAMCTL__tenCaptureMode enCaptureMode; 00067 }CAMCTL__tstCameraOptions; 00068 00069 /********************************************************************************** 00070 * Global functions 00071 ***********************************************************************************/ 00072 void CAMCTL_vInit(void); 00073 void CAMCTL_vTriggerOneShotCapture(void); 00074 void CAMCTL_vTriggerContinuousCapture(void); 00075 void CAMCTL_vStopContinuousCapture(void); 00076 uint16_t * CAMCTL_pu16GetData( void ); 00077 bool CAMCTL_boCalibrateCamera(uint16_t* pu16ThreshCalibration, uint8_t u8Frames2Wait); 00078 00079 #endif /* END OF _CAMCTL_H_ */
Generated on Thu Jul 21 2022 03:34:01 by
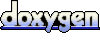