CAMCTL - One shot capture. - Calibration of camera to be able to work under different light conditions.
Dependents: frdm_MasterVehicle
camctl.cpp
00001 #include "camctl.h" 00002 00003 /********************************************************************************** 00004 * Object declaration 00005 ***********************************************************************************/ 00006 00007 DigitalOut CAMCTL__xPinSI(PTD1); 00008 DigitalOut CAMCTL__xPinCLK(PTD3); 00009 AnalogIn CAMCTL__xPinAN(PTB2); 00010 DigitalOut debug(PTB3); 00011 DigitalOut debugGetData(PTB10); 00012 00013 Timeout CAMCTL__xGlobalTimer; 00014 Timeout CAMCTL__xClkTimer; 00015 Timeout CAMCTL__xSiTimer; 00016 00017 /********************************************************************************** 00018 * Local function prototypes 00019 ***********************************************************************************/ 00020 static void CAMCTL__vGlobalTimer(void); 00021 static void CAMCTL__vSignalHdlr_SI(void); 00022 static void CAMCTL__vSignalHdlr_CLK(void); 00023 00024 /********************************************************************************** 00025 * Local variables 00026 ***********************************************************************************/ 00027 static CAMCTL__tstCameraOptions CAMCTL__stCameraOptions; 00028 static uint16_t CAMCTL__au16LineBuffer[BUFFER_LENGTH] = {0}; 00029 00030 /********************************************************************************** 00031 * CAMCTL-Module 00032 ***********************************************************************************/ 00033 static void CAMCTL__vGlobalTimer() 00034 { 00035 static bool boStartDone = false; 00036 if ( CAMCTL__stCameraOptions.enCaptureMode == nOneShot && 00037 CAMCTL__stCameraOptions.u8ActiveCapture == true && 00038 CAMCTL__stCameraOptions.u8ClkEnd == false && 00039 boStartDone == false ) 00040 { 00041 CAMCTL__xSiTimer.attach_us(&CAMCTL__vSignalHdlr_SI, START_DELAY); 00042 CAMCTL__xClkTimer.attach_us(&CAMCTL__vSignalHdlr_CLK, START_DELAY + CLK_PULSE_WIDTH_HALF); 00043 boStartDone = true; 00044 } 00045 else if( CAMCTL__stCameraOptions.enCaptureMode == nOneShot && 00046 CAMCTL__stCameraOptions.u8ActiveCapture == true && 00047 CAMCTL__stCameraOptions.u8ClkEnd == true && 00048 boStartDone == true ) 00049 { 00050 boStartDone = false; 00051 CAMCTL__xGlobalTimer.detach(); 00052 CAMCTL__xClkTimer.detach(); 00053 CAMCTL__xSiTimer.detach(); 00054 } 00055 else 00056 { 00057 } 00058 CAMCTL__xGlobalTimer.attach_us(&CAMCTL__vGlobalTimer,BASE_PERIOD); 00059 } 00060 void CAMCTL__vSignalHdlr_SI() 00061 { 00062 static bool boSIDone = false; 00063 if( boSIDone == false ) 00064 { 00065 boSIDone = true; 00066 CAMCTL__xPinSI = true; 00067 CAMCTL__xSiTimer.attach_us(&CAMCTL__vSignalHdlr_SI, SI_PULSE_WIDTH); 00068 } 00069 else 00070 { 00071 CAMCTL__xPinSI = false; 00072 boSIDone = false; 00073 } 00074 } 00075 void CAMCTL__vSignalHdlr_CLK() 00076 { 00077 static uint8_t u8PulseCtr = 0; 00078 00079 if( u8PulseCtr < NO_OF_CLK_PULSES ) 00080 { 00081 if ( u8PulseCtr == 0 ) 00082 { 00083 CAMCTL__xPinCLK = true; 00084 u8PulseCtr++; 00085 } 00086 else 00087 { 00088 CAMCTL__xPinCLK = !CAMCTL__xPinCLK; 00089 if( CAMCTL__xPinCLK == false ) 00090 { 00091 if( ( u8PulseCtr >= 1 ) && (u8PulseCtr < BUFFER_LENGTH ) ) 00092 { 00093 CAMCTL__au16LineBuffer[u8PulseCtr-1] = CAMCTL__xPinAN.read_u16(); 00094 } 00095 u8PulseCtr++; 00096 } 00097 } 00098 CAMCTL__xClkTimer.attach_us(&CAMCTL__vSignalHdlr_CLK, CLK_PULSE_WIDTH); 00099 } 00100 else 00101 { 00102 u8PulseCtr = 0; 00103 CAMCTL__stCameraOptions.u8ClkEnd = true; 00104 } 00105 00106 } 00107 00108 void CAMCTL_vTriggerOneShotCapture() 00109 { 00110 CAMCTL__stCameraOptions.u8ActiveCapture = true; 00111 CAMCTL__stCameraOptions.u8ClkEnd = false; 00112 CAMCTL__stCameraOptions.enCaptureMode = nOneShot; 00113 CAMCTL__xGlobalTimer.attach_us(&CAMCTL__vGlobalTimer,BASE_PERIOD); 00114 } 00115 00116 void CAMCTL_vTriggerContinuousCapture() 00117 { 00118 CAMCTL__stCameraOptions.u8ActiveCapture = true; 00119 CAMCTL__stCameraOptions.u8ClkEnd = false; 00120 CAMCTL__stCameraOptions.enCaptureMode = nContinuous; 00121 CAMCTL__xGlobalTimer.attach_us(&CAMCTL__vGlobalTimer,BASE_PERIOD); 00122 } 00123 00124 void CAMCTL_vStopContinuousCapture( void ) 00125 { 00126 CAMCTL__stCameraOptions.u8ActiveCapture = false; 00127 CAMCTL__stCameraOptions.enCaptureMode = nNone; 00128 CAMCTL__xGlobalTimer.detach(); 00129 CAMCTL__xClkTimer.detach(); 00130 CAMCTL__xSiTimer.detach(); 00131 } 00132 00133 uint16_t * CAMCTL_pu16GetData( void ) 00134 { 00135 debugGetData = !debugGetData; 00136 debug = CAMCTL__stCameraOptions.u8ClkEnd; 00137 if( CAMCTL__stCameraOptions.u8ClkEnd == true ) 00138 { 00139 return CAMCTL__au16LineBuffer; 00140 } 00141 else 00142 { 00143 return NULL; 00144 } 00145 } 00146 00147 void CAMCTL_vInit(void) 00148 { 00149 CAMCTL__stCameraOptions.u8ActiveCapture = false; 00150 CAMCTL__stCameraOptions.u8ClkEnd = false; 00151 CAMCTL__stCameraOptions.enCaptureMode = nNone; 00152 } 00153 00154 bool CAMCTL_boCalibrateCamera(uint16_t* pu16ThreshCalibration, uint8_t u8Frames2Wait) 00155 { 00156 static uint8_t u8FrameCtr = 0; 00157 uint16_t* pu16DataBuffer; 00158 bool boConvDone = false; 00159 00160 CAMCTL_vTriggerOneShotCapture(); 00161 pu16DataBuffer = NULL; 00162 while( pu16DataBuffer == NULL ) 00163 { 00164 pu16DataBuffer = CAMCTL_pu16GetData(); 00165 } 00166 u8FrameCtr++; 00167 if( u8FrameCtr > u8Frames2Wait ) 00168 { 00169 u8FrameCtr = 0; 00170 boConvDone = true; 00171 memcpy( pu16ThreshCalibration, pu16DataBuffer, (BUFFER_LENGTH)*sizeof(uint16_t)); 00172 } 00173 return boConvDone; 00174 }
Generated on Thu Jul 21 2022 03:34:01 by
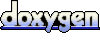