
Bus alarm system with WIZwiki-W7500 for WIZnet Academy
Dependencies: Adafruit_GFX WIZnetInterface mbed
Fork of Bus_Alarm_System_Helloworld_WIZwiki-W7500 by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "Adafruit_SSD1306.h" 00004 00005 class I2CPreInit : public I2C 00006 { 00007 public: 00008 I2CPreInit(PinName sda, PinName scl) : I2C(sda, scl) 00009 { 00010 frequency(100000); 00011 start(); 00012 }; 00013 }; 00014 00015 I2CPreInit gI2C(PA_10,PA_9); 00016 Adafruit_SSD1306_I2c gOled(gI2C,NC,0x78,64,128); 00017 00018 //internal led 00019 DigitalOut red_led(LED1,1); 00020 DigitalOut blue_led(LED3,1); 00021 00022 int main() 00023 { 00024 int phy_link; 00025 printf("Wait a second...\r\n"); 00026 uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0x00, 0x01, 0x09}; 00027 00028 EthernetInterface eth; 00029 eth.init(mac_addr); //Use DHCP 00030 00031 eth.connect(); 00032 00033 //phy link 00034 do{ 00035 phy_link = eth.ethernet_link(); 00036 printf("..."); 00037 wait(2); 00038 }while(!phy_link); 00039 printf("IP Address is %s\r\n\r\n", eth.getIPAddress()); 00040 00041 char buffer[1024]; 00042 int ret; 00043 00044 //BUS "GET /ws/rest/busarrivalservice?serviceKey=??&routeId=??&stationId=?? \n\n" 00045 char http_cmd[] = "GET /ws/rest/busarrivalservice?serviceKey=1234567890&routeId=234000878&stationId=206000082 \n\n"; // 8100 00046 00047 while(1) 00048 { 00049 //TCP socket connect 00050 TCPSocketConnection sock; 00051 sock.connect("openapi.gbis.go.kr", 80); 00052 00053 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00054 00055 while (true) 00056 { 00057 ret = sock.receive(buffer, sizeof(buffer)-1); 00058 00059 if (ret <= 0) 00060 printf("fail\r\n"); 00061 //break; 00062 else{ 00063 buffer[ret] = '\0'; 00064 printf("Received %d chars from server: %s\r\n", ret, buffer); 00065 break; 00066 } 00067 } 00068 printf("\r\n\r\n"); 00069 00070 00071 // parsing data 00072 char *date; 00073 char *bus_1st; 00074 char *bus_2nd; 00075 00076 char current_date[11] = {0}; 00077 char arrive_bus1[2] = {0}; 00078 char arrive_bus2[2] = {0}; 00079 00080 date = strstr(buffer, "<queryTime>"); 00081 for(int i=0;i<10;i++){ 00082 current_date[i] = date[i+11]; 00083 } 00084 00085 bus_1st = strstr(buffer, "<predictTime1>"); 00086 for(int i=0; i<2;i++){ 00087 arrive_bus1[i] = bus_1st[i+14]; 00088 if(arrive_bus1[i] == '<'){ 00089 arrive_bus1[i] = 0; 00090 break; 00091 } 00092 } 00093 00094 bus_2nd = strstr(buffer, "<predictTime2>"); 00095 for(int i=0; i<2;i++){ 00096 arrive_bus2[i] = bus_2nd[i+14]; 00097 if(arrive_bus2[i] == '<'){ 00098 arrive_bus2[i] = 0; 00099 break; 00100 } 00101 } 00102 00103 printf("current date : %s\r\n", current_date); 00104 printf("arrival bus1 : %s\r\n", arrive_bus1); 00105 printf("arrival bus2 : %s\r\n", arrive_bus2); 00106 00107 00108 // OLED Display 00109 gOled.begin(); 00110 gOled.clearDisplay(); 00111 00112 gOled.printf("%s\n\n", current_date); 00113 gOled.printf("No.8100\n\n"); 00114 gOled.printf("You have %s mins\n\n", arrive_bus1); 00115 gOled.printf("Next Bus %s mins\n", arrive_bus2); 00116 gOled.display(); 00117 gOled.setTextCursor(0,0); 00118 00119 wait(12); 00120 }; 00121 00122 }
Generated on Mon Jul 18 2022 22:46:41 by
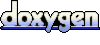