
Advanced gyroscope program example for Hexiwear featuring OLED Display
Dependencies: FXAS21002 Hexi_OLED_SSD1351
Fork of Hexi_Gyro-v2_Example by
main.cpp
00001 #include "mbed.h" 00002 #include "FXAS21002.h" 00003 #include "Hexi_OLED_SSD1351.h" 00004 #include "images.h" 00005 #include "string.h" 00006 00007 // Pin connections 00008 DigitalOut led1(LED_GREEN); // RGB LED 00009 Serial pc(USBTX, USBRX); // Serial interface 00010 FXAS21002 gyro(PTC11,PTC10); // Gyroscope 00011 SSD1351 oled(PTB22,PTB21,PTC13,PTB20,PTE6, PTD15); // SSD1351 OLED Driver (MOSI,SCLK,POWER,CS,RST,DC) 00012 00013 // Variables 00014 float gyro_data[3]; // Storage for the data from the sensor 00015 float gyro_rms=0.0; // RMS value from the sensor 00016 float gx, gy, gz; // Integer value from the sensor to be displayed 00017 const uint8_t *image1; // Pointer for the image1 to be displayed 00018 char text1[20]; // Text Buffer for dynamic value displayed 00019 char text2[20]; // Text Buffer for dynamic value displayed 00020 char text3[20]; // Text Buffer for dynamic value displayed 00021 00022 int main() { 00023 00024 // Configure Gyroscope FXAS21002 00025 gyro.gyro_config(); 00026 00027 /* Setting pointer location of the 96 by 96 pixel bitmap */ 00028 image1 = Gyro; 00029 00030 // Dimm Down OLED backlight 00031 // oled.DimScreenON(); 00032 00033 /* Fill 96px by 96px Screen with 96px by 96px NXP Image starting at x=0,y=0 */ 00034 oled.DrawImage(image1,0,0); 00035 00036 00037 while (true) 00038 { 00039 00040 gyro.acquire_gyro_data_dps(gyro_data); 00041 gyro_rms = sqrt(((gyro_data[0]*gyro_data[0])+(gyro_data[1]*gyro_data[1])+(gyro_data[2]*gyro_data[2]))/3); 00042 pc.printf("Gyroscope \tRoll (G) %4.2f \tPitch (G) %4.2f \tYaw (G) %4.2f \tRMS %4.2f\n\r",gyro_data[0],gyro_data[1],gyro_data[2],gyro_rms); 00043 wait(0.01); 00044 gx = gyro_data[0]; 00045 gy = gyro_data[1]; 00046 gz = gyro_data[2]; 00047 00048 /* Get OLED Class Default Text Properties */ 00049 oled_text_properties_t textProperties = {0}; 00050 oled.GetTextProperties(&textProperties); 00051 00052 /* Set text properties to white and right aligned for the dynamic text */ 00053 textProperties.fontColor = COLOR_BLUE; 00054 textProperties.alignParam = OLED_TEXT_ALIGN_LEFT; 00055 oled.SetTextProperties(&textProperties); 00056 00057 /* Display Legends */ 00058 strcpy((char *) text1,"Roll (dps):"); 00059 oled.Label((uint8_t *)text1,3,45); 00060 00061 /* Format the value */ 00062 sprintf(text1,"%4.2f",gx); 00063 /* Display time reading in 35px by 15px textbox at(x=55, y=40) */ 00064 oled.TextBox((uint8_t *)text1,70,45,20,15); //Increase textbox for more digits 00065 00066 /* Set text properties to white and right aligned for the dynamic text */ 00067 textProperties.fontColor = COLOR_GREEN; 00068 textProperties.alignParam = OLED_TEXT_ALIGN_LEFT; 00069 oled.SetTextProperties(&textProperties); 00070 00071 /* Display Legends */ 00072 strcpy((char *) text2,"Pitch (dps):"); 00073 oled.Label((uint8_t *)text2,3,62); 00074 00075 /* Format the value */ 00076 sprintf(text2,"%4.2f",gy); 00077 /* Display time reading in 35px by 15px textbox at(x=55, y=40) */ 00078 oled.TextBox((uint8_t *)text2,70,62,20,15); //Increase textbox for more digits 00079 00080 /* Set text properties to white and right aligned for the dynamic text */ 00081 textProperties.fontColor = COLOR_RED; 00082 textProperties.alignParam = OLED_TEXT_ALIGN_LEFT; 00083 oled.SetTextProperties(&textProperties); 00084 00085 /* Display Legends */ 00086 strcpy((char *) text3,"Yaw (dps):"); 00087 oled.Label((uint8_t *)text3,3,79); 00088 00089 /* Format the value */ 00090 sprintf(text3,"%4.2f",gz); 00091 /* Display time reading in 35px by 15px textbox at(x=55, y=40) */ 00092 oled.TextBox((uint8_t *)text3,70,79,20,15); //Increase textbox for more digits 00093 00094 led1 = !led1; 00095 Thread::wait(250); 00096 } 00097 }
Generated on Wed Jul 13 2022 09:01:23 by
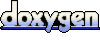