
Click Relay example for Hexiwear featuring Touch buttons and Haptic feedback
Dependencies: Hexi_KW40Z
main.cpp
00001 #include "mbed.h" 00002 #include "Hexi_KW40Z.h" 00003 00004 #define LED_ON 0 00005 #define LED_OFF 1 00006 00007 void StartHaptic(void); 00008 void StopHaptic(void const *n); 00009 00010 DigitalOut relay1(PTA10); 00011 DigitalOut relay2(PTC4); 00012 00013 DigitalOut redLed(LED1); 00014 DigitalOut greenLed(LED2); 00015 DigitalOut blueLed(LED3); 00016 DigitalOut haptic(PTB9); 00017 00018 /* Define timer for haptic feedback */ 00019 RtosTimer hapticTimer(StopHaptic, osTimerOnce); 00020 00021 /* Instantiate the Hexi KW40Z Driver (UART TX, UART RX) */ 00022 KW40Z kw40z_device(PTE24, PTE25); 00023 00024 void ButtonLeft(void) 00025 { 00026 StartHaptic(); 00027 00028 redLed = LED_ON; 00029 greenLed = LED_ON; 00030 blueLed = LED_OFF; 00031 00032 relay2 = !relay2; 00033 } 00034 00035 void ButtonRight(void) 00036 { 00037 StartHaptic(); 00038 00039 redLed = LED_ON; 00040 greenLed = LED_OFF; 00041 blueLed = LED_OFF; 00042 00043 relay1 = !relay1; 00044 } 00045 00046 void StartHaptic(void) 00047 { 00048 hapticTimer.start(75); 00049 haptic = 1; 00050 } 00051 00052 void StopHaptic(void const *n) { 00053 haptic = 0; 00054 hapticTimer.stop(); 00055 redLed = LED_OFF; 00056 greenLed = LED_OFF; 00057 blueLed = LED_OFF; 00058 } 00059 00060 int main() 00061 { 00062 redLed = LED_OFF; 00063 greenLed = LED_OFF; 00064 blueLed = LED_OFF; 00065 /* Register callbacks to application functions */ 00066 kw40z_device.attach_buttonLeft(&ButtonLeft); 00067 kw40z_device.attach_buttonRight(&ButtonRight); 00068 00069 while (true) { 00070 Thread::wait(500); 00071 } 00072 } 00073 00074
Generated on Fri Jul 29 2022 18:34:46 by
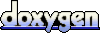