
Simple catch the dot game
Dependencies: FXOS8700 Hexi_KW40Z NeatGUI
Fork of Hexi_Bubble_Game by
main.cpp
00001 //HexiGame.cpp 00002 //R. Scott Coppersmith 00003 //Build 23 September 2016 00004 #include "mbed.h" 00005 #include <SSD1351_SPI.h> 00006 #include <HexiGame.h> 00007 #include "FXOS8700.h" 00008 #include "Hexi_KW40Z.h" 00009 00010 #define LED_ON 0 00011 #define LED_OFF 1 00012 00013 void StartHaptic(void); 00014 void StopHaptic(void const *n); 00015 AnalogIn analog(PTB2); 00016 Serial pc(USBTX, USBRX); 00017 unsigned int m_z = 22222; 00018 unsigned int m_w = 98764; 00019 unsigned int rgen (void) 00020 { 00021 00022 m_z = 36969 * (m_z & 65535) + (m_z >>16); 00023 m_w = 18000 * (m_w & 65535) + (m_w >>16); 00024 return ((m_z <<16) + m_w); 00025 } 00026 00027 //SSD1351_SPI ( mosi, miso, sclk, cs, dc ) ; 00028 SSD1351_SPI OLED96x96(PTB22,PTB23,PTB21,PTB20,PTD15); 00029 // Pin connections for Hexiwear 00030 FXOS8700 accel(PTC11, PTC10); 00031 // Storage for the data from the sensor 00032 float accel_data[3]; float accel_rms=0.0; 00033 DigitalOut led1(LED1); 00034 DigitalOut led2(LED2); 00035 DigitalOut led3(LED3); 00036 DigitalOut BOOSTEN(PTC13); //oled power enable 00037 DigitalOut haptic(PTB9); 00038 int count = 0; 00039 int count2 = 0; 00040 int count3 = 0; 00041 extern const uint8_t Main_screen_bmp[ 36864 ]; 00042 int argb = 0xff000000; 00043 int rgbd = 0x00000000; 00044 int xposg = 0; 00045 int yposg = 0; 00046 int randx = 0; 00047 int randy = 0; 00048 int main() { 00049 led1 = 1; 00050 led2 = 1; 00051 led3 = 1; 00052 pc.printf("HexiGame \r\n"); 00053 // Configure Accelerometer FXOS8700 00054 accel.accel_config(); 00055 BOOSTEN = 1; 00056 OLED96x96.open(); 00057 OLED96x96.state(Display::DISPLAY_ON); 00058 OLED96x96.fillRect(16,0,111,96,0xff000000);//alpha, BGR 00059 for(int xpos=0;xpos<97;xpos++) 00060 { 00061 for(int ypos=0;ypos<96;ypos++) 00062 { 00063 rgbd = ((Main_screen_bmp[count+3]<<24)|(Main_screen_bmp[count]<<16)|(Main_screen_bmp[count+1]<<8)|(Main_screen_bmp[count+2])); 00064 OLED96x96.drawPixel(ypos+16,xpos,rgbd); 00065 count=count+4; 00066 } 00067 } 00068 Thread::wait(6000); 00069 while(1) 00070 { 00071 accel.acquire_accel_data_g(accel_data); 00072 xposg=(accel_data[1]*(-50.0)); 00073 if (xposg > 36) 00074 xposg = 36; 00075 if (xposg < -36) 00076 xposg = -36; 00077 yposg=accel_data[0]*50.0; 00078 if (yposg > 36) 00079 yposg = 36; 00080 if (yposg < -36) 00081 yposg = -36; 00082 OLED96x96.drawCircle(61-xposg,47+yposg,5,0xff00ff00); 00083 OLED96x96.drawCircle(61-xposg,47+yposg,4,0xff00ff00); 00084 OLED96x96.drawCircle(61-xposg,47+yposg,3,0xff00ff00); 00085 OLED96x96.drawCircle(61-xposg,47+yposg,2,0xff00ff00); 00086 OLED96x96.drawCircle(61-xposg,47+yposg,1,0xff00ff00); 00087 Thread::wait(10); 00088 OLED96x96.drawCircle(61-xposg,47+yposg,5,0xff000000); 00089 OLED96x96.drawCircle(61-xposg,47+yposg,4,0xff000000); 00090 OLED96x96.drawCircle(61-xposg,47+yposg,3,0xff000000); 00091 OLED96x96.drawCircle(61-xposg,47+yposg,2,0xff000000); 00092 OLED96x96.drawCircle(61-xposg,47+yposg,1,0xff000000); 00093 if ((61-xposg == 40+randx) and (47+yposg == 40+randy)) 00094 { 00095 pc.printf("Hit!\r\n"); 00096 led1 = LED_ON; 00097 haptic = 1; 00098 count3 = 0; 00099 } 00100 count3++; 00101 count2++; 00102 if ((count3 > 20)and (haptic == 1)) 00103 { 00104 count2 = 101; 00105 haptic = 0; 00106 } 00107 if (count2 > 100) 00108 { //blank display to avoid image burn 00109 haptic = 0; 00110 count3 = 0; 00111 led1 = LED_OFF; 00112 OLED96x96.fillRect(16,0,111,96,0xff000000);//alpha, BGR 00113 count2 = 0; 00114 randx = rgen()%40; 00115 pc.printf("randx = %d\r\n",randx); 00116 randy = rgen()%40; 00117 pc.printf("randy = %d\r\n",randy); 00118 OLED96x96.drawCircle(40+randx,40+randy,3,0xffff00ff); 00119 OLED96x96.drawCircle(40+randx,40+randy,2,0xffff00ff); 00120 OLED96x96.drawCircle(40+randx,40+randy,1,0xff00ff00); 00121 } 00122 //BOOSTEN = 0; //turn off display after about 1 minute to avoid image burn 00123 } 00124 00125 } 00126
Generated on Sat Jul 23 2022 00:30:12 by
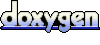