An mbed wrapper around the helium-client to communicate with the Helium Atom
Embed:
(wiki syntax)
Show/hide line numbers
BufferedSerial.h
00001 /** 00002 * \copyright Copyright 2017, Helium Systems, Inc. 00003 * All Rights Reserved. See LICENSE.txt for license information 00004 */ 00005 00006 #include "mbed.h" 00007 #include "RingBuffer.h" 00008 00009 #ifndef BUFFEREDSERIAL_H 00010 #define BUFFEREDSERIAL_H 00011 00012 /** 00013 * \class BufferedSerial 00014 * 00015 * \brief Implements a buffered serial port 00016 * 00017 * This is a simple buffered serial port to work around `readable` 00018 * issues on a number of mbed implementations. Receiving on the serial 00019 * port is buffered through an interrupt handler. 00020 * 00021 * Note that writing is not buffered at this time since writing to the 00022 * serial port at speed had not been an issue so far. 00023 */ 00024 class BufferedSerial : public RawSerial 00025 { 00026 public: 00027 /** Crete a buffered serial port. 00028 * 00029 * @param tx Serial port tx pin to use 00030 * @param rx Serial port rx pin to use 00031 * @param baud The baud rate to use. Defaults to the default for the current 00032 * board 00033 */ 00034 BufferedSerial(PinName tx, 00035 PinName rx, 00036 int baud = MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE); 00037 00038 /** Destructor */ 00039 ~BufferedSerial(); 00040 00041 00042 /** Sets the baud rate for the serial port 00043 * 00044 * @param baud The baud rate to set on the serial port 00045 */ 00046 void baud(int baud); 00047 00048 /** Get a byte from the serial port buffer. 00049 * 00050 * @return a buffered byte or -1 if no bytes are available 00051 */ 00052 int getc(); 00053 00054 /** Write a byte to the serial port 00055 * 00056 * @param ch The byte to write 00057 * @return the byte that was written 00058 */ 00059 int putc(int ch); 00060 00061 /** Check if there are bytes to read. 00062 * 00063 * @return 1 if bytes are available, 0 otherwise 00064 */ 00065 int readable(); 00066 00067 00068 private: 00069 RingBuffer<uint8_t, 16> _rx_buffer; 00070 void _rx_interrupt(); 00071 00072 }; 00073 00074 00075 #endif
Generated on Tue Jul 12 2022 12:08:01 by
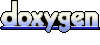