
EPD
Dependencies: mbed-STM32F103C8T6 mbed
main.cpp
00001 #include "stm32f103c8t6.h" 00002 #include "mbed.h" 00003 #include "epd.h" 00004 00005 DigitalOut myled1(LED1); 00006 00007 DigitalOut edp_reset(PB_0); 00008 DigitalOut edp_dataCommand(PB_10); 00009 DigitalOut edp_chipSelect(PA_4); 00010 DigitalIn edp_busy(PB_11); 00011 SPI spi(PA_7,PA_6,PA_5); // mosi, miso, sclk, ss 00012 //DigitalOut eink(PB_0); 00013 /* 00014 EaEpaper epaper(NC, // PWR_CTRL 00015 NC, // BORDER 00016 PB_10, // DISCHARGE 00017 PB_0, // RESET_DISP 00018 PB_11, // BUSY 00019 PA_4, // SSEL 00020 NC, // PWM 00021 PA_7,PA_6,PA_5, // MOSI,MISO,SCLK 00022 "eink"); // SDA,SCL */ 00023 00024 void EPD_SendCommand(unsigned char data) { 00025 edp_chipSelect=0; 00026 edp_dataCommand=0; 00027 spi.write(data); 00028 edp_chipSelect=1; 00029 } 00030 00031 void EPD_SendData(unsigned char data) { 00032 edp_chipSelect=0; 00033 edp_dataCommand=1; 00034 spi.write(data); 00035 edp_chipSelect=1; 00036 } 00037 00038 void EPD_Reset() { 00039 edp_reset=0; 00040 wait_ms(200); 00041 edp_reset=1; 00042 wait_ms(200); 00043 } 00044 00045 void EPD_WaitUntilIdle() { 00046 while(edp_busy == 1) { //0: busy, 1: idle 00047 wait_ms(100); 00048 } 00049 } 00050 00051 /** 00052 * @brief: private function to specify the memory area for data R/W 00053 */ 00054 static void EPD_SetMemoryArea(int x_start, int y_start, int x_end, int y_end) { 00055 EPD_SendCommand(SET_RAM_X_ADDRESS_START_END_POSITION); 00056 /* x point must be the multiple of 8 or the last 3 bits will be ignored */ 00057 EPD_SendData((x_start >> 3) & 0xFF); 00058 EPD_SendData((x_end >> 3) & 0xFF); 00059 EPD_SendCommand(SET_RAM_Y_ADDRESS_START_END_POSITION); 00060 EPD_SendData(y_start & 0xFF); 00061 EPD_SendData((y_start >> 8) & 0xFF); 00062 EPD_SendData(y_end & 0xFF); 00063 EPD_SendData((y_end >> 8) & 0xFF); 00064 } 00065 00066 /** 00067 * @brief: private function to specify the start point for data R/W 00068 */ 00069 static void EPD_SetMemoryPointer(int x, int y) { 00070 EPD_SendCommand(SET_RAM_X_ADDRESS_COUNTER); 00071 /* x point must be the multiple of 8 or the last 3 bits will be ignored */ 00072 EPD_SendData((x >> 3) & 0xFF); 00073 EPD_SendCommand(SET_RAM_Y_ADDRESS_COUNTER); 00074 EPD_SendData(y & 0xFF); 00075 EPD_SendData((y >> 8) & 0xFF); 00076 EPD_WaitUntilIdle(); 00077 } 00078 00079 void EPD_SetLut(const unsigned char* lut) { 00080 EPD_SendCommand(WRITE_LUT_REGISTER); 00081 /* the length of look-up table is 30 bytes */ 00082 for (int i = 0; i < 30; i++) { 00083 EPD_SendData(lut[i]); 00084 } 00085 } 00086 00087 int EPD_Init() { 00088 EPD_Reset(); 00089 EPD_SendCommand(DRIVER_OUTPUT_CONTROL); 00090 EPD_SendData((EPD_HEIGHT - 1) & 0xFF); 00091 EPD_SendData(((EPD_HEIGHT - 1) >> 8) & 0xFF); 00092 EPD_SendData(0x00); // GD = 0; SM = 0; TB = 0; 00093 EPD_SendCommand(BOOSTER_SOFT_START_CONTROL); 00094 EPD_SendData(0xD7); 00095 EPD_SendData(0xD6); 00096 EPD_SendData(0x9D); 00097 EPD_SendCommand(WRITE_VCOM_REGISTER); 00098 EPD_SendData(0xA8); // VCOM 7C 00099 EPD_SendCommand(SET_DUMMY_LINE_PERIOD); 00100 EPD_SendData(0x1A); // 4 dummy lines per gate 00101 EPD_SendCommand(SET_GATE_TIME); 00102 EPD_SendData(0x08); // 2us per line 00103 EPD_SendCommand(DATA_ENTRY_MODE_SETTING); 00104 EPD_SendData(0x03); // X increment; Y increment 00105 EPD_SetLut(lut_full_update); 00106 //EPD_SetLut(lut_partial_update); 00107 /* EPD hardware init end */ 00108 return 0; 00109 } 00110 00111 /** 00112 * @brief: put an image buffer to the frame memory. 00113 * this won't update the display. 00114 */ 00115 void EPD_SetFrameMemory( 00116 const unsigned char* image_buffer, 00117 int x, 00118 int y, 00119 int image_width, 00120 int image_height 00121 ) { 00122 int x_end; 00123 int y_end; 00124 00125 if ( 00126 image_buffer == NULL || 00127 x < 0 || image_width < 0 || 00128 y < 0 || image_height < 0 00129 ) { 00130 return; 00131 } 00132 /* x point must be the multiple of 8 or the last 3 bits will be ignored */ 00133 x &= 0xF8; 00134 image_width &= 0xF8; 00135 if (x + image_width >= EPD_WIDTH) { 00136 x_end = EPD_WIDTH - 1; 00137 } else { 00138 x_end = x + image_width - 1; 00139 } 00140 if (y + image_height >= EPD_HEIGHT) { 00141 y_end = EPD_HEIGHT - 1; 00142 } else { 00143 y_end = y + image_height - 1; 00144 } 00145 EPD_SetMemoryArea(x, y, x_end, y_end); 00146 EPD_SetMemoryPointer(x, y); 00147 EPD_SendCommand(WRITE_RAM); 00148 /* send the image data */ 00149 for (int j = 0; j < y_end - y + 1; j++) { 00150 for (int i = 0; i < (x_end - x + 1) / 8; i++) { 00151 EPD_SendData(image_buffer[i + j * (image_width / 8)]); 00152 } 00153 } 00154 } 00155 00156 /** 00157 * @brief: clear the frame memory with the specified color. 00158 * this won't update the display. 00159 */ 00160 void EPD_ClearFrameMemory(unsigned char color) { 00161 EPD_SetMemoryArea(0, 0, EPD_WIDTH - 1, EPD_HEIGHT - 1); 00162 EPD_SetMemoryPointer(0, 0); 00163 EPD_SendCommand(WRITE_RAM); 00164 /* send the color data */ 00165 for (int i = 0; i < EPD_WIDTH / 8 * EPD_HEIGHT; i++) { 00166 EPD_SendData(color); 00167 } 00168 } 00169 00170 /** 00171 * @brief: update the display 00172 * there are 2 memory areas embedded in the e-paper display 00173 * but once this function is called, 00174 * the the next action of SetFrameMemory or ClearFrame will 00175 * set the other memory area. 00176 */ 00177 void EPD_DisplayFrame() { 00178 EPD_SendCommand(DISPLAY_UPDATE_CONTROL_2); 00179 EPD_SendData(0xC4); 00180 EPD_SendCommand(MASTER_ACTIVATION); 00181 EPD_SendCommand(TERMINATE_FRAME_READ_WRITE); 00182 EPD_WaitUntilIdle(); 00183 } 00184 00185 /** 00186 * @brief: After this command is transmitted, the chip would enter the 00187 * deep-sleep mode to save power. 00188 * The deep sleep mode would return to standby by hardware reset. 00189 * You can use EPD_Init() to awaken 00190 */ 00191 void EPD_Sleep() { 00192 EPD_SendCommand(DEEP_SLEEP_MODE); 00193 EPD_WaitUntilIdle(); 00194 } 00195 00196 void setChar(char chr, int x, int y, unsigned char color){ 00197 unsigned char arr[8]; 00198 memcpy(arr, (unsigned char*)&(FONT8x8[chr - 0x1F][0]), 8); 00199 00200 if(color==0){ 00201 for(int i=0;i<8;i++){ 00202 arr[i]=~arr[i]; 00203 } 00204 } 00205 00206 EPD_SetFrameMemory(arr,x,y,8,8); 00207 } 00208 00209 void setString(char *str, int len, int x, int y, unsigned char color){ 00210 for (int i=0;i<len;i++) { 00211 if(str[i]=='\n' && i<len-1){y+=8;i++;x-=i*8;} 00212 setChar(str[i], x+i*8, y, color); 00213 } 00214 } 00215 00216 int main() { 00217 confSysClock(); 00218 spi.format(8,0); 00219 spi.frequency(4000000); 00220 00221 EPD_Init(); 00222 00223 //EPD_ClearFrameMemory(BACKGROUND_WHITE); 00224 //EPD_DisplayFrame(); 00225 setString("Alexander\nSemion",16,0,0,0); 00226 EPD_DisplayFrame(); 00227 /*unsigned char arr[]={0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00}; 00228 EPD_SetFrameMemory((unsigned char*)&(FONT8x8['S' - 0x1F][0]),0,0,8,8); 00229 EPD_SetFrameMemory((unsigned char*)&(FONT8x8['P' - 0x1F][0]),8,0,8,8); 00230 EPD_SetFrameMemory((unsigned char*)&(FONT8x8['I' - 0x1F][0]),16,0,8,8); 00231 EPD_SetFrameMemory((unsigned char*)&(FONT8x8['N' - 0x1F][0]),24,0,8,8); 00232 EPD_SetFrameMemory((unsigned char*)&(FONT8x8['7' - 0x1F][0]),32,0,8,8); 00233 EPD_SetFrameMemory((unsigned char*)&(FONT8x8['I' - 0x1F][0]),40,0,8,8); 00234 EPD_SetFrameMemory((unsigned char*)&(FONT8x8['O' - 0x1F][0]),48,0,8,8); 00235 EPD_SetFrameMemory((unsigned char*)&(FONT8x8['N' - 0x1F][0]),56,0,8,8); 00236 EPD_DisplayFrame();*/ 00237 /* 00238 while(1){ 00239 myled1 = 1; 00240 wait_ms(1000); 00241 myled1 = 0; 00242 wait_ms(1000); 00243 }*/ 00244 }
Generated on Wed Jul 13 2022 21:41:22 by
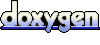