
Presentation code for PROJECT #1 - ES200 Fall 2014
Dependencies: Servo mbed Motor
main.cpp
00001 //MIDN 3/C W. O'Brien, D. Thompson-Sevcik, C. Stabler 00002 //Candy scooper program for Project #1, ES200 Fall 2014 00003 //Modifications: 9 OCT- Initial code 00004 00005 #include "mbed.h" 00006 #include "Motor.h" 00007 #include "Servo.h" 00008 00009 Motor kate(p26, p29, p30); 00010 Servo arm(p21); 00011 Servo scoop(p22); 00012 DigitalIn sw1(p16); //up 00013 DigitalIn sw2(p17); //down 00014 DigitalIn sw3(p18); //dump 00015 DigitalIn sw4(p19); 00016 DigitalIn sw5(p20); 00017 BusOut w(p5, p6, p7, p8, p11); //data bus for LEDs 00018 00019 00020 int main() 00021 { 00022 00023 00024 00025 00026 00027 float armpos; 00028 float servopos; 00029 float mspeed; 00030 w = 16; 00031 while(1) { 00032 00033 if( sw4 == 1 && sw5 == 0 && sw1 == 0 && sw2 == 0 ) {//while switch four is on 00034 mspeed = 0.40; 00035 kate.speed(mspeed); 00036 wait(.05);//structure move for .05 seconds 00037 mspeed = 0.0; 00038 kate.speed(mspeed); 00039 wait(.25);//structure stop for .25 seconds 00040 00041 if(w < 1 || w > 16){ 00042 w=16; } 00043 w = w/2; //moves LED to the right by changing binary value 00044 } else if( sw5 == 1 && sw4 == 0 && sw1 == 0 && sw2 == 0) {//while switch five is on 00045 mspeed = -0.40; 00046 kate.speed(mspeed); 00047 wait(.05);//structure moves in reverse for .05 seconds 00048 mspeed = 0.0; 00049 kate.speed(mspeed); 00050 wait(.25);//structure stops moving for .25 seconds 00051 00052 if(w < 1 || w > 16){ 00053 w=1; 00054 } 00055 w = w*2;//move LED to the left by changing the binary value 00056 } else if (sw3 == 1 && sw2 == 0 && sw1 == 0 && sw4 == 0 && sw5 == 0 ) {//while switch three is on 00057 for (servopos = 0; servopos <= 0.5; servopos += 0.5) {//closes the claw 00058 scoop = servopos; 00059 w = 8; // LED three is on 00060 } 00061 wait (1); 00062 } else if(sw1 == 1 && sw2 == 0 && sw5 == 0 && sw4 == 0 ) {//while switch one is on 00063 armpos += .02; 00064 arm = armpos;//arm position moves up by .02 00065 wait(.05); 00066 w=31; 00067 wait(.05);//lights flicker between on and off 00068 w= 0; 00069 } else if(sw2 == 1 && sw1 == 0 && sw4 == 0 && sw5 == 0) {//while stich two is on 00070 armpos -= .08; 00071 arm = armpos;//arm position moves down by .08 00072 wait(.05); 00073 w=3; 00074 wait(.25);//lights flicker between LED 1 and 2 on and LED 3, 4, and 5 on 00075 w=28; 00076 } else if (sw4 == 0 && sw1 == 0 && sw2 == 0 && sw3 == 0 && sw5 == 0) {//while all switches off 00077 mspeed = 0.0;// no movement of the structure 00078 kate.speed(mspeed); 00079 arm = armpos;// arm stays in the position 00080 wait(.1); 00081 for (servopos = 1.0; servopos >= 0; servopos -=1.0) { 00082 scoop = servopos;//claw opens up 00083 } 00084 } 00085 } 00086 00087 }//end main 00088
Generated on Fri Jul 29 2022 00:09:22 by
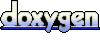