Library release of Simon Ford's GraphicsDisplay Display Library Base Class.
Dependents: ese_project_copy ese_project_share Test_ColorMemLCD rIoTwear_LCD ... more
GraphicsDisplay.h
00001 /* mbed GraphicsDisplay Display Library Base Class 00002 * Copyright (c) 2007-2009 sford 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 * 00005 * A library for providing a common base class for Graphics displays 00006 * To port a new display, derive from this class and implement 00007 * the constructor (setup the display), pixel (put a pixel 00008 * at a location), width and height functions. Everything else 00009 * (locate, printf, putc, cls, window, putp, fill, blit, blitbit) 00010 * will come for free. You can also provide a specialised implementation 00011 * of window and putp to speed up the results 00012 */ 00013 00014 #ifndef MBED_GRAPHICSDISPLAY_H 00015 #define MBED_GRAPHICSDISPLAY_H 00016 00017 #include "TextDisplay.h" 00018 00019 /** A common base class for Graphics displays 00020 */ 00021 class GraphicsDisplay : public TextDisplay { 00022 00023 public: 00024 00025 /** Create a GraphicsDisplay interface 00026 * @param name The name used by the parent class to access the interface 00027 */ 00028 GraphicsDisplay(const char* name); 00029 00030 // functions needing implementation in derived implementation class 00031 // ---------------------------------------------------------------- 00032 /** Draw a pixel in the specified color. 00033 * @note this method must be supported in the derived class. 00034 * @param x is the horizontal offset to this pixel. 00035 * @param y is the vertical offset to this pixel. 00036 * @param colour defines the color for the pixel. 00037 */ 00038 virtual void pixel(int x, int y, int colour) = 0; 00039 00040 /** get the screen width in pixels 00041 * @note this method must be supported in the derived class. 00042 * @returns screen width in pixels. 00043 */ 00044 virtual int width() = 0; 00045 00046 /** get the screen height in pixels 00047 * @note this method must be supported in the derived class. 00048 * @returns screen height in pixels. 00049 */ 00050 virtual int height() = 0; 00051 00052 // functions that come for free, but can be overwritten 00053 // ---------------------------------------------------- 00054 /** Set the window, which controls where items are written to the screen. 00055 * When something hits the window width, it wraps back to the left side 00056 * and down a row. If the initial write is outside the window, it will 00057 * be captured into the window when it crosses a boundary. 00058 * @param x is the left edge in pixels. 00059 * @param y is the top edge in pixels. 00060 * @param w is the window width in pixels. 00061 * @param h is the window height in pixels. 00062 * @note this method may be overridden in a derived class. 00063 */ 00064 virtual void window(int x, int y, int w, int h); 00065 00066 /** Put a single pixel at the current pixel location 00067 * and update the pixel location based on window settings. 00068 * @param colour is the pixel colour. 00069 * @note this method may be overridden in a derived class. 00070 */ 00071 virtual void putp(int colour); 00072 00073 /** clear the entire screen 00074 */ 00075 virtual void cls(); 00076 00077 /** Fill a region using a single colour. 00078 * @param x is the left-edge of the region. 00079 * @param y is the top-edge of the region. 00080 * @param w specifies the width of the region. 00081 * @param h specifies the height of the region. 00082 * @param colour is the fill colour. 00083 * @note this method may be overridden in a derived class. 00084 */ 00085 virtual void fill(int x, int y, int w, int h, int colour); 00086 00087 /** Fill a region using an array. 00088 * @param x is the left-edge of the region. 00089 * @param y is the top-edge of the region. 00090 * @param w specifies the width of the region. 00091 * @param h specifies the height of the region. 00092 * @param colour is a pointer to the array with size = w * h. 00093 * @note this method may be overridden in a derived class. 00094 */ 00095 virtual void blit(int x, int y, int w, int h, const int *colour); 00096 00097 /** Fill a region using a font array. 00098 * @param x is the left-edge of the region. 00099 * @param y is the top-edge of the region. 00100 * @param w specifies the width of the region. 00101 * @param h specifies the height of the region. 00102 * @param colour is a pointer to the font array. 00103 * @note this method may be overridden in a derived class. 00104 */ 00105 virtual void blitbit(int x, int y, int w, int h, const char* colour); 00106 00107 /** Print one character at the specified row, column. 00108 * @param column is the horizontal character position. 00109 * @param row is the vertical character position. 00110 * @param value is the character to print. 00111 * @note this method may be overridden in a derived class. 00112 */ 00113 virtual void character(int column, int row, int value); 00114 00115 /** Get the number of columns based on the currently active font. 00116 * @returns number of columns. 00117 * @note this method may be overridden in a derived class. 00118 */ 00119 virtual int columns(); 00120 00121 /** Get the number of rows based on the currently active font. 00122 * @returns number of rows. 00123 * @note this method may be overridden in a derived class. 00124 */ 00125 virtual int rows(); 00126 00127 protected: 00128 00129 // pixel location 00130 short _x; 00131 short _y; 00132 00133 // window location 00134 short _x1; 00135 short _x2; 00136 short _y1; 00137 short _y2; 00138 00139 }; 00140 00141 #endif
Generated on Fri Jul 15 2022 01:46:38 by
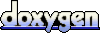