
Show2Me control FW, initial shared version
Dependencies: SDFileSystem_HelloWorld mbed FATFileSystem
Fork of 000_GEO_SHOW2ME_OK_F411RE by
SDFileSystem.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2012 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef MBED_SDFILESYSTEM_H 00023 #define MBED_SDFILESYSTEM_H 00024 00025 #include "mbed.h" 00026 #include "FATFileSystem.h" 00027 #include <stdint.h> 00028 00029 /** Access the filesystem on an SD Card using SPI 00030 * 00031 * @code 00032 * #include "mbed.h" 00033 * #include "SDFileSystem.h" 00034 * 00035 * SDFileSystem sd(p5, p6, p7, p12, "sd"); // mosi, miso, sclk, cs 00036 * 00037 * int main() { 00038 * FILE *fp = fopen("/sd/myfile.txt", "w"); 00039 * fprintf(fp, "Hello World!\n"); 00040 * fclose(fp); 00041 * } 00042 */ 00043 class SDFileSystem : public FATFileSystem { 00044 public: 00045 00046 /** Create the File System for accessing an SD Card using SPI 00047 * 00048 * @param mosi SPI mosi pin connected to SD Card 00049 * @param miso SPI miso pin conencted to SD Card 00050 * @param sclk SPI sclk pin connected to SD Card 00051 * @param cs DigitalOut pin used as SD Card chip select 00052 * @param name The name used to access the virtual filesystem 00053 */ 00054 SDFileSystem(PinName mosi, PinName miso, PinName sclk, PinName cs, const char* name); 00055 virtual int disk_initialize(); 00056 virtual int disk_status(); 00057 virtual int disk_read(uint8_t* buffer, uint32_t block_number, uint32_t count); 00058 virtual int disk_write(const uint8_t* buffer, uint32_t block_number, uint32_t count); 00059 virtual int disk_sync(); 00060 virtual uint32_t disk_sectors(); 00061 00062 protected: 00063 00064 int _cmd(int cmd, int arg); 00065 int _cmdx(int cmd, int arg); 00066 int _cmd8(); 00067 int _cmd58(); 00068 int initialise_card(); 00069 int initialise_card_v1(); 00070 int initialise_card_v2(); 00071 00072 int _read(uint8_t * buffer, uint32_t length); 00073 int _write(const uint8_t *buffer, uint32_t length); 00074 uint32_t _sd_sectors(); 00075 uint32_t _sectors; 00076 00077 void set_init_sck(uint32_t sck) { _init_sck = sck; } 00078 // Note: The highest SPI clock rate is 20 MHz for MMC and 25 MHz for SD 00079 void set_transfer_sck(uint32_t sck) { _transfer_sck = sck; } 00080 uint32_t _init_sck; 00081 uint32_t _transfer_sck; 00082 00083 SPI _spi; 00084 DigitalOut _cs; 00085 int cdv; 00086 int _is_initialized; 00087 }; 00088 00089 #endif
Generated on Tue Jul 26 2022 00:58:23 by
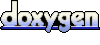