
東北大学学友会準加盟団体 From The Earth の高高度ロケットFTE-06(通称:海豚)にて使用したソフトウェアです.ご自由にお使いください.このプログラムによって生じた損害について当団体は一切責任を負いません.また,各モジュールのライブラリは当団体が作成したものではないので再配布は禁止します.
Dependencies: mbed FATFileSystem
Fork of FTE-06 by
GPS.h
00001 #include "mbed.h" 00002 00003 #ifndef MBED_GPS_H 00004 #define MBED_GPS_H 00005 00006 #define NO_LOCK 1 00007 #define NOT_PARSED 2 00008 #define GGA 3 00009 #define GLL 4 00010 #define RMC 5 00011 #define VTG 6 00012 00013 #define PI (3.141592653589793) 00014 00015 /** A GPS interface for reading from a Globalsat EM-406 GPS Module */ 00016 class GPS { 00017 public: 00018 00019 /** Create the GPS interface, connected to the specified serial port 00020 */ 00021 GPS(PinName tx, PinName rx); 00022 00023 /** Sample the incoming GPS data, returning whether there is a lock 00024 * 00025 * @return 1 if there was a lock when the sample was taken (and therefore .longitude and .latitude are valid), else 0 00026 */ 00027 int sample(); 00028 float get_nmea_longitude(); 00029 float get_nmea_latitude(); 00030 float get_dec_longitude(); 00031 float get_dec_latitude(); 00032 float get_msl_altitude(); 00033 float get_course_t(); 00034 float get_course_m(); 00035 float get_speed_k(); 00036 float get_speed_km(); 00037 int get_satelites(); 00038 float get_altitude_ft(); 00039 00040 // navigational functions 00041 float calc_course_to(float, float); 00042 double calc_dist_to_mi(float, float); 00043 double calc_dist_to_ft(float, float); 00044 double calc_dist_to_km(float, float); 00045 double calc_dist_to_m(float, float); 00046 00047 #ifdef OPEN_LOG 00048 void start_log(void); 00049 void new_file(void); 00050 void stop_log(void); 00051 #endif 00052 00053 private: 00054 float nmea_to_dec(float, char); 00055 float trunc(float v); 00056 void getline(); 00057 void format_for_log(void); 00058 00059 Serial _gps; 00060 char msg[1024]; 00061 char bfr[1030]; 00062 bool is_logging; 00063 #ifdef OPEN_LOG 00064 Logger _openLog; 00065 #endif 00066 // calculated values 00067 float dec_longitude; 00068 float dec_latitude; 00069 float altitude_ft; 00070 00071 // GGA - Global Positioning System Fixed Data 00072 float nmea_longitude; 00073 float nmea_latitude; 00074 float utc_time; 00075 char ns, ew; 00076 int lock; 00077 int satelites; 00078 float hdop; 00079 float msl_altitude; 00080 char msl_units; 00081 00082 // RMC - Recommended Minimmum Specific GNS Data 00083 char rmc_status; 00084 float speed_k; 00085 float course_d; 00086 int date; 00087 00088 // GLL 00089 char gll_status; 00090 00091 // VTG - Course over ground, ground speed 00092 float course_t; // ground speed true 00093 char course_t_unit; 00094 float course_m; // magnetic 00095 char course_m_unit; 00096 char speed_k_unit; 00097 float speed_km; // speek km/hr 00098 char speed_km_unit; 00099 }; 00100 00101 #endif
Generated on Wed Jul 13 2022 10:37:15 by
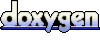