
東北大学学友会準加盟団体 From The Earth の高高度ロケットFTE-06(通称:海豚)にて使用したソフトウェアです.ご自由にお使いください.このプログラムによって生じた損害について当団体は一切責任を負いません.また,各モジュールのライブラリは当団体が作成したものではないので再配布は禁止します.
Dependencies: mbed FATFileSystem
Fork of FTE-06 by
24LCXXX.cpp
00001 /** 00002 ***************************************************************************** 00003 * File Name : _24LCXXX.cpp 00004 * 00005 * Title : I2C EEPROM 24LCXXX Claass Source File 00006 * Revision : 0.1 00007 * Notes : 00008 * Target Board : mbed NXP LPC1768, mbed LPC1114FN28 etc 00009 * Tool Chain : ???? 00010 * 00011 * Revision History: 00012 * When Who Description of change 00013 * ----------- ----------- ----------------------- 00014 * 2012/12/06 Hiroshi M init 00015 ***************************************************************************** 00016 * 00017 * Copyright (C) 2013 Hiroshi M, MIT License 00018 * 00019 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00020 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00021 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00022 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00023 * furnished to do so, subject to the following conditions: 00024 * 00025 * The above copyright notice and this permission notice shall be included in all copies or 00026 * substantial portions of the Software. 00027 * 00028 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00029 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00030 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00031 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00032 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00033 * 00034 **/ 00035 00036 /* Includes ----------------------------------------------------------------- */ 00037 #include "24LCXXX.h" 00038 #include "mbed.h" 00039 00040 /* Private typedef ---------------------------------------------------------- */ 00041 /* Private define ----------------------------------------------------------- */ 00042 /* Private macro ------------------------------------------------------------ */ 00043 /* Private variables -------------------------------------------------------- */ 00044 00045 /* member fanctions --------------------------------------------------------- */ 00046 00047 // Constractor 00048 _24LCXXX::_24LCXXX(I2C *i2c, const int address): 00049 _i2c_address(address<<1), _i2c(i2c), _pc(NULL), _debug(false) 00050 { 00051 } 00052 00053 _24LCXXX::_24LCXXX(I2C *i2c, Serial *pc, const int address): 00054 _i2c_address(address<<1), _i2c(i2c), _pc(pc), _debug(true) 00055 { 00056 } 00057 00058 int _24LCXXX::byte_write( int mem_addr, char data ) 00059 { 00060 int res; 00061 char buf[3]; 00062 int uni_add=_i2c_address; 00063 buf[0] = (0xff00 & mem_addr)>>8; // Write Address High byte set 00064 buf[1] = (0xff & mem_addr); // Write Address Low byte set 00065 buf[2] = data; 00066 //----------------- 00067 if(mem_addr& 0x10000){ 00068 uni_add+=0x02; 00069 00070 } 00071 //------------------- 00072 res = _i2c->write(uni_add, buf, sizeof(buf),false); 00073 if(0) 00074 { 00075 if(res==0) 00076 { 00077 printf("Success! Byte Data Write. \n"); 00078 } 00079 else 00080 { 00081 printf("Failed! Byte Data Write %d.\n", res); 00082 } 00083 } 00084 00085 wait_ms(5); // 5mS 00086 00087 return res; 00088 } 00089 00090 int _24LCXXX::nbyte_write( int mem_addr, void *data, int size ) 00091 { 00092 int i; 00093 int res; 00094 char buf[3]; 00095 char *p; 00096 00097 p = (char *)data; 00098 res = -1; 00099 for ( i = 0; i < size; i++ ) 00100 { 00101 buf[0] = (0xff00 & mem_addr)>>8; // Read Address High byte set 00102 buf[1] = (0x00ff & mem_addr); // Read Address Low byte set 00103 buf[2] = *p++; 00104 00105 res = _i2c->write(_i2c_address, buf, sizeof(buf), false); 00106 if(_debug) 00107 { 00108 if(res==0) 00109 { 00110 _pc->printf("Success! N-Byte Data Write. \n"); 00111 } 00112 else 00113 { 00114 _pc->printf("Failed! N-Byte Data Write %d.\n", res); 00115 } 00116 } 00117 00118 if(res!=0) 00119 { 00120 return res; 00121 } 00122 00123 wait_ms(5); // 5mS 00124 00125 if( ++mem_addr >= MAXADR_24LCXXX ) // Address counter +1 00126 { 00127 return -1; // Address range over 00128 } 00129 } 00130 00131 return res; 00132 } 00133 00134 int _24LCXXX::page_write( int mem_addr, char *data ) 00135 { 00136 int i; 00137 int res; 00138 char buf[PAGE_SIZE_24LCXXX+2]; 00139 00140 buf[0] = (0xff00 & mem_addr)>>8; // Write Address High byte set 00141 buf[1] = (0x00ff & mem_addr); // Write Address Low byte set 00142 00143 for (i=0; i<PAGE_SIZE_24LCXXX; i++) 00144 { 00145 buf[i+2] = data[i]; 00146 } 00147 res = _i2c->write(_i2c_address, buf, sizeof(buf), false); 00148 if(_debug) 00149 { 00150 if(res==0) 00151 { 00152 _pc->printf("Success! Page Data Write. \n"); 00153 } 00154 else 00155 { 00156 _pc->printf("Failed! Page Data Write %d.\n", res); 00157 } 00158 } 00159 00160 return res; 00161 } 00162 00163 00164 int _24LCXXX::nbyte_read( int mem_addr, void *data, int size ) 00165 { 00166 int res; 00167 char buf[2]; 00168 00169 buf[0] = (0xff00 & mem_addr)>>8; // Read Address High byte set 00170 buf[1] = (0x00ff & mem_addr); // Read Address Low byte set 00171 00172 res = _i2c->write(_i2c_address, buf, sizeof(buf), true); 00173 if(_debug) 00174 { 00175 if(res==0) 00176 { 00177 _pc->printf("Success! nbyte read address send. \n"); 00178 } 00179 else 00180 { 00181 _pc->printf("Failed! nbyte read address send %d.\n", res); 00182 } 00183 } 00184 00185 // 00186 res = _i2c->read(_i2c_address, (char *)data, size, false); 00187 if(_debug) 00188 { 00189 if(res==0) 00190 { 00191 _pc->printf("Success! nbyte read address send. \n"); 00192 } 00193 else 00194 { 00195 _pc->printf("Failed! nbyte read address send %d.\n", res); 00196 } 00197 } 00198 00199 return res; 00200 }
Generated on Wed Jul 13 2022 10:37:15 by
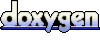