The PWM output by software. Can be output to any pin. It can be used to replace the Pwmout.
Fork of SoftPWM by
SoftPWM.cpp
00001 #include "mbed.h" 00002 #include "InterruptIn.h" 00003 #include "SoftPWM.h" 00004 00005 SoftPWM::SoftPWM(PinName _outpin,bool _positive) : pulse(_outpin) //constructa 00006 { 00007 if ( _positive ) 00008 pulse = 0; 00009 else 00010 pulse = 1; 00011 positive = _positive; 00012 interval = 0.02; 00013 width = 0; 00014 start(); 00015 } 00016 00017 double SoftPWM::read() 00018 { 00019 if ( width <= 0.0 ) return 0.0; 00020 if ( width > 1.0 ) return 1.0; 00021 return width / interval; 00022 } 00023 00024 void SoftPWM::write(double duty) 00025 { 00026 width = interval * duty; 00027 if ( duty <= 0.0 ) width = 0.0; 00028 if ( duty > 1.0 ) width = interval; 00029 } 00030 00031 void SoftPWM::start() 00032 { 00033 _ticker.attach(callback(this,&SoftPWM::TickerInterrapt),interval); 00034 } 00035 00036 void SoftPWM::stop() 00037 { 00038 _ticker.detach(); 00039 if ( positive ) 00040 pulse = 0; 00041 else 00042 pulse = 1; 00043 wait(width); 00044 } 00045 00046 void SoftPWM::period(double _period) 00047 { 00048 interval = _period; 00049 start(); 00050 } 00051 00052 void SoftPWM::period_ms(int _period) 00053 { 00054 period((double)_period / 1000); 00055 start(); 00056 } 00057 00058 void SoftPWM::period_us(int _period) 00059 { 00060 period((double)_period / 1000000); 00061 start(); 00062 } 00063 00064 void SoftPWM::pulsewidth(double _width) 00065 { 00066 width = _width; 00067 if ( width < 0.0 ) width = 0.0; 00068 } 00069 00070 void SoftPWM::pulsewidth_ms(int _width) 00071 { 00072 pulsewidth((double)_width / 1000); 00073 } 00074 00075 void SoftPWM::pulsewidth_us(int _width) 00076 { 00077 pulsewidth((double)_width / 1000000); 00078 } 00079 00080 void SoftPWM::TickerInterrapt() 00081 { 00082 if ( width <= 0 ) return; 00083 _timeout.attach(callback(this,&SoftPWM::end),width); 00084 if ( positive ) 00085 pulse = 1; 00086 else 00087 pulse = 0; 00088 } 00089 00090 void SoftPWM::end() 00091 { 00092 if ( positive ) 00093 pulse = 0; 00094 else 00095 pulse = 1; 00096 // _timeout.detach(); 00097 } 00098 ; 00099
Generated on Tue Jul 26 2022 21:40:50 by
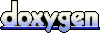