
A basic example of using the mbed NXP LPC1768 Application Board with Exosite.
Dependencies: C12832_lcd EthernetInterface HTTPClient LM75B MMA7660 RGBLed mbed-rtos mbed
Fork of exosite_http_example by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "HTTPClient.h" 00004 #include "C12832_lcd.h" 00005 #include <FileSystemLike.h> 00006 #include <FileHandle.h> 00007 #include "LM75B.h" 00008 00009 /* known issues: 00010 1. CIK becomes corrupted after updating bin files on mbed device -or- resets 00011 2. Does not read led1 yet to control LED1 on/off 00012 00013 */ 00014 const char VENDOR[] = "exosite"; 00015 const char MODEL[] = "mbed_lpc1768_appbrdv1"; 00016 00017 #define WRITE_ALIAS "temp" 00018 #define READ_ALIASES "?screen&led1" 00019 00020 char EXO_CIK_HDR[] = "X-Exosite-CIK: 0000000000000000000000000000000000000000\r\n"; 00021 const char EXO_CIK_HDR_FMT[] = "X-Exosite-CIK: %s\r\n"; 00022 char EXO_ACCEPT_HDR[] = "Accept: application/x-www-form-urlencoded; charset=utf-8\r\n"; 00023 char EXO_CONTYP_HDR[] = "Content-Type: application/x-www-form-urlencoded; charset=utf-8\r\n"; 00024 const char EXO_URI[] = "https://m2.exosite.com:443/onep:v1/stack/alias" READ_ALIASES; 00025 // NOTE: Need to specify port due to parsing bug in lib on "onep:v1" 00026 const char EXO_ACTIVATE_URI[] = "https://m2.exosite.com/provision/activate"; 00027 00028 #define TIMEOUT 2000 00029 #define BUFFSIZE 1024 00030 00031 AnalogIn aPot1(p19); 00032 Timer send_timer, connection_timer, run_time; 00033 00034 EthernetInterface eth; 00035 HTTPClient http; 00036 00037 LocalFileSystem localfs("local"); 00038 00039 static C12832_LCD lcd; 00040 LM75B tmp(p28,p27); 00041 00042 AnalogIn Pot1(p19); 00043 AnalogIn Pot2(p20); 00044 DigitalOut Led1Out(LED1); 00045 00046 int main() 00047 { 00048 int uptime = 0; 00049 00050 run_time.start(); 00051 send_timer.start(); 00052 00053 printf("\r\nStart\r\n"); 00054 00055 eth.init(); //Use DHCP 00056 00057 lcd.printf("Exosite HTTP Cloud Demo"); 00058 lcd.locate(0,11); 00059 lcd.printf("MAC: %s", eth.getMACAddress()); 00060 lcd.locate(0,22); 00061 lcd.printf("IP: Requesting From DHCP..."); 00062 00063 eth.connect(); 00064 00065 printf("IP Address is %s\r\n", eth.getIPAddress()); 00066 printf("MAC Address is %s\r\n", eth.getMACAddress()); 00067 00068 lcd.locate(0,22); 00069 lcd.printf("IP: %s ", eth.getIPAddress()); 00070 00071 printf("[%f] Running...\r\n", run_time.read()); 00072 00073 printf("[%f] Attempting Activation\r\n", run_time.read()); 00074 { 00075 int ret; 00076 char incomming_buffer[BUFFSIZE]; 00077 HTTPText hti = HTTPText(incomming_buffer, BUFFSIZE); 00078 HTTPMap hto = HTTPMap(); 00079 hto.put("vendor", VENDOR); 00080 hto.put("model", MODEL); 00081 hto.put("sn", eth.getMACAddress()); 00082 if ((ret = http.post(EXO_ACTIVATE_URI, hto, &hti)) == 0) { 00083 printf("Success, Got: %s\n", incomming_buffer); 00084 lcd.locate(0,0); 00085 sprintf(EXO_CIK_HDR, EXO_CIK_HDR_FMT, incomming_buffer); 00086 FILE *fp = fopen("/local/cik.txt", "w"); 00087 fprintf(fp, "%s", incomming_buffer); 00088 fclose(fp); 00089 } else if (ret == 0) { 00090 printf("Couldn't Connect to Exosite, Check Network\r\n"); 00091 lcd.locate(0,0); 00092 lcd.printf("Couldn't Connect to Exosite"); 00093 lcd.locate(0,11); 00094 lcd.printf("Check Network"); 00095 } else { 00096 printf("Error %d\r\n", http.getHTTPResponseCode()); 00097 lcd.locate(0,0); 00098 lcd.printf("Act Error (%d)", http.getHTTPResponseCode()); 00099 FILE *fp = fopen("/local/cik.txt", "r"); 00100 if (fp !=0 && fread(incomming_buffer, 1, 40, fp) == 40) { 00101 sprintf(EXO_CIK_HDR, EXO_CIK_HDR_FMT, incomming_buffer); 00102 printf("Found cik in nv: %s\r\n", incomming_buffer); 00103 } else if (ret != 0) { 00104 printf("No CIK, Please Re-Enable Device for Activation in Portals\r\n"); 00105 lcd.locate(0,0); 00106 lcd.printf("No CIK, Re-Enable in Portals"); 00107 lcd.locate(0,22); 00108 lcd.printf("Then Hard-Reset Board"); 00109 while(1); 00110 } 00111 00112 if (fp != 0) 00113 fclose(fp); 00114 } 00115 } 00116 00117 while (1) { 00118 int ret; 00119 char *key, *value; 00120 char incomming_buffer[BUFFSIZE]; 00121 char scratch[32]; 00122 char tmp_str[8]={0}; 00123 char uptime_str[8]={0}; 00124 char pot1_str[8]={0}; 00125 char pot2_str[8]={0}; 00126 00127 HTTPMap read_map = HTTPMap(incomming_buffer, BUFFSIZE); 00128 HTTPMap write_map = HTTPMap(); 00129 00130 if(tmp.open()) 00131 { 00132 snprintf(tmp_str, 16, "%.2f", (float)tmp); 00133 printf("[%f] temp: %s C\r\n", run_time.read(), tmp_str); 00134 write_map.put("tempc", tmp_str); 00135 } 00136 else { 00137 error("Temp Sensor not detected!\n"); 00138 } 00139 00140 snprintf(pot1_str, 16, "%3.3f", Pot1.read()*100.0f); 00141 printf("[%f] Pot1: %s %%\r\n", run_time.read(), pot1_str); 00142 write_map.put("pot1", pot1_str); 00143 00144 snprintf(pot2_str, 16, "%3.3f", Pot2.read()*100.0f); 00145 printf("[%f] Pot2: %s %%\r\n", run_time.read(), pot2_str); 00146 write_map.put("pot2", pot2_str); 00147 00148 /*if(Pot1 > 0.3f) { 00149 Led1Out = 1; 00150 } else { 00151 Led1Out = 0; 00152 }*/ 00153 00154 snprintf(uptime_str, 16, "%d", uptime); 00155 printf("[%f] Uptimer Counter: %s\r\n", run_time.read(), uptime_str); 00156 write_map.put("uptime", uptime_str); 00157 00158 http.setHeader(0,EXO_CIK_HDR); 00159 http.setHeader(1,EXO_ACCEPT_HDR); 00160 //http.setHeader(2,EXO_CONTYP_HDR); 00161 00162 printf("[%f] Making Request...\r\n", run_time.read()); 00163 connection_timer.reset(); 00164 connection_timer.start(); 00165 00166 // make request 00167 ret = http.post(EXO_URI, write_map, &read_map); 00168 connection_timer.stop(); 00169 printf("[%f] Done! Status: %d, %d\r\n", run_time.read(), ret, http.getHTTPResponseCode()); 00170 lcd.locate(0,0); 00171 lcd.printf(" "); 00172 if (!ret) { 00173 // write 'screen' dp to screen 00174 lcd.locate(0,0); 00175 if (read_map.get("screen", scratch, 32)) { 00176 lcd.printf(scratch); 00177 } else { 00178 lcd.printf("couldn't read 'screen' dp"); 00179 } 00180 00181 // list returned dataports 00182 while(read_map.pop(key, value)) { 00183 printf(" %s: %s\r\n", key, value); 00184 } 00185 } else { 00186 lcd.locate(0,0); 00187 lcd.printf("Error (%d, %d)", ret, http.getHTTPResponseCode()); 00188 } 00189 00190 printf("[%f] Completed in %f seconds.\r\n", run_time.read(), connection_timer.read()); 00191 uptime++; 00192 wait(5); 00193 } 00194 00195 }
Generated on Mon Jul 18 2022 16:39:46 by
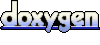