This is the Interface library for WIZnet W5500 chip which forked of EthernetInterfaceW5500, WIZnetInterface and WIZ550ioInterface. This library has simple name as "W5500Interface". and can be used for Wiz550io users also.
Dependents: EvrythngApi Websocket_Ethernet_HelloWorld_W5500 Websocket_Ethernet_W5500 CurrentWeatherData_W5500 ... more
TCPSocketConnection.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #include "TCPSocketConnection.h" 00019 #include <cstring> 00020 00021 using std::memset; 00022 using std::memcpy; 00023 00024 // not a big code. 00025 // refer from EthernetInterface by mbed official driver 00026 TCPSocketConnection::TCPSocketConnection() : 00027 _is_connected(false) 00028 { 00029 } 00030 00031 int TCPSocketConnection::connect(const char* host, const int port) 00032 { 00033 if (_sock_fd < 0) { 00034 _sock_fd = eth->new_socket(); 00035 if (_sock_fd < 0) { 00036 return -1; 00037 } 00038 } 00039 if (set_address(host, port) != 0) { 00040 return -1; 00041 } 00042 if (!eth->connect(_sock_fd, get_address(), port)) { 00043 return -1; 00044 } 00045 set_blocking(false); 00046 // add code refer from EthernetInterface. 00047 _is_connected = true; 00048 00049 return 0; 00050 } 00051 00052 bool TCPSocketConnection::is_connected(void) 00053 { 00054 // force update recent state. 00055 _is_connected = eth->is_connected(_sock_fd); 00056 return _is_connected; 00057 } 00058 00059 int TCPSocketConnection::send(char* data, int length) 00060 { 00061 // add to cover exception. 00062 if ((_sock_fd < 0) || !_is_connected) 00063 return -1; 00064 00065 int size = eth->wait_writeable(_sock_fd, _blocking ? -1 : _timeout); 00066 if (size < 0) { 00067 return -1; 00068 } 00069 if (size > length) { 00070 size = length; 00071 } 00072 return eth->send(_sock_fd, data, size); 00073 } 00074 00075 // -1 if unsuccessful, else number of bytes written 00076 int TCPSocketConnection::send_all(char* data, int length) 00077 { 00078 int writtenLen = 0; 00079 while (writtenLen < length) { 00080 int size = eth->wait_writeable(_sock_fd, _blocking ? -1 : _timeout); 00081 if (size < 0) { 00082 return -1; 00083 } 00084 if (size > (length-writtenLen)) { 00085 size = (length-writtenLen); 00086 } 00087 int ret = eth->send(_sock_fd, data + writtenLen, size); 00088 if (ret < 0) { 00089 return -1; 00090 } 00091 writtenLen += ret; 00092 } 00093 return writtenLen; 00094 } 00095 00096 // -1 if unsuccessful, else number of bytes received 00097 int TCPSocketConnection::receive(char* data, int length) 00098 { 00099 // add to cover exception. 00100 if ((_sock_fd < 0) || !_is_connected) 00101 return -1; 00102 00103 int size = eth->wait_readable(_sock_fd, _blocking ? -1 : _timeout); 00104 if (size < 0) { 00105 return -1; 00106 } 00107 if (size > length) { 00108 size = length; 00109 } 00110 return eth->recv(_sock_fd, data, size); 00111 } 00112 00113 // -1 if unsuccessful, else number of bytes received 00114 int TCPSocketConnection::receive_all(char* data, int length) 00115 { 00116 int readLen = 0; 00117 while (readLen < length) { 00118 int size = eth->wait_readable(_sock_fd, _blocking ? -1 :_timeout); 00119 if (size <= 0) { 00120 break; 00121 } 00122 if (size > (length - readLen)) { 00123 size = length - readLen; 00124 } 00125 int ret = eth->recv(_sock_fd, data + readLen, size); 00126 if (ret < 0) { 00127 return -1; 00128 } 00129 readLen += ret; 00130 } 00131 return readLen; 00132 }
Generated on Thu Jul 14 2022 02:02:03 by
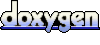