voor willem test
Dependencies: 4DGL MODSERIAL mbed mbos
screen_handler.cpp
00001 #include "mbed.h" 00002 #include "TFT_4DGL.h" 00003 #include "display.h" 00004 #include "FS_datastructures.h" 00005 #include "MODSERIAL.h" 00006 00007 00008 #include <string> 00009 using namespace std; 00010 00011 extern MODSERIAL SERIAL_DEBUG; // <----- debug only !! 00012 00013 extern int FSdata_received_flag; //Prototype of data flag (declared in main.cpp) 00014 extern TFT_4DGL display; //Prototype of Display Driver (declared in display.cpp) 00015 00016 //Prototype of indicators (declared in keyboard.cpp) 00017 extern DigitalOut EXEC; 00018 extern DigitalOut FAIL; 00019 extern DigitalOut DSPY; 00020 extern DigitalOut MSG; 00021 extern DigitalOut OFST; 00022 00023 extern void CDU_SET_BGL_INTENSITY( int nVal ); //Prototype of function controlling CDU backlight (declared in keyboard.cpp) 00024 extern int nFontSize( int nfont_number ); //Prototype of function for fontselection (declared in display.cpp) 00025 extern int nFontWidth (int nfont_number ); //Prototype of function to retrieve font width (declared in display.cpp) 00026 extern int nLine2Pixel( int nLine ); //Prototype of function to calculate vertical pixelposition from line number (declared in display.cpp) 00027 extern int LeftOrRight( int nTextLine, string cString, int nChars, int nCharWidth ); //declared in display.cpp 00028 extern int cRGB( char cRED, char cGREEN, char cBLUE ); //Prototype of function for assembly color word (declared in display.cpp) 00029 00030 // FS_data_update_ID: 00031 // These global flags indicate what data has been updated. 00032 // Should be tested when FS_DATA_EVENT occurs. 00033 extern int Background_Col_Update; // 1 when color was updated, must be reset to 0 when data has been read 00034 extern int CDU_Status_Update ; // 1 when status was updated, must be reset to 0 when data has been read 00035 extern int DO_CLR_SCREEN ; // 1 when screen should be cleared, must be reset to 0 when done 00036 extern int Text_Line_Update ; // equal to line number whose text was updated, must be reset to 0 when text has been read 00037 extern int Key_Maintext_Update ; // equal to keynumber whose main text line was updated, must be reset to -1 (!)when text has been read 00038 extern int Key_Subtext_Update ; // equal to keynumber whose sub text line was updated, must be reset to -1 (!) when text has been read 00039 00040 // Common flag to signal that one or more updates were performed: 00041 //int FSdata_received_flag = false; // : true when one or more FS-to-CDU data structures were updated 00042 00043 // -------------------------------------------------------------------------------------------------- 00044 00045 void CDU_DSP_CSS() 00046 /*Check flags to see if action is required 00047 Background_Col_Update; // : 1 when color was updated, must be reset to 0 when data has been read 00048 CDU_Status_Update ; // : 1 when status was updated, must be reset to 0 when data has been read 00049 DO_CLR_SCREEN ; // : 1 when screen should be cleared, must be reset to 0 when done 00050 Text_Line_Update ; // : equal to line number whose text was updated, must be reset to 0 when text has been read 00051 Key_Maintext_Update ; // : equal to keynumber whose main text line was updated, must be reset to -1 (!)when text has been read 00052 Key_Subtext_Update ; // : equal to keynumber whose sub text line was updated, must be reset to -1 (!) when text has been read 00053 */ 00054 00055 { 00056 int nLine = 1; //default value 00057 //check common flag 00058 00059 if ( Background_Col_Update == 1) 00060 { 00061 display.background_color( cRGB( BACKGROUND_COL.BG_RED, BACKGROUND_COL.BG_GREEN, BACKGROUND_COL.BG_BLUE ) ); 00062 Background_Col_Update = 0; 00063 } 00064 00065 if ( Key_Maintext_Update > -1 ) 00066 //Key_Maintext_Update contains the line number 00-49 is LSK text, 50-99 is RSK text 00067 //Currently used: 00068 //00-14 left side of screen --> left adjust, horizontal position = 0 00069 //50-64 right side of screen --> right adjust, horizontal position calculated with righttext() (declared in display.cpp) 00070 00071 //00 = LSK1 50 = RSK1 Print on LINE 3 00072 //01 = LSK2 51 = RSK2 Print on LINE 5 00073 //02 = LSK3 52 = RSK3 Print on LINE 7 00074 //03 = LSK4 53 = RSK4 Print on LINE 9 00075 //04 = LSK5 54 = RSK5 Print on LINE 11 00076 //05 = LSK6 55 = RSK6 Print on LINE 13 00077 { 00078 switch ( Key_Maintext_Update ) 00079 { 00080 case ( 00 ): nLine = 3; break; 00081 case ( 50 ): nLine = 3; break; 00082 case ( 01 ): nLine = 5; break; 00083 case ( 51 ): nLine = 5; break; 00084 case ( 02 ): nLine = 7; break; 00085 case ( 52 ): nLine = 7; break; 00086 case ( 03 ): nLine = 9; break; 00087 case ( 53 ): nLine = 9; break; 00088 case ( 04 ): nLine = 11; break; 00089 case ( 54 ): nLine = 11; break; 00090 case ( 05 ): nLine = 13; break; 00091 case ( 55 ): nLine = 13; break; 00092 } 00093 00094 SERIAL_DEBUG.printf("Key MAINTEXT is : %s\r\n",SELKEY_MAINTEXT[Key_Maintext_Update].text ); // show text 00095 00096 //display.graphic_string(char *s, int x, int y, char font, int color, char width multiplier, char height multiplier) 00097 /* 00098 display.graphic_string( SELKEY_MAINTEXT[Key_Maintext_Update].text , //Text to display 00099 LeftOrRight( Key_Maintext_Update, SELKEY_MAINTEXT[Key_Maintext_Update].text,24,24*nFontWidth( SELKEY_MAINTEXT[Key_Maintext_Update].font_size )) , //Horizontal position 00100 nLine2Pixel( nLine ), //Vertical position 00101 nFontSize( SELKEY_MAINTEXT[Key_Maintext_Update].font_size ), //Font 00102 cRGB( SELKEY_MAINTEXT[Key_Maintext_Update].text_RED ,SELKEY_MAINTEXT[Key_Maintext_Update].text_GREEN ,SELKEY_MAINTEXT[Key_Maintext_Update].text_BLUE ), 00103 1, 1 ); 00104 */ 00105 display.graphic_string( SELKEY_MAINTEXT[Key_Maintext_Update].text , //Text to display 00106 12*24 , //Horizontal position 00107 nLine2Pixel( nLine ), //Vertical position 00108 nFontSize( SELKEY_MAINTEXT[Key_Maintext_Update].font_size ), //Font 00109 cRGB( SELKEY_MAINTEXT[Key_Maintext_Update].text_RED ,SELKEY_MAINTEXT[Key_Maintext_Update].text_GREEN ,SELKEY_MAINTEXT[Key_Maintext_Update].text_BLUE ), 00110 1, 1 ); 00111 00112 00113 Key_Maintext_Update = -1; 00114 } 00115 00116 if ( Key_Subtext_Update > -1 ) 00117 //Key Subtext_Update contains the line number 00-49 is LSK subtext, 50-99 is RSK subtext 00118 //Currently used: 00119 //00-14 left side of screen --> left adjust, horizontal position = 0 00120 //50-64 right side of screen --> right adjust, horizontal position calculated with righttext() (declared in display.cpp) 00121 //00 = LSK1 50 = RSK1 Print on LINE 2 00122 //01 = LSK2 51 = RSK2 Print on LINE 4 00123 //02 = LSK3 52 = RSK3 Print on LINE 6 00124 //03 = LSK4 53 = RSK4 Print on LINE 8 00125 //04 = LSK5 54 = RSK5 Print on LINE 10 00126 //05 = LSK6 55 = RSK6 Print on LINE 12 00127 { 00128 switch ( Key_Subtext_Update ) 00129 { 00130 case ( 00 ): nLine = 2; break; 00131 case ( 50 ): nLine = 2; break; 00132 case ( 01 ): nLine = 4; break; 00133 case ( 51 ): nLine = 4; break; 00134 case ( 02 ): nLine = 6; break; 00135 case ( 52 ): nLine = 6; break; 00136 case ( 03 ): nLine = 8; break; 00137 case ( 53 ): nLine = 8; break; 00138 case ( 04 ): nLine = 10; break; 00139 case ( 54 ): nLine = 10; break; 00140 case ( 05 ): nLine = 12; break; 00141 case ( 55 ): nLine = 12; break; 00142 } 00143 //display.graphic_string(char *s, int x, int y, char font, int color, char width multiplier, char height multiplier) 00144 display.graphic_string( SELKEY_SUBTEXT[Key_Subtext_Update].text , //Text to display 00145 LeftOrRight( Key_Subtext_Update, SELKEY_SUBTEXT[Key_Subtext_Update].text,12,48*nFontWidth( SELKEY_SUBTEXT[Key_Subtext_Update].font_size )) , //Horizontal position 00146 nLine2Pixel( nLine ), //Vertical position 00147 nFontSize( SELKEY_SUBTEXT[Key_Subtext_Update].font_size ), //Font 00148 cRGB( SELKEY_SUBTEXT[Key_Subtext_Update].text_RED ,SELKEY_SUBTEXT[Key_Subtext_Update].text_GREEN ,SELKEY_SUBTEXT[Key_Subtext_Update].text_BLUE ), 00149 1, 1 ); //multiplier always on 1 00150 Key_Subtext_Update = -1; 00151 } 00152 00153 if ( Text_Line_Update > 0) 00154 { 00155 //Text_Line_Update contains the the line number to write 00156 display.graphic_string( TEXTLINE[Text_Line_Update].text , //Text to display 00157 0, //Horizontal position always 0 00158 nLine2Pixel( Text_Line_Update ), //Vertical position 00159 nFontSize( TEXTLINE[Text_Line_Update].font_size ), //Font 00160 cRGB( TEXTLINE[Text_Line_Update].text_RED ,TEXTLINE[Text_Line_Update].text_GREEN ,TEXTLINE[Text_Line_Update].text_BLUE ), 00161 1, 1 ); //multiplier always on 1 00162 Text_Line_Update = 0; 00163 } 00164 00165 if ( CDU_Status_Update == 1 ) 00166 { 00167 /* 00168 CDU_STATUS.stby_mode; // : 0 = operational mode, 1 = standby mode 00169 */ if ( CDU_STATUS.backlight ) // Backlight control 00170 { 00171 CDU_SET_BGL_INTENSITY( 255 ); 00172 } 00173 else 00174 { 00175 CDU_SET_BGL_INTENSITY( 0 ); 00176 } 00177 // Set CDU indicators 00178 MSG = CDU_STATUS.msg_indicator; 00179 EXEC = CDU_STATUS.exec_indicator; 00180 FAIL = CDU_STATUS.fail_indicator; 00181 DSPY = CDU_STATUS.dspy_indicator; 00182 OFST = CDU_STATUS.ofst_indicator; 00183 00184 CDU_Status_Update =0; 00185 } 00186 if ( DO_CLR_SCREEN ) 00187 { 00188 display.cls(); 00189 DO_CLR_SCREEN =0; 00190 } 00191 }
Generated on Tue Jul 12 2022 11:39:27 by
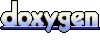