voor willem test
Dependencies: 4DGL MODSERIAL mbed mbos
display.cpp
00001 //Display control functions -- 00002 00003 #include "mbed.h" 00004 #include "TFT_4DGL.h" 00005 #include "display.h" 00006 #include <string> 00007 using namespace std; 00008 00009 DigitalOut VGA_SOURCE( p7 ); //control line for video switch between INT and EXT video 00010 DigitalOut VGA_SELECT( p8 ); //control line to select/deselect video switch 00011 00012 /* 00013 ===================================================== 00014 SGC (Serial Graphics Controller) PLATFORM OUTPUT FILE 00015 ===================================================== 00016 00017 ******************************************************* 00018 * Must set 'New image format' for usage on Picaso SGC * 00019 * Data: * 00020 * 0x59, 0x06, 0x00 * 00021 * 4DSL command: * 00022 * Control(6,0) * 00023 ******************************************************* 00024 00025 --------------------------------------------------------------------------------------- 00026 File "logo_flyengravity.jpg" (logo_flyengravity.jpg) 00027 Sector Address 0x000000 00028 X = 0 Y = 135 Width = 640 Height = 215 Bits = 16 00029 00030 Display Image from Memory Card (Serial Command): 00031 Syntax: 00032 @, I, x, y, SectorAdd(hi), SectorAdd(mid), SectorAdd(lo) 00033 Picaso Data: 00034 0x40, 0x49, 0x00, 0x00, 0x00, 0x87, 0x00, 0x00, 0x00 00035 4DSL command: 00036 NewUSDImage(0, 135, 0x000000) 00037 00038 --------------------------------------------------------------------------------------- 00039 File "Testscreen.png" (Testscreen.png) 00040 Sector Address 0x00021A 00041 X = 0 Y = 0 Width = 640 Height = 480 Bits = 16 00042 00043 Display Image from Memory Card (Serial Command): 00044 Syntax: 00045 @, I, x, y, SectorAdd(hi), SectorAdd(mid), SectorAdd(lo) 00046 Picaso Data: 00047 0x40, 0x49, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x1A 00048 4DSL command: 00049 NewUSDImage(0, 0, 0x00021A) 00050 00051 */ 00052 00053 /*Display Commands 00054 00055 $PCDUCLS Erase to active background colour (default black) or white 00056 =================================================================== 00057 Syntax: $PCDUCLS, <mode>*<checksum>CRLF 00058 <mode>=0 : Clear screen to current background colour (default=black) 00059 <mode>=1 : Clear screen to white 00060 00061 $PCDUSBC Set Background Color 00062 ==================================================================== 00063 Syntax: $PCDUSBC,<Rcolour>,<Gcolour>,<Bcolour>*<checksum>CRLF 00064 < Rcolour > : RED (000‐255) 00065 < Gcolour > : GREEN (000‐255) 00066 < Bcolour > : BLUE (000‐255) 00067 Set background colour to RGB as defined in the three data fields. 00068 00069 $PCDUWTX WTX=WRITE TEXT Write text on any X,Y position on the screen. 00070 ===================================================================== 00071 Syntax: $PCDUWTX,<Fsize>,<Fstyle>,<Rcolour>,<Gcolour>,<Bcolour>,<Text><Col>,<Row>*<checksum>CRLF 00072 <Fsize> : Font size (F0..F9). Currently F0 and F1 are implemented. 00073 <Fstyle> : Font style (S=Standard, B=Bold, I=Italic, U=Underline, N=Negative) 00074 < Rcolour > : RED (000‐255) 00075 < Gcolour > : GREEN (000‐255) 00076 < Bcolour > : BLUE (000‐255) 00077 <Text> : Any printable ASCII character except the * character as this is used as the text field delimiter. 00078 Maximum length is 24 characters, minimum length is 1 character for font F0. 00079 Maximum length is 48 characters, minumum length is 1 character for font F1. 00080 <Col> : Horizontal position of the first character (1..24) 00081 <Row> : Vertical position of the line (1..14) 00082 00083 $PCDUETX ETX=ERASE TEXT 00084 ============================================================= 00085 Syntax: $PCDUETX, <Col>,<Row>,<n>*<checksum>CRLF 00086 <Col> : Horizontal position of the first character (1..24 for font F0 or 1..48 for font F1) 00087 <Row> : Vertical position of the line (1‐14) 00088 <n> : number of characters to be erased (1..24 for font F0 or 1..48 for font F1) 00089 00090 $PCDUKTX Write text attached to a Select Key 00091 =========================================================== 00092 Syntax: $PCDUKTX,<KeyID>,<Texttype>,<Fsize>,<Fstyle>,<Rcolour>,<Gcolour>,<Bcolour>,<Text>*< checksum>CRLF 00093 <KeyID> : Numbering is 00 – 49 for left keys and 50 – 99 for right keys. Top keys are 00 and 50. 00094 <Texttype> : M or S, meaning Main text or Subtext. 00095 <Fsize> : Font size (F0..F9). Currently F0 and F1 are implemented. 00096 <Fstyle> : Font style (S=Standard, B=Bold, I=Italic, U=Underline, N=Negative) 00097 < Rcolour > : RED (000‐255) 00098 < Gcolour > : GREEN (000‐255) 00099 < Bcolour > : BLUE (000‐255) 00100 <Text> : Any printable ASCII character within the character set.except the * character as this is used as the text field delimiter. 00101 Maximum length is 24 characters, minimum length is 1 character for font F0 00102 Maximum length is 48 characters, minumum length is 1 character for font F1. 00103 */ 00104 00105 //Control lines for VGA driver board 00106 TFT_4DGL display(p13,p14,p15); // serial tx, serial rx, reset pin 00107 00108 //Character & String functions 00109 00110 char* str2char( string cString ) //convert a string to a character array 00111 { 00112 int nStrLen=cString.size(); 00113 std::string cInput( cString ); 00114 char* cText = new char[nStrLen+1]; 00115 strncpy(cText, cInput.c_str(), nStrLen); 00116 cText[nStrLen] = '\0'; 00117 return cText; 00118 } 00119 00120 int centertext( string cString, int nChars, int nCharWidth ) 00121 //calculates the startposition on the screen to center the text 00122 //needs the actual string (cString), screenwidth in characters (nChars) and the character width (nCharWidth) 00123 { 00124 int nStart; 00125 nStart = nCharWidth*( nChars/2-( cString.size()/2 )); 00126 return nStart; 00127 } 00128 00129 int righttext( string cString, int nChars, int nCharWidth ) 00130 //calculates the startposition on the screen to right-align the text 00131 //needs the actual string (cString), screenwidth in characters (nChars) and the character width (nCharWidth) 00132 { 00133 int nStart; 00134 nStart = nCharWidth*( nChars - cString.size()); 00135 return nStart; 00136 } 00137 00138 int nFontSize( int nfont_number ) 00139 { 00140 int nFont = 0; 00141 switch ( nfont_number ) 00142 { 00143 case 0: 00144 { 00145 nFont = FONT_12X34; 00146 break; 00147 } 00148 case 1: 00149 { 00150 nFont = FONT_24X34; 00151 break; 00152 } 00153 } 00154 return ( nFont ); 00155 } 00156 00157 int nFontWidth (int nfont_number ) 00158 { 00159 int nFont = 12; 00160 switch ( nfont_number ) 00161 { 00162 case 0: 00163 { 00164 nFont = 12; 00165 break; 00166 } 00167 case 1: 00168 { 00169 nFont = 24; 00170 break; 00171 } 00172 } 00173 return ( nFont ); 00174 } 00175 00176 unsigned int cRGB( char cRED, char cGREEN, char cBLUE ) 00177 { 00178 //assemble separate colors into 1 integer value as 0xRRGGBB 00179 //Display driver requires this format 00180 unsigned int RGB = cBLUE + 256*cGREEN + 65536*cRED; 00181 return ( RGB ); 00182 } 00183 00184 int LeftOrRight( int nTextLine, string cString, int nChars, int nCharWidth ) 00185 { 00186 //decide to print data left aligned or right aligned 00187 //00 - 49 is left side of screen 00188 //50 - 99 is right side of screen 00189 int nHorPos = 0; 00190 // nChars is number of characters on this line (24 or 48) 00191 // nCharWidth is the character width in pixels 00192 00193 if ( nTextLine < 50 ) 00194 // Left side adjust 00195 { 00196 nHorPos = 0; 00197 } 00198 else 00199 // Right side adjust 00200 { 00201 nHorPos = righttext( cString, nChars, nCharWidth ); 00202 } 00203 return ( nHorPos ); 00204 } 00205 00206 int nLine2Pixel( int nLine ) 00207 { 00208 //calculate vertical pixelposition from linenumber 00209 int nPixel = 0; 00210 switch ( nLine ) 00211 { 00212 case 1: 00213 { 00214 nPixel = LINE1; 00215 break; 00216 } 00217 case 2: 00218 { 00219 nPixel = LINE2; 00220 break; 00221 } 00222 00223 case 3: 00224 { 00225 nPixel = LINE3; 00226 break; 00227 } 00228 00229 case 4: 00230 { 00231 nPixel = LINE4; 00232 break; 00233 } 00234 00235 case 5: 00236 { 00237 nPixel = LINE5; 00238 break; 00239 } 00240 00241 case 6: 00242 { 00243 nPixel = LINE6; 00244 break; 00245 } 00246 00247 case 7: 00248 { 00249 nPixel = LINE7; 00250 break; 00251 } 00252 00253 case 8: 00254 { 00255 nPixel = LINE8; 00256 break; 00257 } 00258 00259 case 9: 00260 { 00261 nPixel = LINE9; 00262 break; 00263 } 00264 00265 case 10: 00266 { 00267 nPixel = LINE10; 00268 break; 00269 } 00270 00271 case 11: 00272 { 00273 nPixel = LINE11; 00274 break; 00275 } 00276 00277 case 12: 00278 { 00279 nPixel = LINE12; 00280 break; 00281 } 00282 00283 case 13: 00284 { 00285 nPixel = LINE13; 00286 break; 00287 } 00288 00289 case 14: 00290 { 00291 nPixel = LINE14; 00292 break; 00293 } 00294 00295 } 00296 return ( nPixel ) ; 00297 } 00298 00299 void VGA_SIGNAL( int Source, int On_Off) 00300 { 00301 VGA_SOURCE = Source; 00302 VGA_SELECT = On_Off; 00303 } 00304 00305 void CDU_InitDisplay() 00306 { 00307 display.baudrate( 9600 ); //init uVGAIII card 00308 VGA_SIGNAL( VGA_INT, VGA_ON ); //select INTERNTAL video and set VGA switch ON 00309 } 00310 00311 void CDU_StartScreen() 00312 { 00313 string cTitle1="ENGRAVITY"; 00314 string cTitle2="CONTROL & DISPLAY UNIT"; 00315 display.cls(); 00316 00317 display.graphic_string( str2char( cTitle1 ), centertext( cTitle1, 24, LARGECHAR), LINE6, FONT_24X34, WHITE, 1, 1 ); 00318 wait_ms(1000); 00319 display.graphic_string( str2char( cTitle2 ), centertext( cTitle2, 24, LARGECHAR), LINE8, FONT_24X34, RED, 1, 1 ); 00320 wait_ms(1000); 00321 display.graphic_string( str2char( cTitle2 ), centertext( cTitle2, 24, LARGECHAR), LINE8, FONT_24X34, GREEN, 1, 1 ); 00322 wait_ms(1000); 00323 display.graphic_string( str2char( cTitle2) , centertext( cTitle2, 24, LARGECHAR), LINE8, FONT_24X34, BLUE, 1, 1 ); 00324 wait_ms(1000); 00325 display.graphic_string( str2char( cTitle2) , centertext( cTitle2, 24, LARGECHAR), LINE8, FONT_24X34, WHITE, 1, 1 ); 00326 wait_ms(1000); 00327 } 00328 00329 void CDU_ScreenAlign() 00330 //Draw a wireframe for aligning the screen on display with keys 00331 { 00332 display.cls(); 00333 00334 display.pen_size(WIREFRAME); 00335 display.rectangle(XMIN,YMIN,XMAX,YMAX, WHITE); 00336 display.line(XMIN,LINE2,XMAX,LINE2, WHITE); 00337 display.line(XMIN,LINE3,XMAX,LINE3, WHITE); 00338 display.line(XMIN,LINE4,XMAX,LINE4, WHITE); 00339 display.line(XMIN,LINE5,XMAX,LINE5, WHITE); 00340 display.line(XMIN,LINE6,XMAX,LINE6, WHITE); 00341 display.line(XMIN,LINE7,XMAX,LINE7, WHITE); 00342 display.line(XMIN,LINE8,XMAX,LINE8, WHITE); 00343 display.line(XMIN,LINE9,XMAX,LINE9, WHITE); 00344 display.line(XMIN,LINE10,XMAX,LINE10, WHITE); 00345 display.line(XMIN,LINE11,XMAX,LINE11, WHITE); 00346 display.line(XMIN,LINE12,XMAX,LINE12, WHITE); 00347 display.line(XMIN,LINE13,XMAX,LINE13, WHITE); 00348 display.line(XMIN,LINE14,XMAX,LINE14, WHITE); 00349 00350 } 00351 00352 void CDU_TestScreen() 00353 { 00354 display.display_control(IMAGE_FORMAT, NEW); //set correct image for reading from SD 00355 display.cls(); 00356 display.showpicture(0x00, 0x00, 0x00, 0x14, 0x00, 0x01, 0xB5); // Testscreen 00357 } 00358 00359 void CDU_LogoScreen() 00360 { 00361 display.display_control(IMAGE_FORMAT, NEW); //set correct image for reading from SD 00362 display.cls(); 00363 display.showpicture(0x00, 0x00, 0x00, 0x87, 0x00, 0x00, 0x00); // Engravity logo 00364 } 00365 00366 void CDU_Page() 00367 { 00368 display.cls(); 00369 display.graphic_string( "PERF INIT" , 8*24, LINE1, FONT_24X34, WHITE, 1, 1 ); 00370 00371 display.graphic_string( "GW/CRZ CG" , 0, LINE2, FONT_12X34, WHITE, 1, 1 ); 00372 display.graphic_string( "CRZ ALT" , 41*12, LINE2, FONT_12X34, WHITE, 1, 1 ); 00373 00374 display.graphic_string( "___._ / 26.2%" , 0, LINE3, FONT_24X34, WHITE, 1, 1 ); 00375 display.graphic_string( "_____" , 19*24, LINE3, FONT_24X34, WHITE, 1, 1 ); 00376 00377 display.graphic_string( "FUEL" , 0, LINE4, FONT_12X34, WHITE, 1, 1 ); 00378 display.graphic_string( "CRZ/WIND" , 40*12, LINE4, FONT_12X34, WHITE, 1, 1 ); 00379 00380 display.graphic_string( "0.0" , 0, LINE5, FONT_24X34, WHITE, 1, 1 ); 00381 display.graphic_string( "000$ /---" , 15*24, LINE5, FONT_24X34, WHITE, 1, 1 ); 00382 00383 display.graphic_string( "ZFW" , 0, LINE6, FONT_12X34, WHITE, 1, 1 ); 00384 display.graphic_string( "___._ " , 0, LINE7, FONT_24X34, WHITE, 1, 1 ); 00385 00386 display.graphic_string( "RESERVES" , 0, LINE8, FONT_12X34, WHITE, 1, 1 ); 00387 display.graphic_string( "__._ " , 0, LINE9, FONT_24X34, WHITE, 1, 1 ); 00388 00389 display.graphic_string( "COST INDEX" , 0, LINE10, FONT_12X34, WHITE, 1, 1 ); 00390 display.graphic_string( "TRANS ALT" , 39*12, LINE10, FONT_12X34, WHITE, 1, 1 ); 00391 00392 display.graphic_string( "___" , 0, LINE11, FONT_24X34, WHITE, 1, 1 ); 00393 display.graphic_string( "_____" , 19*24, LINE11, FONT_24X34, WHITE, 1, 1 ); 00394 00395 display.graphic_string( "------------------------------------------------" , 0, LINE12, FONT_12X34, WHITE, 1, 1 ); 00396 00397 display.graphic_string( "<INDEX" , 0, LINE13, FONT_24X34, WHITE, 1, 1 ); 00398 display.graphic_string( "N1 LIMIT>" , 15*24, LINE13, FONT_24X34, WHITE, 1, 1 ); 00399 00400 display.graphic_string( "SCRATCHPAD DATA LINE", centertext("SCRATCHPAD DATA LINE", 24, LARGECHAR) , LINE14, FONT_24X34, RED, 1, 1 ); 00401 00402 } 00403 00404 void CDU_displayclear(){ 00405 display.cls(); 00406 } 00407
Generated on Tue Jul 12 2022 11:39:27 by
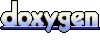