voor willem test
Dependencies: 4DGL MODSERIAL mbed mbos
CDU2FS_message_4.cpp
00001 // L. van der Kolk, ELVEDEKA, Holland 00002 // File: CDU2FS_message_4.cpp 00003 // 00004 // -- Message handling from CDU to FS -- 00005 00006 #include "mbed.h" 00007 #include "MODSERIAL.h" 00008 #include "keys.h" 00009 #include "debug_lvdk.h" // : debug mode control 00010 00011 extern MODSERIAL USB; 00012 extern MODSERIAL SERIAL_DEBUG; 00013 extern int CDU_FS_interface; 00014 00015 int key_hit_ID = 0; // : number of key that was hit, 0 = no hit of any key. ( global flag ) 00016 00017 char key_message[25] = "$PCDUKEY,"; // : setup begin of KEY message to FS 00018 char alive_message[25] = "$PCDUOKE,"; // : setup begin of ALIVE message to FS 00019 00020 00021 void send_message_to_FS(char *message_string) { 00022 // Common fnction to send a created message string (KEY or OKE) to the FS. 00023 // Parameter is pointer to char string that has to be sent. 00024 // Interface can be USB port or Ethernet port. 00025 int i = 0; 00026 if ( CDU_FS_interface == 0 ) { // : messages will be sent out by USB port 00027 while ( message_string[i] != '\0' ) { 00028 USB.putc(message_string[i]); 00029 i++; 00030 } 00031 } 00032 if ( CDU_FS_interface == 1 ) { 00033 // Messages will be sent out by Ehternet interface 00034 // - Not implemented - 00035 } 00036 } 00037 00038 00039 void Send_ALIVE_message(int seconds){ 00040 int i; 00041 char byte_read; 00042 char exor_byte = 0; 00043 //Create alive message: 00044 i = 9; // : i points to first place after "$PCDUOKE," 00045 // Add seconds in 2 dec digits and a '*' char : 00046 sprintf(&alive_message[i],"%02d*",seconds); 00047 // Calculate checksum now : 00048 i = 1; // : i points to first place after '$' 00049 do { byte_read = alive_message[i]; 00050 if (byte_read == '*') break; // : exclude '*' from exor calculation 00051 exor_byte = exor_byte ^ byte_read; 00052 i++; 00053 } while ( i < 20 ); 00054 i++; // : i now points to first digit of checksum after '*' 00055 // Add exor_byte in 2 hex chars (with upper case A-F) and a CR + LF: 00056 sprintf(&alive_message[i],"%02X\r\n",exor_byte); // : + extra NULL char added by sprintf 00057 send_message_to_FS(alive_message); // : send message to defined CDU-FS interface 00058 00059 } 00060 00061 void Send_KEY_message(int key_nr) { 00062 // Function creates a valid KEY message out of key_nr parameter. 00063 // Based on key_nr, a key char string is looked up and added to the message. 00064 // After adding a checksum, the total KEY message will be sent. 00065 int i; 00066 char byte_read; 00067 char exor_byte = 0; 00068 // Create key message, starting with "$PCDUKEY," message header 00069 i = 9; // : i points to first position after "$PCDUKEY," message header 00070 // Add key string to message string including '*' 00071 00072 if ( key_nr != 0 && key_nr < max_keys_CDUpanel ) { 00073 strcpy(&key_message[i],key_value[key_nr]); 00074 // Calculate checksum now : 00075 i = 1; // : i points to first place after '$' in message 00076 do { byte_read = key_message[i]; 00077 if (byte_read == '*') break; // : exclude '*' from calculation 00078 exor_byte = exor_byte ^ byte_read; 00079 i++; 00080 } while ( i < 20 ); 00081 i++; // : i now points to first digit of checksum after '*' 00082 // Add exor_byte in 2 hex digits and a CR + LF: 00083 sprintf(&key_message[i],"%02X\r\n",exor_byte); // : extra NULL char added by sprintf 00084 send_message_to_FS(key_message); // : send message to defined CDU-FS interface 00085 } 00086 } 00087 00088
Generated on Tue Jul 12 2022 11:39:27 by
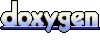