
C++ file for display control
Dependencies: 4DGL mbed ConfigFile
Fork of 4DGLtest by
fs_datastructures.cpp
00001 #include "mbed.h" 00002 #include "TFT_4DGL.h" 00003 #include "display.h" 00004 #include "fs_datastructures.h" 00005 00006 #include <string> 00007 using namespace std; 00008 00009 00010 // ---- FS-to-CDU data structures filled with received data --------------------------------------- 00011 00012 #define max_text0 48 // max text length font 0 00013 #define max_text1 24 // max text length font 1 00014 #define max_lines 14 // max nr of screen lines 00015 #define max_font 1 // possible fonts are 0 to max_font, so now 0 and 1 possible 00016 #define max_col_nr 255 // highest possible R,G, or B colour number 00017 #define fstyle_1 'S' // possible font style character 1 00018 #define fstyle_2 'N' // possible font style character 2 00019 #define max_keys 100 // max total nr of select keys 0 - 99 00020 #define max_leftkeys 6 // max nr of used LEFT select keys ( 0 - 49 ) 00021 #define max_rightkeys 6 // max nr of used RIGHT select keys ( 50 - 99 ) 00022 00023 extern int FSdata_received_flag; //Prototype of data flag (declared in main.cpp) 00024 extern TFT_4DGL display; //Prototype of Display Driver (declared in display.cpp) 00025 00026 //Prototype of indicators (declared in keyboard.cpp) 00027 extern DigitalOut EXEC; 00028 extern DigitalOut FAIL; 00029 extern DigitalOut DSPY; 00030 extern DigitalOut MSG; 00031 extern DigitalOut OFST; 00032 00033 extern void CDU_SET_BGL_INTENSITY( int nVal ); //Prototype of function controlling CDU backlight (declared in keyboard.cpp) 00034 extern int nFontSize( int nfont_number ); //Prototype of function for fontselection (declared in display.cpp) 00035 extern int nFontWidth (int nfont_number ); //Prototype of function to retrieve font width (declared in display.cpp) 00036 extern int nLine2Pixel( int nLine ); //Prototype of function to calculate vertical pixelposition from line number (declared in display.cpp) 00037 extern int LeftOrRight( int nTextLine, string cString, int nChars, int nCharWidth ); //declared in display.cpp 00038 extern unsigned int cRGB( char cRED, char cGREEN, char cBLUE ); //Prototype of function for assembly color word (declared in display.cpp) 00039 00040 // FS_data_update_ID: 00041 // These global flags indicate what data has been updated. 00042 // Should be tested when FS_DATA_EVENT occurs. 00043 extern int Background_Col_Update; // 1 when color was updated, must be reset to 0 when data has been read 00044 extern int CDU_Status_Update ; // 1 when status was updated, must be reset to 0 when data has been read 00045 extern int DO_CLR_SCREEN ; // 1 when screen should be cleared, must be reset to 0 when done 00046 extern int Text_Line_Update ; // equal to line number whose text was updated, must be reset to 0 when text has been read 00047 extern int Key_Maintext_Update ; // equal to keynumber whose main text line was updated, must be reset to -1 (!)when text has been read 00048 extern int Key_Subtext_Update ; // equal to keynumber whose sub text line was updated, must be reset to -1 (!) when text has been read 00049 00050 // Common flag to signal that one or more updates were performed: 00051 int FSdata_received_flag = false; // : true when one or more FS-to-CDU data structures were updated 00052 00053 // -------------------------------------------------------------------------------------------------- 00054 00055 void CDU_DSP_CSS() 00056 /*Check flags to see if action is required 00057 Background_Col_Update; // : 1 when color was updated, must be reset to 0 when data has been read 00058 CDU_Status_Update ; // : 1 when status was updated, must be reset to 0 when data has been read 00059 DO_CLR_SCREEN ; // : 1 when screen should be cleared, must be reset to 0 when done 00060 Text_Line_Update ; // : equal to line number whose text was updated, must be reset to 0 when text has been read 00061 Key_Maintext_Update ; // : equal to keynumber whose main text line was updated, must be reset to -1 (!)when text has been read 00062 Key_Subtext_Update ; // : equal to keynumber whose sub text line was updated, must be reset to -1 (!) when text has been read 00063 */ 00064 00065 { 00066 int nLine = 1; //default value 00067 //check common flag 00068 if ( FSdata_received_flag ) 00069 //if set, check all structures and lock dynamic storage 00070 { 00071 if ( Background_Col_Update == 1) 00072 { 00073 display.background_color( cRGB( BACKGROUND_COL.BG_RED, BACKGROUND_COL.BG_GREEN, BACKGROUND_COL.BG_BLUE ) ); 00074 Background_Col_Update = 0; 00075 } 00076 00077 if ( Key_Maintext_Update > -1 ) 00078 //Key_Maintext_Update contains the line number 00-49 is LSK text, 50-99 is RSK text 00079 //Currently used: 00080 //00-14 left side of screen --> left adjust, horizontal position = 0 00081 //50-64 right side of screen --> right adjust, horizontal position calculated with righttext() (declared in display.cpp) 00082 00083 //00 = LSK1 50 = RSK1 Print on LINE 3 00084 //01 = LSK2 51 = RSK2 Print on LINE 5 00085 //02 = LSK3 52 = RSK3 Print on LINE 7 00086 //03 = LSK4 53 = RSK4 Print on LINE 9 00087 //04 = LSK5 54 = RSK5 Print on LINE 11 00088 //05 = LSK6 55 = RSK6 Print on LINE 13 00089 { 00090 switch ( Key_Maintext_Update ) 00091 { 00092 case ( 00 ): nLine = 3; break; 00093 case ( 50 ): nLine = 3; break; 00094 case ( 01 ): nLine = 5; break; 00095 case ( 51 ): nLine = 5; break; 00096 case ( 02 ): nLine = 7; break; 00097 case ( 52 ): nLine = 7; break; 00098 case ( 03 ): nLine = 9; break; 00099 case ( 53 ): nLine = 9; break; 00100 case ( 04 ): nLine = 11; break; 00101 case ( 54 ): nLine = 11; break; 00102 case ( 05 ): nLine = 13; break; 00103 case ( 55 ): nLine = 13; break; 00104 } 00105 //display.graphic_string(char *s, int x, int y, char font, int color, char width multiplier, char height multiplier) 00106 display.graphic_string( SELKEY_MAINTEXT[Key_Maintext_Update].text , //Text to display 00107 LeftOrRight( Key_Maintext_Update, SELKEY_MAINTEXT[Key_Maintext_Update].text,24,24 )*nFontWidth( SELKEY_MAINTEXT[Key_Maintext_Update].font_size ) , //Horizontal position 00108 nLine2Pixel( nLine ), //Vertical position 00109 nFontSize( SELKEY_MAINTEXT[Key_Maintext_Update].font_size ), //Font 00110 cRGB( SELKEY_MAINTEXT[Key_Maintext_Update].text_RED ,SELKEY_MAINTEXT[Key_Maintext_Update].text_GREEN ,SELKEY_MAINTEXT[Key_Maintext_Update].text_BLUE ), 00111 1, 1 ); 00112 Key_Maintext_Update = -1; 00113 } 00114 00115 if ( Key_Subtext_Update > -1 ) 00116 //Key Subtext_Update contains the line number 00-49 is LSK subtext, 50-99 is RSK subtext 00117 //Currently used: 00118 //00-14 left side of screen --> left adjust, horizontal position = 0 00119 //50-64 right side of screen --> right adjust, horizontal position calculated with righttext() (declared in display.cpp) 00120 //00 = LSK1 50 = RSK1 Print on LINE 2 00121 //01 = LSK2 51 = RSK2 Print on LINE 4 00122 //02 = LSK3 52 = RSK3 Print on LINE 6 00123 //03 = LSK4 53 = RSK4 Print on LINE 8 00124 //04 = LSK5 54 = RSK5 Print on LINE 10 00125 //05 = LSK6 55 = RSK6 Print on LINE 12 00126 { 00127 switch ( Key_Maintext_Update ) 00128 { 00129 case ( 00 ): nLine = 2; break; 00130 case ( 50 ): nLine = 2; break; 00131 case ( 01 ): nLine = 4; break; 00132 case ( 51 ): nLine = 4; break; 00133 case ( 02 ): nLine = 6; break; 00134 case ( 52 ): nLine = 6; break; 00135 case ( 03 ): nLine = 8; break; 00136 case ( 53 ): nLine = 8; break; 00137 case ( 04 ): nLine = 10; break; 00138 case ( 54 ): nLine = 10; break; 00139 case ( 05 ): nLine = 12; break; 00140 case ( 55 ): nLine = 12; break; 00141 } 00142 //display.graphic_string(char *s, int x, int y, char font, int color, char width multiplier, char height multiplier) 00143 display.graphic_string( SELKEY_SUBTEXT[Key_Subtext_Update].text , //Text to display 00144 LeftOrRight( Key_Subtext_Update, SELKEY_SUBTEXT[Key_Subtext_Update].text,12,48 )*nFontWidth( SELKEY_SUBTEXT[Key_Subtext_Update].font_size ) , //Horizontal position 00145 nLine2Pixel( nLine ), //Vertical position 00146 nFontSize( SELKEY_SUBTEXT[Key_Subtext_Update].font_size ), //Font 00147 cRGB( SELKEY_SUBTEXT[Key_Subtext_Update].text_RED ,SELKEY_SUBTEXT[Key_Subtext_Update].text_GREEN ,SELKEY_SUBTEXT[Key_Subtext_Update].text_BLUE ), 00148 1, 1 ); //multiplier always on 1 00149 Key_Subtext_Update = -1; 00150 } 00151 00152 if ( Text_Line_Update > 0) 00153 { 00154 //Text_Line_Update contains the the line number to write 00155 display.graphic_string( TEXTLINE[Text_Line_Update].text , //Text to display 00156 0, //Horizontal position always 0 00157 nLine2Pixel( Text_Line_Update ), //Vertical position 00158 nFontSize( TEXTLINE[Text_Line_Update].font_size ), //Font 00159 cRGB( TEXTLINE[Text_Line_Update].text_RED ,TEXTLINE[Text_Line_Update].text_GREEN ,TEXTLINE[Text_Line_Update].text_BLUE ), 00160 1, 1 ); //multiplier always on 1 00161 Text_Line_Update = 0; 00162 } 00163 00164 if ( CDU_Status_Update == 1 ) 00165 { 00166 /* 00167 CDU_STATUS.stby_mode; // : 0 = operational mode, 1 = standby mode 00168 */ if ( CDU_STATUS.backlight ) // Backlight control 00169 { 00170 CDU_SET_BGL_INTENSITY( 255 ); 00171 } 00172 else 00173 { 00174 CDU_SET_BGL_INTENSITY( 0 ); 00175 } 00176 // Set CDU indicators 00177 MSG = CDU_STATUS.msg_indicator; 00178 EXEC = CDU_STATUS.exec_indicator; 00179 FAIL = CDU_STATUS.fail_indicator; 00180 DSPY = CDU_STATUS.dspy_indicator; 00181 OFST = CDU_STATUS.ofst_indicator; 00182 00183 CDU_Status_Update =0; 00184 } 00185 if ( DO_CLR_SCREEN ) 00186 { 00187 display.cls(); 00188 DO_CLR_SCREEN =0; 00189 } 00190 } 00191 //Reset global flag and release lock on dynamic storage 00192 FSdata_received_flag = false ; 00193 } 00194 00195 /* 00196 void GET_NEW_DATA_TASK(void) // : read new screendata from FS datastructures 00197 { 00198 while(1) { // : loop forever because it is a mbos taskfunction 00199 CDU_OS.WaitEvent(FS_DATA_EVENT); 00200 CDU_OS.LockResource(FS_DATA_RESOURCE); //lock resource to prevent intermediate updates 00201 CDU_CSS() 00202 CDU_OS.FreeResource(FS_DATA_RESOURCE); //free resource 00203 } 00204 } 00205 */
Generated on Thu Jul 14 2022 06:25:42 by
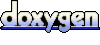