Example program using the emWin GUI library.
Dependencies: DMSupport DMemWin
main.cpp
00001 #include "mbed.h" 00002 #include "DMBoard.h" 00003 #include "EwHAL.h" 00004 #include "WM.h" 00005 00006 void MainTask(void); 00007 00008 int main() 00009 { 00010 DMBoard::BoardError err; 00011 DMBoard* board = &DMBoard::instance(); 00012 RtosLog* log = board->logger(); 00013 Display* disp = board->display(); 00014 00015 do { 00016 err = board->init(); 00017 if (err != DMBoard::Ok) { 00018 log->printf("Failed to initialize the board, got error %d\r\n", err); 00019 break; 00020 } 00021 00022 log->printf("\n\nHello World!\n\n"); 00023 00024 // Create the HAL for emWin 00025 // - Use 3 frame buffers for tripple-buffering 00026 // - Allow emWin to use 12MByte of external SDRAM 00027 EwHAL hal(3, 12*1024*1024); 00028 00029 // Start display in default mode (16-bit) 00030 Display::DisplayError disperr = disp->powerUp(hal.getFrameBufferAddress()); 00031 if (disperr != Display::DisplayError_Ok) { 00032 log->printf("Failed to initialize the display, got error %d\r\n", disperr); 00033 break; 00034 } 00035 00036 // Add extra options here 00037 // - Set WM_CF_MEMDEV option to use "Memory Devices" to reduce flickering 00038 WM_SetCreateFlags(WM_CF_MEMDEV); 00039 00040 // Execute the emWin example and never return... 00041 MainTask(); 00042 } while(false); 00043 00044 mbed_die(); 00045 }
Generated on Fri Jul 15 2022 14:39:12 by
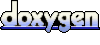