Example using the application launcher.
Dependencies: DMBasicGUI DMSupport
AppTemplate.cpp
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #include "mbed.h" 00019 #include "AppTemplate.h" 00020 #include "lpc_swim_font.h" 00021 #include "lpc_colors.h" 00022 #include "image_data.h" 00023 00024 /****************************************************************************** 00025 * Defines and typedefs 00026 *****************************************************************************/ 00027 00028 #define BTN_WIDTH 65 00029 #define BTN_HEIGHT 40 00030 #define BTN_OFF 20 00031 00032 /****************************************************************************** 00033 * Local variables 00034 *****************************************************************************/ 00035 00036 00037 /****************************************************************************** 00038 * Private functions 00039 *****************************************************************************/ 00040 00041 static void buttonClicked(uint32_t x) 00042 { 00043 bool* done = (bool*)x; 00044 *done = true; 00045 } 00046 00047 void AppTemplate::draw() 00048 { 00049 // Prepare fullscreen 00050 swim_window_open(_win, 00051 _disp->width(), _disp->height(), // full size 00052 (COLOR_T*)_fb, 00053 0,0,_disp->width()-1, _disp->height()-1, // window position and size 00054 0, // border 00055 BLUE, WHITE, BLACK); // colors: pen, backgr, forgr 00056 00057 // Show a message 00058 swim_put_text_xy(_win, "Hello World!", 100, 100); 00059 00060 // Create the OK button that will end this application 00061 _btn = new ImageButton(_win->fb, _win->xpmax - BTN_OFF - BTN_WIDTH, _win->ypmax - BTN_OFF - BTN_HEIGHT, BTN_WIDTH, BTN_HEIGHT); 00062 _btn->loadImages(img_ok, img_size_ok); 00063 _btn->draw(); 00064 } 00065 00066 /****************************************************************************** 00067 * Public functions 00068 *****************************************************************************/ 00069 00070 AppTemplate::AppTemplate() : _disp(NULL), _win(NULL), _fb(NULL), _btn(NULL) 00071 { 00072 } 00073 00074 AppTemplate::~AppTemplate() 00075 { 00076 teardown(); 00077 } 00078 00079 bool AppTemplate::setup() 00080 { 00081 _disp = DMBoard::instance().display(); 00082 _win = (SWIM_WINDOW_T*)malloc(sizeof(SWIM_WINDOW_T)); 00083 _fb = _disp->allocateFramebuffer(); 00084 00085 return (_win != NULL && _fb != NULL); 00086 } 00087 00088 void AppTemplate::runToCompletion() 00089 { 00090 // Draw on the frame buffer 00091 draw(); 00092 00093 // Configure the button to call buttonClicked() when clicked 00094 bool done = false; 00095 _btn->setAction(buttonClicked, (uint32_t)&done); 00096 00097 // Switch to "our" frame buffer, but save the old one so it can be 00098 // restored when we exit our application 00099 void* oldFB = _disp->swapFramebuffer(_fb); 00100 00101 TouchPanel* touch = DMBoard::instance().touchPanel(); 00102 touch_coordinate_t coord; 00103 while(!done) { 00104 // wait for a new touch signal (signal is sent from AppLauncher, 00105 // which listens for touch events) 00106 ThisThread::flags_wait_all(0x1); 00107 if (touch->read(coord) == TouchPanel::TouchError_Ok) { 00108 00109 // you can do something where with the touch event if you like 00110 // for example 00111 // 00112 // swim_put_circle(_win, coord.x, coord.y, 2, 1); 00113 // 00114 00115 00116 // See if the touch event effects the button 00117 if (_btn->handle(coord.x, coord.y, coord.z > 0)) { 00118 _btn->draw(); 00119 } 00120 } 00121 } 00122 00123 // User has clicked the button, restore the original frame buffer 00124 _disp->swapFramebuffer(oldFB); 00125 swim_window_close(_win); 00126 } 00127 00128 bool AppTemplate::teardown() 00129 { 00130 if (_win != NULL) { 00131 free(_win); 00132 _win = NULL; 00133 } 00134 if (_fb != NULL) { 00135 free(_fb); 00136 _fb = NULL; 00137 } 00138 if (_btn != NULL) { 00139 delete _btn; 00140 _btn = NULL; 00141 } 00142 return true; 00143 } 00144
Generated on Thu Jul 14 2022 03:32:21 by
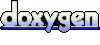