Adaptation of the official mbed USBHost repository to work with the LPC4088 Display Module
Dependents: DMSupport DMSupport DMSupport DMSupport
Fork of DM_USBHost by
USBHALHost.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBHALHOST_H 00018 #define USBHALHOST_H 00019 00020 #include "USBHostTypes.h" 00021 #include "USBHostConf.h" 00022 #include "rtos.h" 00023 00024 class USBHostHub; 00025 00026 #if defined(TARGET_LPC4088) || defined(TARGET_LPC4088_DM) 00027 #define HcRevision Revision 00028 #define HcControl Control 00029 #define HcCommandStatus CommandStatus 00030 #define HcInterruptStatus InterruptStatus 00031 #define HcInterruptEnable InterruptEnable 00032 #define HcHCCA HCCA 00033 #define HcControlHeadED ControlHeadED 00034 #define HcBulkHeadED BulkHeadED 00035 #define HcFmInterval FmInterval 00036 #define HcFmNumber FmNumber 00037 #define HcPeriodicStart PeriodicStart 00038 #define HcRhStatus RhStatus 00039 #define HcRhPortStatus1 RhPortStatus1 00040 #define HcRhPortStatus2 RhPortStatus2 00041 #define OTGStCtrl StCtrl 00042 #endif 00043 00044 /** 00045 * USBHALHost class 00046 */ 00047 class USBHALHost { 00048 public: 00049 uint8_t* getSafeMem(uint32_t size); 00050 void returnSafeMem(uint8_t* mem); 00051 00052 protected: 00053 00054 /** 00055 * Constructor 00056 * init variables and memory where will be stored HCCA, ED and TD 00057 */ 00058 USBHALHost(); 00059 00060 /** 00061 * Initialize host controller. Enable USB interrupts. This part is not in the constructor because, 00062 * this function calls a virtual method if a device is already connected 00063 */ 00064 void init(); 00065 00066 /** 00067 * reset the root hub 00068 */ 00069 void resetRootHub(); 00070 00071 /** 00072 * return the value contained in the control HEAD ED register 00073 * 00074 * @returns address of the control Head ED 00075 */ 00076 uint32_t controlHeadED(); 00077 00078 /** 00079 * return the value contained in the bulk HEAD ED register 00080 * 00081 * @returns address of the bulk head ED 00082 */ 00083 uint32_t bulkHeadED(); 00084 00085 /** 00086 * return the value of the head interrupt ED contained in the HCCA 00087 * 00088 * @returns address of the head interrupt ED contained in the HCCA 00089 */ 00090 uint32_t interruptHeadED(); 00091 00092 /** 00093 * Update the head ED for control transfers 00094 */ 00095 void updateControlHeadED(uint32_t addr); 00096 00097 /** 00098 * Update the head ED for bulk transfers 00099 */ 00100 void updateBulkHeadED(uint32_t addr); 00101 00102 /** 00103 * Update the head ED for interrupt transfers 00104 */ 00105 void updateInterruptHeadED(uint32_t addr); 00106 00107 /** 00108 * Enable List for the specified endpoint type 00109 * 00110 * @param type enable the list of ENDPOINT_TYPE type 00111 */ 00112 void enableList(ENDPOINT_TYPE type); 00113 00114 /** 00115 * Disable List for the specified endpoint type 00116 * 00117 * @param type disable the list of ENDPOINT_TYPE type 00118 */ 00119 bool disableList(ENDPOINT_TYPE type); 00120 00121 /** 00122 * Virtual method called when a device has been connected 00123 * 00124 * @param hub hub number of the device 00125 * @param port port number of the device 00126 * @param lowSpeed 1 if low speed, 0 otherwise 00127 * @param hub_parent reference to the hub where the device is connected (NULL if the hub parent is the root hub) 00128 */ 00129 virtual void deviceConnected(int hub, int port, bool lowSpeed, USBHostHub * hub_parent = NULL) = 0; 00130 00131 /** 00132 * Virtual method called when a device has been disconnected 00133 * 00134 * @param hub hub number of the device 00135 * @param port port number of the device 00136 * @param hub_parent reference to the hub where the device is connected (NULL if the hub parent is the root hub) 00137 * @param addr list of the TDs which have been completed to dequeue freed TDs 00138 */ 00139 virtual void deviceDisconnected(int hub, int port, USBHostHub * hub_parent, volatile uint32_t addr) = 0; 00140 00141 /** 00142 * Virtual method called when a transfer has been completed 00143 * 00144 * @param addr list of the TDs which have been completed 00145 */ 00146 virtual void transferCompleted(volatile uint32_t addr) = 0; 00147 00148 /** 00149 * Find a memory section for a new ED 00150 * 00151 * @returns the address of the new ED 00152 */ 00153 volatile uint8_t * getED(); 00154 00155 /** 00156 * Find a memory section for a new TD 00157 * 00158 * @returns the address of the new TD 00159 */ 00160 volatile uint8_t * getTD(); 00161 00162 /** 00163 * Release a previous memory section reserved for an ED 00164 * 00165 * @param ed address of the ED 00166 */ 00167 void freeED(volatile uint8_t * ed); 00168 00169 /** 00170 * Release a previous memory section reserved for an TD 00171 * 00172 * @param td address of the TD 00173 */ 00174 void freeTD(volatile uint8_t * td); 00175 00176 private: 00177 static void _usbisr(void); 00178 void UsbIrqhandler(); 00179 00180 void memInit(); 00181 00182 HCCA volatile * usb_hcca; //256 bytes aligned 00183 uint8_t volatile * usb_edBuf; //4 bytes aligned 00184 uint8_t volatile * usb_tdBuf; //4 bytes aligned 00185 00186 static USBHALHost * instHost; 00187 00188 bool volatile edBufAlloc[MAX_ENDPOINT]; 00189 bool volatile tdBufAlloc[MAX_TD]; 00190 00191 Mutex safemem_mutex; 00192 }; 00193 00194 #endif
Generated on Tue Jul 12 2022 21:45:29 by
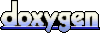