A derived version of the BSD licensed Adafrut GFX library for the SSD1351 controller for an OLED 128x128 display using SPI.
Fork of Adafruit_GFX by
Adafruit_SSD1351.h
00001 /*************************************************** 00002 This is a library for the 1.5" & 1.27" 16-bit Color OLEDs 00003 with SSD1331 driver chip 00004 00005 Pick one up today in the adafruit shop! 00006 ------> http://www.adafruit.com/products/1431 00007 ------> http://www.adafruit.com/products/1673 00008 00009 These displays use SPI to communicate, 4 or 5 pins are required to 00010 interface 00011 Adafruit invests time and resources providing this open source code, 00012 please support Adafruit and open-source hardware by purchasing 00013 products from Adafruit! 00014 00015 Written by Limor Fried/Ladyada for Adafruit Industries. 00016 BSD license, all text above must be included in any redistribution 00017 ****************************************************/ 00018 #pragma once 00019 00020 #include <mbed.h> 00021 00022 #include "Adafruit_GFX.h" 00023 00024 #define swap(a, b) { uint16_t t = a; a = b; b = t; } 00025 00026 #define SSD1351WIDTH 128 00027 #define SSD1351HEIGHT 128 // SET THIS TO 96 FOR 1.27"! 00028 00029 // Select one of these defines to set the pixel color order 00030 #define SSD1351_COLORORDER_RGB 00031 // #define SSD1351_COLORORDER_BGR 00032 00033 #if defined SSD1351_COLORORDER_RGB && defined SSD1351_COLORORDER_BGR 00034 #error "RGB and BGR can not both be defined for SSD1351_COLORODER." 00035 #endif 00036 00037 // Timing Delays 00038 #define SSD1351_DELAYS_HWFILL (3) 00039 #define SSD1351_DELAYS_HWLINE (1) 00040 00041 // SSD1351 Commands 00042 #define SSD1351_CMD_SETCOLUMN 0x15 00043 #define SSD1351_CMD_SETROW 0x75 00044 #define SSD1351_CMD_WRITERAM 0x5C 00045 #define SSD1351_CMD_READRAM 0x5D 00046 #define SSD1351_CMD_SETREMAP 0xA0 00047 #define SSD1351_CMD_STARTLINE 0xA1 00048 #define SSD1351_CMD_DISPLAYOFFSET 0xA2 00049 #define SSD1351_CMD_DISPLAYALLOFF 0xA4 00050 #define SSD1351_CMD_DISPLAYALLON 0xA5 00051 #define SSD1351_CMD_NORMALDISPLAY 0xA6 00052 #define SSD1351_CMD_INVERTDISPLAY 0xA7 00053 #define SSD1351_CMD_FUNCTIONSELECT 0xAB 00054 #define SSD1351_CMD_DISPLAYOFF 0xAE 00055 #define SSD1351_CMD_DISPLAYON 0xAF 00056 #define SSD1351_CMD_PRECHARGE 0xB1 00057 #define SSD1351_CMD_DISPLAYENHANCE 0xB2 00058 #define SSD1351_CMD_CLOCKDIV 0xB3 00059 #define SSD1351_CMD_SETVSL 0xB4 00060 #define SSD1351_CMD_SETGPIO 0xB5 00061 #define SSD1351_CMD_PRECHARGE2 0xB6 00062 #define SSD1351_CMD_SETGRAY 0xB8 00063 #define SSD1351_CMD_USELUT 0xB9 00064 #define SSD1351_CMD_PRECHARGELEVEL 0xBB 00065 #define SSD1351_CMD_VCOMH 0xBE 00066 #define SSD1351_CMD_CONTRASTABC 0xC1 00067 #define SSD1351_CMD_CONTRASTMASTER 0xC7 00068 #define SSD1351_CMD_MUXRATIO 0xCA 00069 #define SSD1351_CMD_COMMANDLOCK 0xFD 00070 #define SSD1351_CMD_HORIZSCROLL 0x96 00071 #define SSD1351_CMD_STOPSCROLL 0x9E 00072 #define SSD1351_CMD_STARTSCROLL 0x9F 00073 00074 00075 class Adafruit_SSD1351 : public virtual Adafruit_GFX 00076 { 00077 public: 00078 Adafruit_SSD1351(PinName cs, PinName rs, PinName dc, PinName clk, PinName data); 00079 00080 void on(); 00081 void off(); 00082 00083 uint16_t Color565(uint8_t r, uint8_t g, uint8_t b); 00084 00085 // drawing primitives! 00086 virtual void drawPixel(int16_t x, int16_t y, uint16_t color); 00087 void fillRect(uint16_t x0, uint16_t y0, uint16_t w, uint16_t h, uint16_t color); 00088 void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color); 00089 void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color); 00090 void fillScreen(uint16_t fillcolor); 00091 00092 void invert(bool); 00093 // commands 00094 void begin(void); 00095 void goTo(int x, int y); 00096 00097 void reset(void); 00098 00099 /* low level */ 00100 00101 void writeData(uint8_t d); 00102 void writeCommand(uint8_t c); 00103 00104 00105 void writeData_unsafe(uint16_t d); 00106 00107 void setWriteDir(void); 00108 void write8(uint8_t d); 00109 00110 private: 00111 SPI _spi; 00112 DigitalOut _cs, _dc, _reset; 00113 00114 void rawFillRect(uint16_t x, uint16_t y, uint16_t w, uint16_t h, uint16_t fillcolor); 00115 void rawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color); 00116 void rawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color); 00117 };
Generated on Mon Jul 18 2022 01:24:27 by
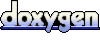