
Laila Al Badwawi 200906179 SpaceInvaders I declare this my own independent work and understand the university rules on plagiarism.
Dependencies: mbed
SpaceEngine.h
00001 #ifndef SPACEENGINE_H 00002 #define SPACEENGINE_H 00003 00004 #include "mbed.h" 00005 #include "N5110.h" 00006 #include "Gamepad.h" 00007 #include "Alien.h" 00008 #include "Spaceship.h" 00009 #include "Bullet.h" 00010 00011 00012 00013 /** bullet Class 00014 @brief SpaceEngine controlls the whole game 00015 @author Laila Al Badwawi, University of Leeds 00016 @date April 2019 00017 */ 00018 00019 class SpaceEngine 00020 { 00021 00022 public: 00023 00024 SpaceEngine(); 00025 ~SpaceEngine(); 00026 00027 /** 00028 *@brief initialise an identity for the spaceship,alien,bullet 00029 *@param x_spaceship @details the x-cooridante of spaceship in intger 00030 *@param y_spaceship @details the y-cooridante of spaceship in integer 00031 *@param speed_spaceship @details the speed of spaceship in integer 00032 *@param x_bullet @details the x-cooridante of bullet in intger 00033 *@param y_bullet @details the y-cooridante of bullet in integer 00034 *@param speed_bullet @details the speed of bullet in integer 00035 *@param fired_bullet@details the bullet fired by the spaceship 00036 *@param x_alien @details the x-cooridante of Alien in intger 00037 *@param y_alien @details the y-cooridante of Alien in integer 00038 *@param speed_alien @details the speed of Alien in integer 00039 */ 00040 void init(int x_spaceship,int y_spaceship, int x_bullet, int y_bullet,int fired_bullet, int x_alien,int y_alien, int speed_alien, int speed_bullet, int speed_spaceship); 00041 /** 00042 *@brief reading the positions of the objects 00043 *@param _(Gamepad and pad)@details the libraries which reading the the positions of the inputs(objects) in class SpaceEngine to detect the collisions. 00044 */ 00045 void read_input(Gamepad &pad); 00046 /** 00047 *@brief updating the positions of the objects 00048 *@param_(Gamepad and pad)@details the libraries which updating the the positions of the inputs(objects) in class SpaceEngine to detect the collisions. 00049 */ 00050 void update(Gamepad &pad); 00051 /** 00052 *@brief drawing the objects in the lcd 00053 *@param _(N5110 &lcd)@details the libraries which drawing the objects of class SpaceEngine on the screen. 00054 */ 00055 void draw(N5110 &lcd); 00056 00057 00058 private: 00059 /** 00060 *@brief checking the objects collision 00061 *@param _(Gamepad &pad)@details the libraries check the collision betwwen the objects of class SpaceEngine. 00062 */ 00063 void check_space_collision(Gamepad &pad); 00064 /** 00065 * _alien the object of class Alien 00066 */ 00067 Alien _alien; 00068 /** 00069 * _spaceship the object of class Spaceship 00070 */ 00071 Spaceship _spaceship; 00072 /** 00073 * _bullet the object of class Bullet 00074 */ 00075 Bullet _bullet; 00076 /* 00077 *@param (_x_spaceship) a private variable of class Spaceship that represents the x-cooridante of the spaceship 00078 */ 00079 int _x_spaceship; 00080 /* 00081 *@param (_y_spaceship) a private variable of class spaceship that represents the y-cooridante of the spaceship 00082 */ 00083 int _y_spaceship; 00084 /* 00085 *@param (_speed_spaceship) declation of a variable private member which shows the speed of the spaceship. 00086 */ 00087 int _speed_spaceship; 00088 /* 00089 *@param (_x_alien) a private variable of class Alien that represents the x-cooridante of the alien 00090 */ 00091 int _x_alien; 00092 /* 00093 *@param (_y_alien)a private variable of class Alien that represents the y-cooridante of the alien 00094 */ 00095 int _y_alien; 00096 /* 00097 *@param (_speed_alien)a private variable of class Alien that represents the speed of the alien 00098 00099 */ 00100 int _speed_alien; 00101 /* 00102 *@param (_alien_killed)a private variable of class SpaceEngine that represents the killed alien in integer 00103 */ 00104 int _alien_killed; 00105 /*@param 00106 (_fired_bullet)declation of a variable member which shows the fired bullet by the spaceship. 00107 */ 00108 int _fired_bullet; 00109 /*@param 00110 (_x_bullet)declation of a variable member which shows the x-cooridante of the bullet. 00111 */ 00112 int _x_bullet; 00113 /*@param 00114 (_y_bullet)declation of a variable member which shows the y-cooridante of the bullet. 00115 */ 00116 int _y_bullet; 00117 /* 00118 *@param (_speed_bullet)a private variable of class Bullet that represents the speed of the bullet. 00119 */ 00120 int _speed_bullet; 00121 00122 /* 00123 *@param (_spaceman)a private variable used for drawing spaceman in the weclcome screen in sprite. 00124 */ 00125 int _spaceman; 00126 /* 00127 *@param (x_alien)a private variable that represents the x-cooridante of the spaceman in integer 00128 */ 00129 int x_spaceman; 00130 /* 00131 *@param (y_alien)a private variable that represents the y-cooridante of the spaceman in integer 00132 */ 00133 int y_spaceman; 00134 /* 00135 *@param (_d)a private variable of class SpaceEngine that represents the direction of the joystic. 00136 */ 00137 Direction _d; 00138 /* 00139 *@param (_mag)a private variable of class SpaceEngine that represents the magitude of the joystic. 00140 */ 00141 float _mag; 00142 00143 00144 }; 00145 00146 #endif
Generated on Sat Aug 6 2022 12:54:46 by
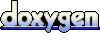