
Laila Al Badwawi 200906179 SpaceInvaders I declare this my own independent work and understand the university rules on plagiarism.
Dependencies: mbed
SpaceEngine.cpp
00001 #include"SpaceEngine.h" 00002 00003 SpaceEngine::SpaceEngine() //constructor of class SpaceEngine 00004 { 00005 } 00006 SpaceEngine::~SpaceEngine() //Destructor of class SpaceEngine 00007 { 00008 } 00009 00010 00011 // this function to initalise the objects of SpaceEngine class. 00012 void SpaceEngine::init(int x_spaceship,int y_spaceship, int x_bullet, int y_bullet,int fired_bullet, int x_alien,int y_alien, int speed_alien, int speed_bullet, int speed_spaceship) 00013 { 00014 _x_spaceship=x_spaceship; // represents the x-cooridante of the spaceship. 00015 _y_spaceship=y_spaceship; // represents the y-cooridante of the spaceship. 00016 _speed_spaceship=speed_spaceship; // speed of the spaceship. 00017 _x_bullet=x_bullet; // represents the x-cooridante of the spaceship. 00018 _y_bullet=y_bullet; // represents the y-cooridante of the spaceship. 00019 _fired_bullet= fired_bullet; // spaceship fired the alien by relaseing the bullet. 00020 _x_alien= x_alien; // represents the x-cooridante of the alien. 00021 _y_alien= y_alien; // represents the y-cooridante of the spaceship. 00022 _speed_alien= speed_alien; // speed of the alien. 00023 _speed_bullet=speed_bullet; // speed of the bullet. 00024 00025 _bullet.init(_x_bullet, _y_bullet, speed_bullet,fired_bullet); // initalise the object bullet. 00026 _alien.init (_x_alien, _y_alien,_speed_alien); // initalise the object alien. 00027 _spaceship.init( _x_spaceship, _y_spaceship, _speed_spaceship); // initalise the object spaceship. 00028 _alien_killed = 0; // set the killed alien at 0. 00029 } 00030 00031 // void function for Gamepad library to read the position of the objects. 00032 void SpaceEngine::read_input(Gamepad &pad) 00033 { 00034 _d = pad.get_direction(); 00035 _mag = pad.get_mag(); 00036 } 00037 00038 /**this function draw the objects on the screen 00039 *by using the (N5110 &lcd) libraries 00040 * if statment to check if the alien is alive so the lcd can draw it on the screen 00041 * else if statment will check if the alien dead so it should disappear 00042 *also it draws the spaceship 00043 */ 00044 void SpaceEngine::draw(N5110 &lcd) 00045 { 00046 _bullet.draw(lcd); 00047 if (_alien.isAlive() == true) { 00048 _alien.draw(lcd); 00049 // printf("alien is alive\n") 00050 } else { // alien is dead 00051 int y_pos = (rand() % 30) + 10; // random number 10 to 39 00052 _alien.init(70,y_pos,1); //initalise the alien with its parameters. 00053 wait(0.2);//wait 0.2 sec 00054 } 00055 _spaceship.draw(lcd); 00056 lcd.printString("alien_killed",0,0); 00057 char kills[14]; 00058 sprintf(kills,"%2d",_alien_killed); 00059 lcd.printString(kills,70,0); 00060 } 00061 00062 /* 00063 *this void function for reading the the positions of the inputs(objects)by(Gamepad &pad)libraries in class SpaceEngine to detect the collisions. 00064 *this inculde if statment that shows when joystic's direction to the east the bullt will be fired. 00065 */ 00066 void SpaceEngine::update(Gamepad &pad) 00067 { 00068 _bullet.update(_d,_mag); 00069 _alien.update(_d,_mag); 00070 _spaceship.update(_d,_mag); 00071 check_space_collision(pad); 00072 _y_alien = _alien.get_pos_y(); 00073 _x_alien = _alien.get_pos_x(); 00074 _y_bullet = _bullet.get_pos_y(); 00075 _x_bullet = _bullet.get_pos_x(); 00076 if(_d==E) { 00077 _fired_bullet=1; 00078 pad.tone(1000.0,0.1); 00079 // printf("bullet fired alien\n") 00080 _bullet.set_pos(_spaceship.get_pos_x()+33, _spaceship.get_pos_y()); 00081 } 00082 } 00083 00084 /*void function that checks the collision between the objects 00085 *if statment is to detect if x-coordinate of the bullet is larger or equal 65 00086 *and less than or equal to the 68 and if y-cooridante of the bullet is larger 00087 *than y-corrdindate of the alien-5 and y_alien+15 is larger or equal y_bullet 00088 *then the alien gets killed. 00089 */ 00090 void SpaceEngine::check_space_collision(Gamepad &pad) 00091 { 00092 if(_x_bullet >= 65 && _x_bullet <= 68 && _y_bullet >= _y_alien-5 && _y_bullet <= _y_alien+15) { 00093 _alien.setAlive(false); 00094 // printf("alive alien false\n") 00095 _alien_killed++; 00096 // printf(" killed alien incremebt by 1\n") 00097 } 00098 }
Generated on Sat Aug 6 2022 12:54:46 by
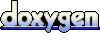