Project Submission (late)
Dependencies: mbed
VictoryMenu.h
00001 #ifndef VICTORYMENU_H 00002 #define VICTORYMENU_H 00003 00004 #include "Menus.h" 00005 00006 // yes button used in the VictoryMenu and the DefeatMenu 00007 /** YesButton Class 00008 * @brief Derived from Button. Used in VictoryMenu and DefeatMenu. 00009 * @brief Sends user to the StartMenu. 00010 */ 00011 class YesButton : public Button { 00012 public: 00013 YesButton() { 00014 x = 5; 00015 y = 32; 00016 } 00017 void virtual run() { 00018 printf("YesButton pushed\n"); 00019 restartFlag = true; 00020 } 00021 }; 00022 // no button used in the VictoryMenu and the DefeatMenu 00023 /** NoButton Class 00024 * @brief Derived from Button. Used in VictoryMenu and DefeatMenu. 00025 * @brief Sends user to the MainMenu. 00026 */ 00027 class NoButton : public Button { 00028 public: 00029 NoButton() { 00030 x = 58; 00031 y = 32; 00032 } 00033 void virtual run() { 00034 printf("NoButton pushed\n"); 00035 menuFlag = true; 00036 } 00037 }; 00038 // VictoryMenu displays when the player escapes the maze, it displays score 00039 // and restart options 00040 /** VictoryMenu Class 00041 * @brief Derived from Menu. Displayed when the maze is escaped. 00042 * @brief Or more accurately when the winFlag = true. 00043 */ 00044 class VictoryMenu : public Menu { 00045 public: 00046 VictoryMenu(N5110* screenPtr) : Menu(screenPtr) { 00047 buttons[0] = new YesButton; 00048 buttons[1] = new NoButton; 00049 currentButton = buttons[0]; 00050 numOfButtons = 2; 00051 buttonIndex = 0; 00052 } 00053 void virtual draw() { 00054 std::stringstream sscore; 00055 sscore << "Score: " << score; 00056 lcd->printString("VICTORY",10,1); 00057 lcd->printString(sscore.str().c_str(),10,2); 00058 lcd->printString("Play again?",10,3); 00059 lcd->printString("Yes No",10,4); 00060 } 00061 /** Destructor 00062 */ 00063 ~VictoryMenu() { 00064 delete buttons[0]; 00065 delete buttons[1]; 00066 } 00067 }; 00068 00069 #endif // VICTORYMENU_H
Generated on Thu Jul 14 2022 20:06:29 by
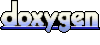