Project Submission (late)
Dependencies: mbed
Vector2Di.cpp
00001 #include "Vector2Di.h" 00002 00003 // Vector manipulation functions 00004 // they are pretty self explanatory (very basic) 00005 Vector2Di Vector2Di::operator -() { 00006 Vector2Di negV = {x*-1, y*-1}; 00007 return negV; 00008 } 00009 00010 void Vector2Di::addVector(Vector2Di v) { 00011 int newX = x + v.x; 00012 int newY = y + v.y; 00013 x = newX; 00014 y = newY; 00015 } 00016 00017 void Vector2Di::rotateVector(double angle) { 00018 double newX = (x * cos(angle)) + (y * -sin(angle)); 00019 double newY = (x * sin(angle)) + (y * cos(angle)); 00020 x = static_cast<int>(newX); 00021 y = static_cast<int>(newY); 00022 }
Generated on Thu Jul 14 2022 20:06:29 by
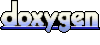