Project Submission (late)
Dependencies: mbed
StartMenu.h
00001 #ifndef STARTMENU_H 00002 #define STARTMENU_H 00003 00004 #include "Menus.h" 00005 00006 00007 // button which changes the size of the maze 00008 /** SizeButton Class 00009 * @brief Derived from Button. Allows the user to pick the size of Maze they wish to solve. 00010 * @brief Mazes can only be 12x12, 16x16 or 20x20. 00011 * @brief If enabled, the timer increases in length for larger mazes. 00012 */ 00013 class SizeButton : public Button { 00014 public: 00015 SizeButton() { 00016 x = 5; 00017 y = 16; 00018 } 00019 void virtual run() { 00020 if (mazeSize < 20) 00021 mazeSize += 4; 00022 printf("sizeButton pushed\n"); 00023 } 00024 void virtual runBack() { 00025 if (mazeSize > 12) 00026 mazeSize -= 4; 00027 printf("sizeButton decreasing\n"); 00028 } 00029 }; 00030 00031 // button which toggles the timer on or off 00032 /** TimerButton Class 00033 * @brief Derived from Button. Toggles the timer on or off. 00034 * @brief If turned off, the timer will count up instead of down and there is no faliure state. 00035 * @brief I.e. the program will only end when the maze is finished. 00036 */ 00037 class TimerButton : public Button { 00038 public: 00039 TimerButton() { 00040 x = 5; 00041 y = 24; 00042 } 00043 void virtual run() { 00044 timerFlag = !timerFlag; 00045 printf("timerButton pushed\n"); 00046 } 00047 }; 00048 00049 // button that triggers the main game loop 00050 /** PlayButton Class 00051 * @brief Derived from Buttons. Triggers the main game loop. 00052 */ 00053 class PlayButton : public Button { 00054 public: 00055 PlayButton() { 00056 x = 5; 00057 y = 40; 00058 } 00059 void virtual run() { 00060 printf("PlayButton pushed\n"); 00061 beginFlag = true; 00062 } 00063 }; 00064 00065 00066 // the StartMenu is the menu for game setup and starting the game. 00067 /** VictoryMenu Class 00068 * @brief Derived from Menu. Used to change the game's parameters of maze size and timer on or off. 00069 */ 00070 class StartMenu : public Menu { 00071 public: 00072 StartMenu(N5110* screenPtr) : Menu(screenPtr) { 00073 buttons[0] = new SizeButton; 00074 buttons[1] = new TimerButton; 00075 buttons[2] = new PlayButton; 00076 currentButton = buttons[0]; 00077 numOfButtons = 3; 00078 buttonIndex = 0; 00079 } 00080 void virtual draw() { 00081 std::stringstream ssize; 00082 ssize << "Size: " << mazeSize; 00083 std::string someString; 00084 if (timerFlag) 00085 someString = "Timer: YES"; 00086 else 00087 someString = "Timer: NO"; 00088 lcd->printString("Game params:",10,1); 00089 lcd->printString(ssize.str().c_str(),10,2); 00090 lcd->printString(someString.c_str(),10,3); 00091 lcd->printString("Play",10,5); 00092 } 00093 /** Destructor 00094 */ 00095 ~StartMenu() { 00096 delete buttons[0]; 00097 delete buttons[1]; 00098 } 00099 }; 00100 00101 #endif // STARTMENU_H
Generated on Thu Jul 14 2022 20:06:29 by
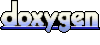