Project Submission (late)
Dependencies: mbed
Menus.h
00001 #ifndef MENUS_H 00002 #define MENUS_H 00003 00004 #include <string> 00005 #include <sstream> 00006 00007 #include "N5110.h" 00008 00009 /* because these classes are so short and so closely linked, they are declared 00010 and defined in the same files for convenience and clarity */ 00011 00012 /** Button Class 00013 * @brief An abstract class for all button's in the program 00014 * @brief The intent was to take advantage of polymorphism in C++ to cut out some large if-else blocks. 00015 * @brief There are two functions to overload, run() is always overloaded. 00016 * @brief runBack() is only overloaded for buttons attributed to increasing and decreasing variables, 00017 * @brief for example buttons to modify the maze size or contrast/brightness. 00018 * 00019 * @brief Version 1.0 00020 * @author Thomas Caine 00021 * @date May 2019 00022 */ 00023 class Button { 00024 public: 00025 int x; 00026 int y; 00027 /** Button default constructor 00028 */ 00029 Button() { 00030 x = y = 0; 00031 } 00032 /** virtual run function for polymorphism 00033 */ 00034 void virtual run() { 00035 printf("Button\n"); 00036 } 00037 /** virtual runBack function for polymorphism 00038 */ 00039 void virtual runBack() { 00040 printf("Back functionality\n"); 00041 // only overridden in functions that can be pressed backwards e.g. sliders 00042 // for contrast and brightness 00043 } 00044 }; 00045 00046 /** Menu Class 00047 * @brief An abstract class for all Menus's in the program 00048 * @brief Takes advantage of polymorphism. Draw() function is overriden in every inheriting Menu class. 00049 * @brief Score is used in the VictoryMenu and DefeatMenu classes. 00050 * @details Due to the similarity of Menu classes and their small size, I did not give them all header and source files. 00051 * @details Instead, classes are defined when they are declared in the same file and Buttons and Menus are grouped 00052 * @details into the same header files based on common functionality. 00053 * 00054 * @brief Version 1.0 00055 * @author Thomas Caine 00056 * @date May 2019 00057 */ 00058 class Menu { 00059 public: 00060 Button* buttons[3]; 00061 int score; // used in the victory and defeat menus 00062 int numOfButtons; 00063 int buttonIndex; 00064 N5110* lcd; 00065 /** Constructor for the Menu base class. 00066 * @param lcd pointer - Passed to every menu object (derived classes super this constructor) so they can print to the lcd 00067 */ 00068 Menu(N5110* screenPtr) : lcd(screenPtr) { 00069 numOfButtons = buttonIndex = 0; 00070 } 00071 /** Virtual draw function, overloaded by Menus to print unique stuff. 00072 */ 00073 void virtual draw() { 00074 printf("This is a menu\n"); 00075 } 00076 }; 00077 00078 // important flags used throughout the menus to interact with the game's settings 00079 Menu *currentMenu; 00080 Button *currentButton; 00081 double contrastVal = 0.5; 00082 double brightnessVal = 0.5; 00083 int mazeSize = 12; 00084 bool beginFlag = false; 00085 bool restartFlag = false; 00086 bool menuFlag = false; 00087 bool timerFlag = true; 00088 00089 00090 #endif // MENUS_H
Generated on Thu Jul 14 2022 20:06:28 by
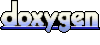